As a .NET developer, I’ve often been asked: “Why is Dot Net called a framework?”
This fundamental question piques the curiosity of many beginners in the field, so today, I’m going to provide an easy-to-understand, engaging exploration into the world of .NET.
/
To start we should first ask the fundamental question what exactly is a programming framework?
What are the differences between a programming language and a programming framework?
A programming language is a set of rules and syntax that allows developers to write instructions or commands that a computer can understand and execute. It defines the vocabulary and grammar for writing code.
On the other hand, a programming framework is a software platform or set of tools that provides a structured environment for building and developing applications. It includes libraries, components, and utilities that simplify and accelerate the development process. A programming framework often supports multiple programming languages and offers additional services and features beyond the capabilities of a programming language alone.
Here’s a comparison table highlighting the key differences between a programming language and a programming framework:
Programming Language | Programming Framework |
---|---|
Defines syntax, grammar, and rules for writing code. | Provides a structured platform and tools for application development. |
Focuses on writing code instructions that a computer can understand and execute. | Extends beyond the language and offers additional functionality, libraries, and services. |
Operates at a lower level of abstraction, closer to machine instructions. | Operates at a higher level of abstraction, providing pre-built components and utilities. |
Determines the vocabulary and grammar for expressing computations. | Simplifies and accelerates the development process by providing a structured environment. |
Tied to a specific syntax and grammar. | Supports multiple programming languages, allowing code written in different languages to work together. |
Examples: Java, Python, C++, JavaScript. | Examples: .NET, Ruby on Rails, Django, Angular, React. |
What are the features of .NET that mean we would consider it as a programming framework?
Dot Net is a framework rather than just a programming language due to the following features:
- Common Language Runtime (CLR): .NET includes the CLR, which provides services like memory management, exception handling, and security. These runtime services go beyond the capabilities of a programming language.
- Base Class Library (BCL): The .NET framework offers a comprehensive set of classes and libraries, known as the Base Class Library (BCL), which provides a wide range of pre-built functionality for tasks like file I/O, networking, data access, and more. These libraries extend the capabilities of the programming language.
- Language Interoperability: .NET supports multiple programming languages such as C#, Visual Basic .NET, F#, and more. This interoperability allows developers to write code in different languages that can seamlessly work together, leveraging the framework’s common runtime environment.
- Application Deployment and Configuration: .NET provides tools and mechanisms for deploying and configuring applications. It includes features like the Global Assembly Cache (GAC) for managing shared assemblies, configuration files for application settings, and deployment options that go beyond the scope of a programming language.
- Development Tools and Ecosystem: The .NET framework comes with a rich ecosystem of development tools, including integrated development environments (IDEs) like Visual Studio, code editors, debugging tools, and more. This tooling support enhances the development experience and extends beyond what a programming language alone can provide.
These features collectively make .NET more than just a programming language, establishing it as a robust programming framework that encompasses runtime services, libraries, language interoperability, deployment mechanisms, and a supportive development ecosystem.
Buckle up for our deep dive into the 21 reasons why
1. Language Interoperability
Dot Net Framework supports multiple programming languages, including C#, VB.NET, and F#. This language interoperability feature means that developers can choose the language they are most comfortable with, or even mix and match languages across different components of a single application.
// C# code public class Hello { public void SayHello() { Console.WriteLine("Hello from C#!"); } } // VB.NET code Public Class Hi Public Sub SayHi() Console.WriteLine("Hi from VB.NET!") End Sub End Class
The above snippets, though written in different languages, can interact seamlessly within the .NET framework.
2. Common Language Runtime (CLR)
The Common Language Runtime (CLR) is the heart of the .NET Framework, handling the execution of .NET programs. It provides a host of services that make the development process smoother and the running of applications more secure and robust.
Now, let’s examine the key features of the CLR:
1. Intermediate Language (IL) and Just-In-Time Compilation (JIT)
When you write and compile a .NET application, the source code is converted into an intermediate language, also known as Common Intermediate Language (CIL) or MSIL (Microsoft Intermediate Language).
This IL is a CPU-independent set of instructions that can be efficiently converted to native code.
public class HelloWorld
{
public static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
In the simple C# program above, when compiled, the Main method will be transformed into IL code that looks something like this using Assembly:
.method public hidebysig static void Main(string[] args) cil managed
{
.entrypoint
.maxstack 8
IL_0000: ldstr "Hello, World!"
IL_0005: call void [mscorlib]System.Console::WriteLine(string)
IL_000a: ret
}
The CLR then uses a Just-In-Time (JIT) compiler to convert the IL code to native code, specific to the computer architecture. This conversion happens right before execution, hence the name “Just-In-Time”.
2. Garbage Collection
The CLR automatically manages the allocation and release of memory for your application. It keeps track of all the objects in your .NET application, and when an object is no longer needed or reachable, the garbage collector releases the memory occupied by that object.
