The Real Reasons .NET Core Boasts Platform Independence
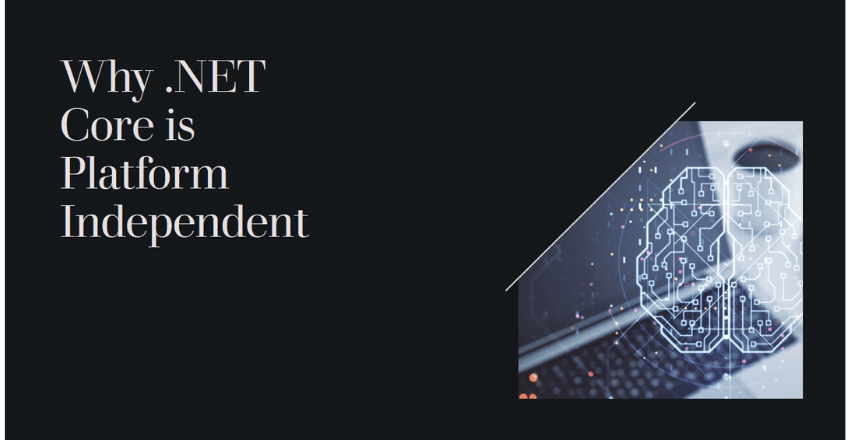
Introduction: The Topic Worth Your Attention
Claims float around in the fast-paced world of programming, triggering debates and sparking curiosity. The buzzword these days is that .NET Core is platform independent. As a .NET developer, I’ve dug into the core of .NET Core and now, I’m eager to reveal why .NET Core rightfully earns its label of being platform independent.
.NET Core: Understanding Its Roots
Before we leap into the heart of .NET Core’s platform independence, let’s first travel back in time to understand its inception and growth. Microsoft introduced .NET in 2002, designed primarily to run on Windows. Yet, as technological advancements demanded more versatile, cross-platform solutions, 2016 saw the birth of .NET Core—an open-source, platform-independent framework.
Key Takeaways:
- .NET Core’s Cross-Platform Capabilities: One of the primary benefits of .NET Core is its ability to run on multiple operating systems, including Windows, Linux, and macOS, without altering the codebase. This makes it an ideal choice for developers seeking flexibility and wide reach.
- Open-Source and Community Driven: Both .NET Core and ASP.NET Core are open source, fostering a community-driven approach that accelerates innovation, enhances code quality, and promotes transparency and trust among users.
- Self-Contained Deployments and Docker: .NET Core supports self-contained deployments and can be containerized using Docker, making it even more platform-independent. This makes it a great fit for cloud and container-based environments.
- Compatibility and .NET Standard: Always consider the compatibility of your libraries when targeting multiple platforms. Libraries that comply with .NET Standard are designed to be platform-independent, simplifying development across various environments.
- The Power of MAUI: With the introduction of MAUI (Multi-platform App UI) in .NET 6, developers can create native user interfaces that work across Windows, macOS, Android, and iOS. This makes .NET an increasingly attractive choice for cross-platform application development.
Platform Independence: What’s in a Name?
Platform independence signifies that a software product can flawlessly run across various operating systems without needing any modifications. This attribute is the holy grail of software development, offering unparalleled flexibility, extended reach, and significant cost savings.

Core Reasons: Making .NET Core Platform Independent
Let’s delve deeper into the heart of the matter: what exactly allows .NET Core to confidently declare itself platform independent?
1. Cross-platform Runtime
.NET Core employs the CoreCLR (Common Language Runtime), a powerful runtime environment that has the flexibility to adapt its behavior according to the operating platform. This ensures that your applications run smoothly across Windows, Linux, and macOS.
Consider this simple “Hello, World!” application, written in C#:
using System; namespace HelloWorld { class Program { static void Main(string[] args) { Console.WriteLine("Hello, World!"); } } }
Once you’ve compiled this application, you can run it on any platform where .NET Core runtime is installed. This speaks volumes about the cross-platform capabilities of .NET Core.
2. Versatile Deployment
.NET Core offers you two major deployment methods: framework-dependent and self-contained. This versatility in deployment options ensures that your applications can run effectively, no matter what the environment is.
Framework-dependent deployment (FDD), as the name implies, requires the .NET Core framework to be installed on the target machine. This reduces the size of your deployment package.
On the other hand, self-contained deployment (SCD) packages the .NET Core runtime along with your application, ensuring it can run on systems that do not have .NET Core installed.
