Introduction
Stay ahead and protect your business from cyberattacks with top-notch security measures and effective strategies.
Technology constantly changes, and cyberattacks are becoming more dangerous, necessitating vital security steps.
Developers must ensure app safety and security. Keeping up with security best practices for .NET apps is difficult due to the wide range of tools and technologies available.
Below is a list of essential .NET security tips to help other software developers stay ahead of the curve and keep their applications safe from malicious exploits.
By implementing security measures such as data encryption and preventing cross-site scripting attacks, you can develop .NET applications that are robust to sophisticated cyberattacks.
The following tips will give you the knowledge and skills to create secure and robust software.
Common .NET Security Vulnerabilities
As a .NET developer, you create secure and reliable applications that protect sensitive data and prevent unauthorized access.
With cyber threats becoming more advanced, staying up-to-date with the latest security best practices is crucial.
This post will cover six essential .NET security tips to help you create secure and resilient applications.
SQL Injection Protection
Now lets dive deeper into the workings of a .NET SQL injection and explore effective techniques to prevent such vulnerabilities.
Exploring the Issue: Consider a scenario where you have developed a blog engine using .NET. One common feature of blog engines is to include post titles in URLs without spaces or special characters, known as a “slug.” This slug is utilized to retrieve the corresponding post from the database.
Let’s examine a code snippet typically found in such applications:
var query = "SELECT Title, Body, Excerpt FROM Post WHERE Slug = '" + slug + "' ORDER BY Published DESC";
// Query execution, etc.
Identifying the Problem: The issue arises when the slug
variable, derived from user input, is directly concatenated into the query. This approach makes the application vulnerable to SQL injection attacks. An attacker could manipulate the URL to resemble the following:
<YOUR-DOMAIN-NAME>/posts/'; DROP TABLE Post;--
The concatenation of the slug
variable results in the following query:
SELECT Title, Body, Excerpt FROM Post WHERE Slug = ''; DROP TABLE Post;--' ORDER BY Published DESC
The single quote provided by the attacker matches the existing opening quote in the query. Subsequently, the malicious query executes a “DROP TABLE” statement. The inclusion of “–” denotes a comment, rendering the remaining part of the query ineffective.
Preventing .NET SQL Injection: Now that we comprehend the devastating consequences of SQL injection attacks, it is crucial to implement preventive measures. While input sanitization is often suggested, it alone is not sufficient, as explained by Kevin Smith in this informative article. Instead, we recommend the following strategies:
- Validating Input: Frequently, your application methods may only accept a restricted set of valid values. For example, if an action in your ASP.NET MVC app expects an integer parameter, there is no reason to allow a string input. Specifying the correct parameter type in the action method will cause any non-integer values to fail automatically.
- Using Allow lists: Building upon the previous approach, consider situations where the set of valid values is small enough to be enumerated. For instance, in a bug-tracking system, you might filter tickets based on severity levels (e.g., low, medium, high, critical). Since the range of possible values is limited, your application code can maintain a list of valid options in memory and validate URL parameters against this list. Any values not present in the list will be rejected outright.
- Implementing Parametrized Queries: The most effective technique to prevent .NET SQL injections (or injections in any tech stack) is by utilizing parametrized queries. Parametrized queries tackle SQL injection by completely excluding user-provided values from the query text. Instead, these values are supplied separately to the database.
Consider the following revised query from our previous example:
var query = "SELECT Title, Body, Excerpt FROM Post WHERE Slug = @slug ORDER BY Published DESC";
By using a placeholder (@slug
), we avoid string concatenation. But how do we pass the actual value?
After creating a new SqlCommand
, you can add a parameter using the AddWithValue
method:
var query = "SELECT Title, Body, Excerpt FROM Post WHERE Slug = @slug ORDER BY Published DESC";
var command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@slug", slug);
Implement Two-Factor Authentication to Secure User Accounts
User accounts are often the target of cyber attacks, and password-based authentication is no longer sufficient to ensure their security.
Providing users with two forms of identification is an essential part of two-factor authentication (2FA) as a security measure.
This can be a combination of a password and a fingerprint, a code sent to their phone or a security token. Software developers can significantly reduce unauthorized access to user accounts by implementing Two-Factor Authentication (2FA).