This process significantly reduces the chances of memory leaks in your application.
3. Security
The CLR offers a robust security system, known as Code Access Security (CAS). This system governs what your .NET application can and can’t do, based on various permissions set by policy. This level of security is beneficial in maintaining the integrity of your system and preventing malicious activities.
4. Exception Handling
CLR provides a structured exception handling model. This means that when exceptions occur, CLR provides mechanisms to gracefully recover from them, allowing your application to continue running smoothly.
try { int x = 10; int y = 0; int result = x / y; } catch (DivideByZeroException ex) { Console.WriteLine("Cannot divide by zero!"); }
In the above code, an attempt to divide by zero would usually crash a program. However, thanks to CLR’s exception handling mechanism, we can catch the DivideByZeroException
and handle it appropriately.
These features are some of the reasons why CLR is such a fundamental part of the .NET Framework.
As you can see, it handles many tasks automatically, thereby simplifying the job of a .NET developer and enabling them to focus more on business logic rather than on low-level programming details.
3. Base Class Library (BCL)
The Base Class Library is a set of common functionalities available to all languages using .NET. It’s a large collection of predefined classes, interfaces, structures, and methods that provide fundamental capabilities to your applications.
This reduces the amount of code that developers have to write from scratch, improving productivity and application robustness.
Let’s look at some examples of how the BCL simplifies common tasks.
1. File Operations
The System.IO
namespace contains types that enable reading and writing to files and data streams.
Here’s a simple example of creating a new file and writing some text into it:
using System.IO; File.WriteAllText("test.txt", "Hello, World!");
2. Data Structures
The System.Collections
namespace provides a number of data structures for organizing data.
Here’s an example of using a Dictionary<TKey,TValue>
to store key-value pairs:
using System.Collections.Generic; var capitals = new Dictionary<string, string> { {"USA", "Washington, D.C."}, {"UK", "London"}, {"India", "New Delhi"} };
3. Networking
The System.Net
namespace provides a simple API for sending and receiving data over a network.
Here’s an example of downloading a webpage:
using System.Net; WebClient client = new WebClient(); string htmlCode = client.DownloadString("http://example.com");
4. Threading
The System.Threading
namespace provides types for multi-threaded programming.
Here’s an example of starting a new thread:
using System.Threading; Thread newThread = new Thread(() => { Console.WriteLine("Hello from a new thread!"); }); newThread.Start();
5. Database Access
The System.Data
namespace provides types for working with data sources like databases and XML files.
Here’s an example of connecting to a SQL Server database and executing a command:
using System.Data.SqlClient; string connectionString = "Server=(local);Database=TestDB;Integrated Security=True"; using (SqlConnection connection = new SqlConnection(connectionString)) { connection.Open(); SqlCommand command = new SqlCommand("SELECT * FROM Users", connection); SqlDataReader reader = command.ExecuteReader(); }
These are just a few examples that highlight the power and diversity of the Base Class Library (BCL) in .NET. Whether you’re manipulating strings, accessing databases, performing file I/O operations, or anything else, chances are the BCL has functionality that makes your task easier.
As a developer, the BCL becomes your go-to toolset for a wide variety of common programming tasks.
4. Consistent Programming Model
.NET provides a consistent object-oriented programming model. This consistent approach simplifies the development process as developers can anticipate how to use different components based on their experience with others.
5. Security
.NET comes with a robust security model that prevents unauthorized access and stops malicious activities. This built-in security model is another feature that qualifies .NET as a framework.
6. Development Tools
Microsoft offers powerful development tools like Visual Studio for .NET, which make coding, debugging, and deploying applications easier. This comprehensive IDE contributes to the framework’s robustness.
7. Platform Independence
.NET Core, a part of the .NET Framework, allows developers to create apps that can run on multiple platforms like Windows, Linux, and macOS, further bolstering the versatility of this framework.
8. Networking
.NET Framework includes libraries for network programming. These enable applications to send and receive data over the internet, interact with firewalls, and perform other network-related tasks.
9. Web Services Support
.NET Framework supports the creation and use of XML Web services, allowing applications to communicate and share data over the internet, regardless of operating system or programming language.
10. ASP.NET for Web Development
ASP.NET, a part of the .NET Framework, provides a model for building robust web applications. It includes features like authentication, state management, and a server-side event model.
public class HomeController : Controller { public IActionResult Index() { return View(); } }
In this code snippet, you can see the simplicity of handling HTTP requests with ASP.NET.
11. Integrated Development Environment (IDE) Support
With .NET, developers can use any IDE they prefer. While Visual Studio is the most popular choice due to its advanced features and direct support from Microsoft, other IDEs such as Rider or Visual Studio Code can also be used to write .NET code.