Here’s a sample command to publish a self-contained application targeting Linux:
dotnet publish --runtime linux-x64 --configuration Release --self-contained true
3. Unified Base Class Library (BCL)
.NET Core comes with a core Base Class Library (BCL) that includes classes for collections, file systems, console, XML, async, and many more. This core BCL is designed to work consistently across different platforms. It serves as a foundational layer, ensuring your application behaves predictably no matter where it runs.
4. Docker Support
.NET Core’s support for Docker is another testament to its platform independence. You can containerize your .NET Core applications using Docker, which not only makes them platform independent but also enhances scalability, isolation, and deployment speed.
Here is a basic Dockerfile
for a .NET Core application:
FROM mcr.microsoft.com/dotnet/core/sdk:3.1 AS build-env
WORKDIR /app
# Copy csproj and restore as distinct layers
COPY *.csproj ./
RUN dotnet restore
# Copy everything else and build
COPY . ./
RUN dotnet publish -c Release -o out
# Build runtime image
FROM mcr.microsoft.com/dotnet/core/aspnet:3.1
WORKDIR /app
COPY --from=build-env /app/out .
ENTRYPOINT ["dotnet", "yourapp.dll"]
You can build the Docker image and run it on any platform where Docker is installed, once again reinforcing the platform independence of .NET Core.
These four critical components underpin the platform independence of .NET Core. Understanding these elements can be the key to exploiting the cross-platform capabilities of .NET Core to their fullest.
Addressing Doubts: Can We Call .NET Core Truly Platform Independent?
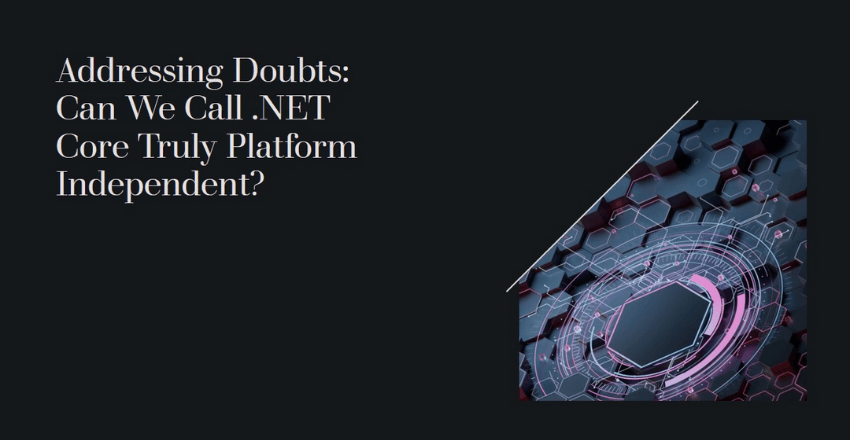
Let’s face it – even with the strong evidence in favor of .NET Core’s platform independence, skepticism exists. Some of the concerns raised by developers are valid and deserve attention. Let’s address some of these doubts.
The Microsoft Connection
The first doubt that often arises is the strong connection between .NET Core and Microsoft. Critics argue that .NET Core, being a Microsoft product, can never be truly platform independent.
They worry that Microsoft might subtly favor Windows or introduce proprietary features that work better on Windows than on Linux or macOS.
However, Microsoft has made a significant commitment to maintaining the open-source nature of .NET Core and ensuring its cross-platform functionality. They’ve established the .NET Foundation, an independent organization to foster open development and collaboration around the .NET ecosystem.
Moreover, a considerable portion of the code contributions to .NET Core now come from outside Microsoft, showing that the wider developer community plays a vital role in shaping .NET Core’s evolution.
OS Specific Limitations
Another concern often raised is about OS-specific limitations. Operating systems have different capabilities and restrictions, and this can sometimes lead to differences in application behavior.
While this is true, .NET Core does an exceptional job of abstracting these differences. It provides a consistent API across different platforms, and where there are OS-specific features, .NET Core provides a way to access them through the System.Runtime.InteropServices.RuntimeInformation
class.
Consider the following code snippet:
if (System.Runtime.InteropServices.RuntimeInformation.IsOSPlatform(OSPlatform.Windows)) { // Execute Windows specific code } else if (System.Runtime.InteropServices.RuntimeInformation.IsOSPlatform(OSPlatform.Linux)) { // Execute Linux specific code }
With this class, you can check the operating system at runtime and perform OS-specific operations when needed.
Lack of GUI Support in Linux and macOS
One valid concern about .NET Core’s platform independence is its lack of GUI support in Linux and macOS. While .NET Core runs smoothly on non-Windows platforms, its support for desktop applications with a graphical user interface is currently limited.