Encrypt Sensitive Data to Protect Against Data Breaches
Software developers need to understand the potential impact of data breaches. Encrypting sensitive data is one of the most effective ways to protect against data breaches.
Encryption converts plain text into ciphertext, making it unreadable to anyone needing the encryption key.
Encrypting sensitive data ensures that attackers cannot read or use the data even if attackers gain access to your database.
Here’s an example of how to encrypt a password in .NET using C#:using System;
using System.Security.Cryptography;
public class PasswordEncryption
{
// Method to encrypt a password using SHA256
public static string EncryptPassword(string password)
{
using (var sha256 = SHA256.Create())
{
// Convert the password string to a byte array
byte[] passwordBytes = System.Text.Encoding.UTF8.GetBytes(password);
// Compute the hash value of the password bytes
byte[] hashBytes = sha256.ComputeHash(passwordBytes);
// Convert the hashed bytes to a hexadecimal string
string hashedPassword = BitConverter.ToString(hashBytes).Replace("-", "");
return hashedPassword;
}
}
// Example usage
public static void Main(string[] args)
{
string password = "myPassword123";
string encryptedPassword = EncryptPassword(password);
Console.WriteLine("Encrypted Password: " + encryptedPassword);
}
}
In this example, we use the SHA256
algorithm from the System.Security.Cryptography
namespace to encrypt the password. The EncryptPassword
method takes the password as input, converts it to a byte array, computes the hash using SHA256, and then converts the hashed bytes to a hexadecimal string representation.
Use HTTPS to Secure Data in Transit
When data is transmitted over the internet, it is susceptible to interception and tampering. HTTPS is a protocol that encrypts data in transit, ensuring that it cannot be intercepted or manipulated.
By using HTTPS, you can ensure that all data transmitted between your application and the user’s browser is secure and private.
Validate User Input to Prevent Cross-Site Scripting Attacks
Cross-site scripting (XSS) attacks happen when a hacker adds malicious code to a web page.
This lets the hacker steal sensitive information or do things they shouldn’t. One of the best ways to stop XSS attacks is to check what the user types in.
How to validate user input in a .NET MVC application to prevent Cross-Site Scripting (XSS) attacks:
- Enable Request Validation: In your
Web.config
file, ensure that request validation is enabled. By default, ASP.NET MVC provides built-in request validation to protect against XSS attacks.
<configuration>
<system.web>
<httpRuntime targetFramework="yourTargetFramework" requestValidationMode="2.0" />
<pages validateRequest="true" />
</system.web>
</configuration>
- Use Model Validation Attributes: In your MVC model classes, apply validation attributes to the properties that accept user input. The following example uses the
RegularExpression
attribute to ensure that theName
property only allows alphabetic characters.
using System.ComponentModel.DataAnnotations;
public class User {
[Required]
[RegularExpression("^[a-zA-Z]*$", ErrorMessage = "Only alphabetic characters are allowed.")]
public string Name { get; set; } // Other properties...
}
- Validate Input in Controller Actions: In your controller actions, validate the user input before processing it. If the input is invalid, return appropriate error messages or redirect back to the form.
public class UserController : Controller {
[HttpPost]
public ActionResult Create(User user) {
if (ModelState.IsValid) {
// Process the user input
// ... return RedirectToAction("Success");
}
return View(user);
// Show the form with validation errors } // Other actions...
}
- Output Encoding: When rendering user input in your views, ensure that you use proper output encoding to prevent script execution. In Razor syntax, use the
@Html.Raw
or@Html.Encode
methods to properly encode user input:
<div> <p>User Name: @Html.Raw(Model.Name)</p> </div>
By applying these practices, you can validate user input to prevent XSS attacks in your .NET MVC application. Remember to perform input validation on both client-side and server-side to ensure robust security.
Keep Your .NET Framework and Dependencies Up to Date
The .NET Framework and its dependencies constantly evolve, and new security vulnerabilities are discovered regularly. Keeping your .NET
Framework and dependencies up-to-date are crucial to ensure your application is secure. Software developers should regularly update their software to keep it safe against the latest threats.