12. Windows Forms and WPF for Desktop Applications
Windows Forms and Windows Presentation Foundation (WPF) allow developers to build rich desktop applications. They offer a wide range of controls and support for complex UI requirements.
public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); } }
The above WPF example illustrates how easy it is to set up a window in a desktop application.
13. Multithreading
.NET provides extensive support for multithreading. This allows for concurrent execution, making applications faster and more responsive.
14. Version Compatibility
.NET ensures backward compatibility, meaning that applications built with an older version of .NET will continue to run smoothly on newer versions of the framework.
15. Microsoft Support
.NET is backed by Microsoft, a technology giant known for its comprehensive developer support. This support is a significant advantage for .NET developers.
16. Open Source
.NET Core, the cross-platform sibling of .NET Framework, is open source. This enables community contributions, making the framework continually evolve and improve.
17. Middleware Components
In ASP.NET Core, middleware components handle requests and responses. Developers can configure how these components interact, creating a customizable pipeline for web requests.
public void Configure(IApplicationBuilder app)
{
app.UseMiddleware<MyCustomMiddleware>();
}
The above snippet shows how to use a custom middleware in an ASP.NET Core application.
18. Entity Framework
Entity Framework (EF) is an open-source object-relational mapping (ORM) framework for .NET. It allows developers to work with databases using .NET objects, eliminating the need for most of the data access code that developers usually need to write.
Entity Framework simplifies data manipulation by abstracting the relational logic and offering an object-centric model, allowing developers to focus on the business logic of the application.
1. Database Operations
Entity Framework abstracts a lot of boilerplate code required for database operations. For example, to query data, you can use LINQ (Language Integrated Query) instead of writing SQL queries.
Here’s an example of how you can retrieve data from a database:
using (var context = new MyDbContext()) { var customers = context.Customers.Where(c => c.City == "London").ToList(); }
In the example above, you’re querying the Customers
table in the database for all customers whose City
is “London”. Notice that the query is written in C# and not SQL, making it easier to work with for .NET developers.
2. Code-First Development
Entity Framework supports a development paradigm called “Code-First”. This approach allows developers to focus on the domain design and start creating classes as per business requirements. Based on these classes, Entity Framework can generate a database automatically.
Here’s an example of how you can define classes that map to your database:
public class Customer { public int CustomerId { get; set; } public string Name { get; set; } public string City { get; set; } }
In the class above, each instance of the Customer
class corresponds to a row in the Customers
table in the database, and each property of the class corresponds to a column in the table.
3. Database Migrations
Another powerful feature of Entity Framework is its ability to handle database schema migrations. When you change your code, Entity Framework can automatically update your database schema to match your code.
Here’s how you can create a new migration:
Add-Migration AddCustomerCity
The command above will create a new migration that adds the City
property to the Customers
table.
4. Lazy Loading
Entity Framework provides “lazy loading” out of the box. This means that the related data is automatically fetched from the database when you access a navigation property.
using (var context = new MyDbContext()) { var customer = context.Customers.First(); Console.WriteLine(customer.Orders.Count); }
In the example above, the Orders
related to the first Customer
are automatically loaded from the database when you access the Orders
property.
Here’s a summarized view of the Entity Framework discussion in a tabular format.
Entity Framework Feature | Description | Example |
---|---|---|
Database Operations | Entity Framework abstracts most of the data access code, allowing developers to query and manipulate data using .NET objects. | var customers = context.Customers.Where(c => c.City == "London").ToList(); |
Code-First Development | This approach enables developers to define classes that map to database tables. Entity Framework can then automatically generate a corresponding database. | public class Customer { public int CustomerId { get; set; } public string Name { get; set; } public string City { get; set; } } |
Database Migrations | Entity Framework can automatically manage database schema changes, providing a simple way to version your database and keep it in sync with your code. | Add-Migration AddCustomerCity (This command in Entity Framework CLI creates a new migration named AddCustomerCity .) |
Lazy Loading | This feature allows Entity Framework to automatically load related data from the database when you access a navigation property. | var customer = context.Customers.First(); Console.WriteLine(customer.Orders.Count); (In this example, Orders related to the first Customer are automatically loaded when you access the Orders property.) |
This table provides a quick overview of the core features of Entity Framework and how they can simplify data access in .NET applications.
In summary, Entity Framework is a powerful tool in the .NET Framework that simplifies data access, abstracts complex database operations, and allows developers to work with data in a more .NET-friendly way.
19. Unit Testing
.NET promotes the practice of unit testing, with built-in tools like MSTest, NUnit, and xUnit that allow developers to write tests for their applications. This encourages the creation of robust, error-free software.
20. Cloud Support
.NET seamlessly integrates with cloud platforms like Azure, facilitating the development of modern, scalable applications. This integration enhances the power and reach of the .NET framework.