However, with the release of .NET 5 and the ongoing unification of .NET frameworks, Microsoft is working on this limitation. The introduction of MAUI (Multi-platform App UI), a cross-platform framework for building native UIs, is a step in this direction.
While these concerns have a basis, it’s clear that .NET Core is moving steadily towards true platform independence. Understanding these nuances can help you make informed decisions when considering .NET Core for cross-platform development.
Common Problems, Consequences, and Solutions in Using .NET Core for Platform Independence
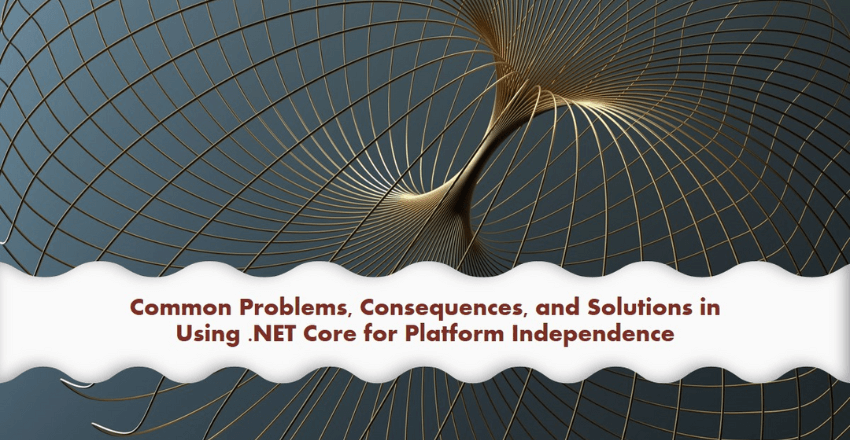
1. Problem: Limited Native UI Support
Developers often express frustration about .NET Core’s limited support for building native user interfaces on platforms other than Windows.
Consequence: This can be particularly problematic if you’re looking to create a cross-platform desktop application with a rich, responsive user interface. It might force developers to look for other frameworks or write platform-specific code, defeating the purpose of platform independence.
Solution: With the release of .NET 6, Microsoft introduced MAUI (Multi-platform App UI), a cross-platform framework for building native UIs.
With MAUI, you can create a single project that renders native UI on Windows, macOS, Android, and iOS. This is a promising development that should address this issue in future .NET versions.
// Use Microsoft.Maui.Controls; var newButton = new Button { Text = "Click me" }; newButton.Clicked += (s, e) => { newButton.Text = "Clicked!"; };
Above code creates a simple button in MAUI that changes text when clicked. It will work on all platforms supported by MAUI.
2. Problem: Different Behavior on Different Operating Systems
Though .NET Core aims to provide a consistent API across different platforms, developers sometimes observe slightly different behaviors on different operating systems due to underlying OS-specific characteristics.
Consequence: This can lead to unexpected results and bugs that are hard to track down. These issues might not appear during development or testing but could surface in production, causing significant problems.
Solution: Thorough testing is the key to managing this issue. Perform extensive testing on all target platforms to catch any OS-specific quirks. Also, .NET Core allows developers to check the current operating system at runtime and write OS-specific code where necessary.
if (System.Runtime.InteropServices.RuntimeInformation.IsOSPlatform(OSPlatform.Windows))
{
// Execute Windows specific code
}
3. Problem: Dependency on External Libraries
.NET Core applications may depend on libraries that are not cross-platform. This can become a significant roadblock in achieving platform independence.
Consequence: If your .NET Core application depends on a library that doesn’t support a specific platform, your application won’t run on that platform. This is a common issue that might force you to rewrite some parts of your application or look for alternative libraries.
Solution: Review the compatibility of all external libraries with your target platforms before starting the development process. .NET Core supports NuGet, which is a vast repository of developer-made libraries, many of which support multiple platforms.
The .NET Standard is another useful tool that helps to ensure code sharing and compatibility across different .NET implementations.
While .NET Core has made significant strides towards platform independence, it is not without challenges. Being aware of these potential hurdles and their solutions can help you navigate the cross-platform development process more effectively with .NET Core.
Future Outlook: The Impact of .NET Core’s Platform Independence
The platform independence of .NET Core ushers in a multitude of benefits:
- Extended Reach: Your applications can cater to a broader audience as they can function on almost any device.
- Economic Efficiency: You no longer need separate codebases for different platforms, which reduces both development and maintenance costs.
