Dot Net Core Azure best practices transform your cloud deployment, ensuring seamless scalability, top-notch security, and efficient resource management.
As more and more businesses move their applications to the cloud, it’s essential to optimize your .NET Core applications for Azure services for achieving optimal performance.
Choosing the Right Azure Services for .NET Core Applications
Optimizing .NET Core applications for Azure services involves careful consideration of the various hosting and deployment options available. Azure provides a multitude of services that can be used to build, run, and scale .NET Core applications, each with distinct advantages and disadvantages depending on the application requirements.
When selecting the appropriate Azure services for .NET Core applications, developers must consider factors like scalability, availability, and cost-effectiveness. Some of the key Azure services to consider for .NET Core applications include:
Service | Description |
---|---|
Azure Virtual Machines | Provides scalable, on-demand computing resources for .NET Core applications, giving developers complete control over the server and operating system configurations. |
Azure App Service | Enables easy deployment and management of .NET Core applications, supporting multiple deployment options such as containerization and integration with DevOps tools. |
Azure Kubernetes Service | Offers a highly scalable and customizable container orchestration platform for .NET Core applications, supporting automation and self-healing capabilities. |
Azure Functions | Allows developers to quickly create event-driven, serverless computing solutions for .NET Core applications, enabling rapid scaling and cost savings. |
Azure SQL Database | Provides a fully managed, scalable relational database solution for .NET Core applications, offering high availability and disaster recovery options. |
Choosing the right Azure services for .NET Core applications is critical to achieving optimal performance and scalability. By taking into account the specific needs of the application and the capabilities of each Azure service, developers can make informed decisions about which services to use and how to use them effectively.
Designing Scalable and Resilient Architectures with .NET Core on Azure
When designing .NET Core applications on Azure, it’s crucial to consider scalability and resiliency. By implementing best practices, you can ensure that your application can handle traffic spikes and recover from failures.
Load balancing is a critical component of a scalable architecture. By distributing traffic across multiple instances of your application, you can handle more requests without overwhelming any single instance. In Azure, you can use Azure Load Balancer or Application Gateway to achieve load balancing.
Auto-scaling is another important technique for handling variable traffic loads. By configuring auto-scaling, your application can automatically adjust the number of instances based on changes in traffic. Azure offers a variety of options for auto-scaling, including Virtual Machine Scale Sets and App Service AutoScale.
Fault tolerance and disaster recovery are also crucial considerations for any cloud-based application. By designing for fault tolerance, you can ensure that your application can continue to function even if individual components fail.
This can be achieved through techniques such as redundancy and failover. Disaster recovery involves having a plan in place to quickly recover from a catastrophic failure, such as a region-wide outage. Azure provides several options for disaster recovery, including Azure Site Recovery and Azure Backup.
Below is an example of how to implement load balancing with Azure Load Balancer:
// Define the backend pool with the endpoints of your instances
var backendAddressPool = new List<string> { "10.0.0.4", "10.0.0.5", "10.0.0.6" };
// Define the load balancer rule
var loadBalancerRule = new LoadBalancerRule
{
Name = "MyLoadBalancerRule",
FrontendIPConfiguration = frontendIPConfig,
BackendAddressPool = backendAddressPool,
Protocol = TransportProtocol.Tcp,
FrontendPort = 80,
BackendPort = 80
};
// Create the load balancer
var loadBalancer = azure.LoadBalancers.Define("MyLoadBalancer")
.WithRegion(Region.USWest)
.WithExistingResourceGroup("MyResourceGroup")
.WithFrontend(frontendIPConfig)
.DefineLoadBalancingRule(loadBalancerRule)
.Create();
Implementing these best practices for scalability and resiliency can help ensure that your .NET Core application on Azure can handle traffic spikes and recover from failures.
Security Best Practices for .NET Core Applications on Azure
When it comes to security, there are several best practices to keep in mind for .NET Core applications running on Azure. These practices can help prevent security breaches and ensure that sensitive data remains secure.
Secure Authentication and Authorization
One essential security practice for .NET Core applications on Azure is to implement secure authentication and authorization mechanisms. This can include multi-factor authentication, role-based access control, and implementing secure communication protocols like HTTPS.
It’s important to use industry-standard methods for secure authentication and authorization. For example, using OAuth 2.0 or OpenID Connect for token-based authentication can provide a more secure authentication mechanism than traditional username/password authentication.