21. Performance in .NET
The Dot Net Framework offers impressive performance, owing to several key features. These include Just-In-Time Compilation (JIT), Garbage Collection, and a powerful set of optimized Base Class Libraries. But beyond these, there are other factors at play that can enhance the performance of a .NET application.
1. Just-In-Time Compilation (JIT)
As we mentioned before, the Dot Net Framework compiles code Just-In-Time (JIT) before executing it. This compilation converts Intermediate Language (IL) code into machine code that’s specific to the computer’s architecture, which can be executed very quickly.
JIT also applies several optimizations during this process, such as inlining (replacing a function call site with the body of the called function), which often results in performance improvements.
2. Garbage Collection
The .NET Garbage Collector (GC) helps optimize application performance by automatically managing memory. The GC frees up memory from objects that are no longer in use, allowing your application to use this memory elsewhere, reducing the risk of out-of-memory errors.
3. Span and Memory
.NET Core introduced Span
Span<byte> span = new byte[100];
In the above example, a Span<byte>
is created, providing a type-safe and memory-safe representation of a contiguous region of arbitrary memory.
4. Hardware Intrinsics
.NET Core also introduced support for hardware intrinsics, which are specialized CPU instructions that can greatly improve performance for certain types of operations, such as numerical computations or text processing.
if (Avx2.IsSupported) { // Use AVX2 hardware intrinsics } else if (Sse2.IsSupported) { // Use SSE2 hardware intrinsics } else { // Use software fallback }
In the above code, we check if certain sets of hardware intrinsics are supported before using them, providing fallbacks if they’re not.
5. Asynchronous Programming
The Dot Net Framework provides extensive support for asynchronous programming, allowing applications to perform other work while waiting for operations to complete, leading to more efficient use of system resources and improved performance, especially in I/O-intensive applications.
public async Task<string> FetchDataFromApiAsync(string url) { using HttpClient client = new HttpClient(); string result = await client.GetStringAsync(url); return result; }
In the example above, the FetchDataFromApiAsync
method retrieves data from a URL asynchronously, freeing up system resources while waiting for the HTTP request to complete.
By understanding and utilizing these performance-oriented features, developers can ensure that their .NET applications are not only powerful and versatile, but also incredibly efficient.
Here is a summary of the main points discussed in this article so far, put into a tabular format:
Topic | Key Points | Example |
---|---|---|
.NET Framework | A software framework developed by Microsoft, providing a unified programming model for building applications on Windows. | N/A |
Common Language Runtime (CLR) | Manages the execution of .NET programs, enabling features such as cross-language integration, exception handling, and enhanced security. | int result = int.Parse("123"); // CLR handles the conversion from string to int |
Base Class Library (BCL) | A set of predefined classes, interfaces, structures, and methods that provide fundamental capabilities to your applications. | Dictionary<string, string> capitals = new Dictionary<string, string> { { "USA", "Washington, D.C." }, { "UK", "London" }, { "India", "New Delhi" } }; |
Performance | The .NET Framework offers impressive performance due to JIT Compilation, Garbage Collection, Span | public async Task<string> FetchDataFromApiAsync(string url) { using HttpClient client = new HttpClient(); string result = await client.GetStringAsync(url); return result; } |
Entity Framework | An ORM framework that allows developers to work with databases using .NET objects, abstracting the relational logic. | var customers = context.Customers.Where(c => c.City == "London").ToList(); |
Please note that this table is a condensed summary and does not capture all the information and nuance discussed in the article. For a full understanding, please refer to the detailed sections.
Wrapping Up about Why Dot Net is Called a Framework
Each of these 21 reasons underlines why Dot Net is called a framework. It provides a foundational structure that supports and simplifies various facets of software development, making it an excellent choice for developers worldwide.
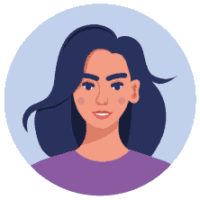
Jessica is a highly accomplished technical author specializing in scientific computer science. With an illustrious career as a developer and program manager at Accenture and Boston Consulting Group, she has made significant contributions to the successful execution of complex projects.
Jessica’s academic journey led her to CalTech University, where she pursued a degree in Computer Science. At CalTech, she acquired a solid foundation in computer systems, algorithms, and software engineering principles. This rigorous education has equipped her with the analytical prowess to tackle complex challenges and the creative mindset to drive innovation.
As a technical author, Jessica remains committed to staying at the forefront of technological advancements and contributing to the scientific computer science community. Her expertise in .NET C# development, coupled with her experience as a developer and program manager, positions her as a trusted resource for those seeking guidance and best practices. With each publication, Jessica strives to empower readers, spark innovation, and drive the progress of scientific computer science.