- Boosted Productivity: Developers can apply their .NET skills across an extensive range of platforms, decreasing the learning curve and enhancing productivity.
Frequently Asked Questions: “.NET Core is Platform Independent”
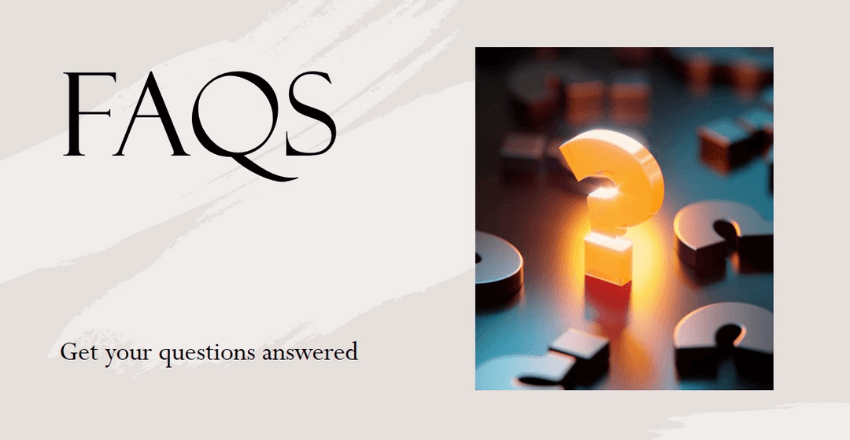
1. How can I create a self-contained deployment in .NET Core?
You can publish a self-contained application with the dotnet publish
command, specifying the target runtime and setting the --self-contained
flag to true.
Here’s an example of how you can create a self-contained deployment for a Linux environment:
dotnet publish --runtime linux-x64 --configuration Release --self-contained true
2. How can I use Docker with .NET Core for platform independence?
Docker allows you to containerize your .NET Core applications, making them platform independent.
Here is a sample Dockerfile for a .NET Core application:
FROM mcr.microsoft.com/dotnet/core/sdk:3.1 AS build-env
WORKDIR /app
# Copy csproj and restore as distinct layers
COPY *.csproj ./
RUN dotnet restore
# Copy everything else and build
COPY . ./
RUN dotnet publish -c Release -o out
# Build runtime image
FROM mcr.microsoft.com/dotnet/core/aspnet:3.1
WORKDIR /app
COPY --from=build-env /app/out .
ENTRYPOINT ["dotnet", "yourapp.dll"]
With this Dockerfile, you can build and run your .NET Core application on any system where Docker is installed.
3. How can I detect the operating system at runtime in .NET Core?
The System.Runtime.InteropServices.RuntimeInformation
class allows you to check the operating system at runtime.
Here’s a code snippet showing how you can use it:
if (System.Runtime.InteropServices.RuntimeInformation.IsOSPlatform(OSPlatform.Windows))
{
// Execute Windows specific code
}
else if (System.Runtime.InteropServices.RuntimeInformation.IsOSPlatform(OSPlatform.Linux))
{
// Execute Linux specific code
}
4. Does .NET Core support GUI applications on Linux and macOS?
While .NET Core’s support for GUI applications on non-Windows platforms has been limited, the new MAUI (Multi-platform App UI) framework introduced with .NET 6 addresses this. With MAUI, you can create native user interfaces that work on Windows, macOS, Android, and iOS.
Here’s a simple MAUI code sample:
// Use Microsoft.Maui.Controls;
var newButton = new Button { Text = "Click me" };
newButton.Clicked += (s, e) =>
{
newButton.Text = "Clicked!";
};
5. How can I ensure my .NET Core application’s dependencies are platform-independent?
Always check the compatibility of your libraries with your target platforms. Consider using libraries that comply with the .NET Standard, as they are designed to be platform independent. NuGet, the package manager for .NET, is a great resource for finding suitable libraries.
If you encounter a library that’s not compatible with your target platform, consider looking for alternatives or creating platform-specific implementations. It’s essential to address such issues early in the development cycle to avoid complications later.
6. What makes .NET Core platform independent?
.NET Core is platform-independent due to its modular design, open-source nature, and cross-platform runtime. The framework includes a set of libraries (known as CoreFX) and a runtime environment (CoreCLR), which can be executed on multiple operating systems.
The same .NET Core code can be run on Windows, Linux, or macOS without any modification.
Consider this simple .NET Core console application:
Console.WriteLine("Hello, .NET Core!");
This application will run on any platform where .NET Core runtime is installed.