Data Encryption
Another important practice for securing .NET Core applications on Azure is to use encryption to protect sensitive data. This can include using encryption algorithms like AES or RSA to encrypt data at rest, as well as encrypting data in transit using SSL or TLS.
In addition to encrypting data, it’s also important to implement secure key management practices to ensure that encryption keys are protected and not easily accessible to unauthorized parties.
Network Security
Ensuring network security is also a crucial aspect of securing .NET Core applications on Azure. This can include implementing firewalls and network security groups to control access to resources, as well as using Azure Virtual Networks to isolate applications from each other.
It’s important to regularly monitor network traffic and logs to detect any suspicious activity and take appropriate action to prevent security breaches.
Industry Standards and Trends
Staying up-to-date with the latest security standards and trends in the industry is also important for ensuring the security of .NET Core applications on Azure. For example, incorporating DevSecOps practices can help ensure that security is built into the development and deployment process from the beginning.
Microsoft also provides several resources for developers to help ensure the security of their applications on Azure, including Azure Security Center and Azure Advisor.
Performance Optimization Techniques for .NET Core on Azure
When it comes to deploying .NET Core applications on Azure, achieving optimal performance is crucial. Not only does it ensure a seamless user experience, but it also contributes to cost-effectiveness and scalability. In this section, we will explore some performance optimization techniques for .NET Core on Azure.
Caching
Caching frequently used data can significantly improve the performance of your .NET Core application on Azure. Azure provides various caching options, including Azure Cache for Redis and Azure Managed Cache, which can be easily integrated into your application. You can also leverage the MemoryCache and DistributedMemoryCache features in .NET Core for in-memory caching.
Here’s an example of using Azure Cache for Redis in a .NET Core application:
// Creating a Redis cache connector
var cacheConnection = ConnectionMultiplexer.Connect("redis-cache.
redis.cache.windows.net:6380,password=yourRedisPassword,ssl=True,abortConnect=False");
// Retrieving a cached value using key "myKey"
var db = cacheConnection.GetDatabase();
var cachedValue = await db.StringGetAsync("myKey");
// Caching a value with key "myKey" for 10 minutes
var cacheOptions = new DistributedCacheEntryOptions()
.SetSlidingExpiration(TimeSpan.FromMinutes(10));
await cache.SetStringAsync("myKey", "myValue", cacheOptions);
Database Optimization
Optimizing your database for performance can also enhance the overall performance of your .NET Core application on Azure. This can be achieved by implementing techniques like indexing, caching, and reducing database calls. Azure offers various database options like Azure SQL Database and Azure Cosmos DB, which provide scalability and high availability.
Here’s an example of using Azure SQL Database in a .NET Core application:
// Creating a SQL connection
var connectionString = "Server=tcp:myserver.database.
windows.net,1433;Initial Catalog=mydatabase;Persist Security Info=False;User ID=myusername;Password=mypassword;MultipleActiveResultSets=False;Encrypt=True;TrustServerCertificate=False;Connection Timeout=30;"
var connection = new SqlConnection(connectionString);
// Retrieving data from the "Users" table
var query = "SELECT * FROM Users";
var command = new SqlCommand(query, connection);
connection.Open();
var reader = command.ExecuteReader();
while (reader.Read())
{
var name = reader["Name"].ToString();
// Do something with the retrieved data
}
connection.Close();
Asynchronous Programming
Using asynchronous programming techniques like async/await can improve the performance of your .NET Core application on Azure by allowing it to handle more requests simultaneously. This can be especially useful for I/O-bound operations like accessing a database or calling an external API. You can also take advantage of Azure’s asynchronous services like Azure Functions for improved performance.
Here’s an example of using async/await in a .NET Core application:
// Retrieving data asynchronously from an external API
using (var httpClient = new HttpClient())
{
httpClient.BaseAddress = new Uri("https://api.example.com");
var response = await httpClient.GetAsync("users");
var content = await response.Content.ReadAsStringAsync();
// Do something with the retrieved data
}
}
Load Testing
Load testing your .NET Core application on Azure can help identify performance issues and bottlenecks. Azure provides load testing services like Azure DevOps Load Testing and Visual Studio Load Testing, which can be integrated into your deployment pipeline.