NuGet Package Manager Console:
One way to update dependencies is by using the NuGet Package Manager Console in Visual Studio. Open the console by going to “Tools” -> “NuGet Package Manager” -> “Package Manager Console”. Then, use the following commands:
- To check for outdated packages in the solution:
Get-Package -Updates
- To update a specific package:
Update-Package PackageName
- To update all packages in the solution:
Update-P
Package Manager Console with PackageReference format:
If your project is using the newer PackageReference format, you can leverage the Package Manager Console with the dotnet
CLI commands:
- To check for outdated packages:
dotnet list package --outdated
- To update a specific package:
dotnet add package PackageName
- To update all packages:
dotnet restore
Pro Tips about Dot Net Security Tips
1. Sign up for security newsletters and blogs to learn about the latest security threats and holes. You can ensure your applications are safe from the latest security threats by staying up-to-date.
2. Don’t rely solely on third-party security tools. While security tools can be helpful, they could be more foolproof. It’s essential to implement multiple layers of security, including secure coding practices and regular security audits.
3. Test your application’s security regularly. Software developers must conduct penetration testing and vulnerability scanning to detect potential vulnerabilities before they can be exploited. By regularly testing your application’s security, you can ensure that it remains secure and resilient.
Key .NET Security Takeaway
As a .NET developer, ensuring that your applications are secure and free from vulnerabilities is crucial.
Ensure your applications are secure and reliable by following these six .NET security tips.
To secure your website: use parameterized queries, 2FA, data encryption, HTTPS, input validation, and stay updated with .NET Framework.
Stay informed of the latest security threats, regularly test your application, and fix security vulnerabilities to secure your apps.
By implementing these best practices and proactively protecting your applications, you can ensure that your users’ data remains secure and your reputation remains intact.
It’s time to dive into our comprehensive guide to .NET security tips if you’re ready to improve your security game.
FAQs about Dot Net Security Tips
What are the most common types of cyberattacks that .NET developers should be aware of?
.NET developers should be aware of several types of cyberattacks, including SQL injection attacks, cross-site scripting (XSS) attacks, and data breaches.
Keep yourself informed about the latest security threats and vulnerabilities as a software developer.
What should I do if I discover a security vulnerability in my .NET application?
Taking immediate action is crucial if you find a security vulnerability in your .NET application. First, assess the severity of the vulnerability and its potential impact on your application and users.
Then, develop a plan to address the vulnerability and implement a fix as soon as possible. It’s also essential to communicate the issue with your users and provide them with updates as you work to resolve the issue.
How can I ensure my .NET application remains secure after deployment?
Ensuring your .NET application remains secure after deployment requires ongoing effort and vigilance.
Security audits, penetration tests, and vulnerability scanning can help identify weaknesses and vulnerabilities that may have been overlooked.
It’s also essential to stay up-to-date with the latest security best practices and software updates to protect your application against the latest security threats.
Ensure you communicate with your users about protecting their data and addressing security issues.
Final thoughts about Dot Net Security Tips
Ensure your applications are secure and reliable by following these six .NET security tips.
Remember to stay up-to-date with the latest security best practices and be proactive in protecting your applications from malicious exploits.
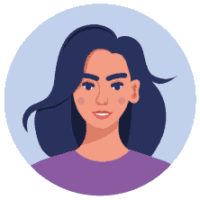
Jessica is a highly accomplished technical author specializing in scientific computer science. With an illustrious career as a developer and program manager at Accenture and Boston Consulting Group, she has made significant contributions to the successful execution of complex projects.
Jessica’s academic journey led her to CalTech University, where she pursued a degree in Computer Science. At CalTech, she acquired a solid foundation in computer systems, algorithms, and software engineering principles. This rigorous education has equipped her with the analytical prowess to tackle complex challenges and the creative mindset to drive innovation.
As a technical author, Jessica remains committed to staying at the forefront of technological advancements and contributing to the scientific computer science community. Her expertise in .NET C# development, coupled with her experience as a developer and program manager, positions her as a trusted resource for those seeking guidance and best practices. With each publication, Jessica strives to empower readers, spark innovation, and drive the progress of scientific computer science.