7. What is meant by cross-platform in .NET Core?
Cross-platform in the context of .NET Core refers to the ability to build and run applications on several operating systems with the same code base. You can develop a .NET Core application on a Windows machine, for example, and run it on Linux or macOS without needing to modify the code.
8. Is .NET Core a framework or platform?
.NET Core is both a framework and a platform. As a platform, it provides a runtime environment (CoreCLR) where applications can be executed. As a framework, it offers a set of libraries (CoreFX) that developers can use to build applications.
Together, these elements allow .NET Core to provide the services necessary for developing and running applications.
9.Why ASP.NET Core is open source?
ASP.NET Core is open source for several reasons. First, making it open source encourages community contributions, which can speed up development, increase innovation, and improve code quality. Second, being open source promotes transparency and trust among users.
Developers can review the code, understand how it works, and have confidence in its security and reliability.
ASP.NET Core’s open-source nature also complements the cross-platform vision of .NET Core, allowing the framework to be used and improved by a broad, diverse group of developers across different platforms.
Here’s a simple ASP.NET Core code snippet demonstrating a basic controller:
public class HomeController : Controller { public IActionResult Index() { return View(); } }
This ASP.NET Core application will run on any platform that supports .NET Core.
10. Is .NET cross platform
Yes, .NET Core is indeed cross-platform, allowing developers to build applications that run on various operating systems including Windows, Linux, and macOS using the same codebase. This is in contrast to the traditional .NET Framework, which is Windows-specific.
This cross-platform capability has been a significant factor in .NET Core’s growing popularity, enabling organizations to target a broader range of environments and systems without needing to switch between different frameworks or languages.
Let’s take a look at an example of .NET Core’s cross-platform functionality.
using System; namespace CrossPlatformApp { class Program { static void Main(string[] args) { Console.WriteLine($"Hello from {System.Runtime.InteropServices.RuntimeInformation.OSDescription}!"); } } }
This is a simple console application that outputs the operating system’s description where it’s running. If you compile and run this application on Windows, it might output “Hello from Microsoft Windows 10.0.19042,” but if you run the same compiled binary on Ubuntu, it could say “Hello from Linux 5.4.0-65-generic #73-Ubuntu.”
This example demonstrates the core benefit of .NET Core’s cross-platform capability – code reuse across different operating systems without modifications. This is particularly advantageous when targeting cloud-based or containerized environments, which often involve various operating systems.
Remember, when writing cross-platform .NET Core code, always consider potential differences between OS environments, such as file paths, newline characters, or specific OS features.
Use .NET Core’s built-in capabilities to manage these differences when necessary, like Path.Combine
for building file paths that work on any OS, or Environment.NewLine
for newline characters that match the current OS.
string path = Path.Combine("folder1", "folder2"); // This will generate "folder1/folder2" on Unix-like systems, // and "folder1\\folder2" on Windows.
By leveraging .NET Core’s cross-platform features, developers can write software that’s more flexible, scalable, and capable of running on any modern computing platform.
By understanding these aspects of .NET Core, you’ll be better equipped to take advantage of its powerful features and capabilities as a platform-independent, cross-platform framework.
Wrapping Up: Embracing the Platform Independence of .NET Core
The claim of .NET Core’s platform independence isn’t an empty boast—it’s a proven fact, reinforced by a robust set of features and a diverse, worldwide community. As we surf through the ever-changing waves of technology, .NET Core shines as a symbol of adaptability, efficiency, and versatility.
So, whether you’re a skeptical observer or an ambitious developer ready to harness .NET Core’s cross-platform capabilities.

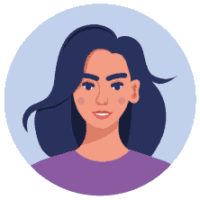
Jessica is a highly accomplished technical author specializing in scientific computer science. With an illustrious career as a developer and program manager at Accenture and Boston Consulting Group, she has made significant contributions to the successful execution of complex projects.
Jessica’s academic journey led her to CalTech University, where she pursued a degree in Computer Science. At CalTech, she acquired a solid foundation in computer systems, algorithms, and software engineering principles. This rigorous education has equipped her with the analytical prowess to tackle complex challenges and the creative mindset to drive innovation.
As a technical author, Jessica remains committed to staying at the forefront of technological advancements and contributing to the scientific computer science community. Her expertise in .NET C# development, coupled with her experience as a developer and program manager, positions her as a trusted resource for those seeking guidance and best practices. With each publication, Jessica strives to empower readers, spark innovation, and drive the progress of scientific computer science.