Here’s an example of load testing a .NET Core application on Azure:
// Creating a load test using Azure DevOps
var loadTest = new LoadTest()
.WithUserAgent("Chrome")
.WithRampUp(TimeSpan.FromMinutes(5))
.WithLoadPattern(SpikeLoadPattern.Create(100, TimeSpan.FromSeconds(10),
TimeSpan.FromMinutes(1)))
.WithDuration(TimeSpan.FromMinutes(30))
.WithCounter("Requests/sec", CounterLocation.Server, "localhost")
.WithCounter("Memory Usage", CounterLocation.Process, "w3wp")
.WithCounter("% Processor Time", CounterLocation.Process, "w3wp");
// Starting the load test
var result = await loadTest.RunAsync();
// Analyzing the load test result
var requestsPerSecond = result.PerformanceCounters.GetCounter("Requests/sec").Average;
var memoryUsage = result.PerformanceCounters.GetCounter("Memory Usage").Average;
var processorTime = result.PerformanceCounters.GetCounter("% Processor Time").Average;
By implementing these performance optimization techniques and leveraging Azure’s services, you can achieve optimal performance for your .NET Core applications on Azure.
Monitoring and Diagnosing .NET Core Applications on Azure
Monitoring and diagnosing .NET Core applications on Azure is crucial for ensuring peak performance and identifying potential issues before they become critical. Azure provides a range of monitoring and diagnostic tools, including Azure Monitor, Azure Application Insights, and Azure Log Analytics.
Azure Monitor helps track the performance and health of applications and infrastructure with customizable alerts and dashboards. Application Insights provides deep insights into application performance, including user sessions, page views, and exceptions. Log Analytics enables real-time log analysis and troubleshooting across multiple sources.
Setting up alerts and analyzing logs are essential for identifying issues with .NET Core applications on Azure. Azure Monitor enables setting up alerts based on metrics, logs, and events, allowing for proactive resolution of issues before they impact users. Azure Application Insights provides detailed telemetry, including exceptions, traces, and custom events. Azure Log Analytics enables real-time log analysis and correlation, enabling fast resolution of issues by identifying patterns and anomalies.
Debugging tools like Visual Studio’s Azure Debugger and Azure Monitor’s Application Insights Snapshot Debugger facilitate debugging .NET Core applications on Azure, allowing developers to pinpoint issues in production environments without affecting users. Tracing and profiling tools like Azure Application Insights Profiler help identify performance bottlenecks and optimize application performance.
Continuous Integration and Deployment for .NET Core on Azure
Implementing continuous integration and deployment (CI/CD) practices is essential for ensuring seamless deployment of .NET Core applications on Azure. Azure DevOps is a comprehensive platform that offers a range of tools and services for agile development and deployment.
Version control is a critical aspect of CI/CD. Azure DevOps provides integration with Git and other version control systems, allowing developers to track code changes and collaborate efficiently. Build pipelines can be set up to automate the build process, including compiling, testing, and packaging the application.
Release management is another critical feature of Azure DevOps. Developers can define release pipelines that deploy applications to different environments, such as development, staging, and production. These pipelines can be customized to include approval workflows, manual interventions, and automated testing.
Leveraging containerization can also enhance the deployment process. Azure offers integration with Docker, allowing developers to package applications as containers and deploy them to Azure Kubernetes Service (AKS) clusters. This approach enables fast and consistent deployment, improved scalability, and easier management of application infrastructure.
By adopting CI/CD practices and leveraging Azure DevOps, developers can streamline the deployment process, increase productivity, and minimize downtime and errors.
Leveraging Azure DevOps for .NET Core Projects
Azure DevOps is a powerful platform that provides end-to-end solutions for managing the entire software development lifecycle. It offers a variety of features that can enhance development productivity, including source control management, continuous integration, continuous delivery, and more.
Version Control with Azure Repos
Azure Repos is a fully-featured source control management system that can handle both Git and Team Foundation Version Control (TFVC) repositories. With Azure Repos, you can easily manage your codebase, collaborate with other developers, and track changes.
To use Azure Repos, start by creating a new repository in Azure DevOps. Then, clone the repository locally and start adding your code. You can use Git commands like commit, push, and pull to manage changes.
Example:
git add .
git commit -m "Added new feature"
git push origin master
Build Pipelines with Azure Pipelines
Azure Pipelines provides a complete continuous integration and continuous delivery (CI/CD) solution that integrates seamlessly with Azure DevOps. With Azure Pipelines, you can create build pipelines that automatically compile, test, and package your code whenever changes are made.
To create a build pipeline, start by defining the build steps in a YAML file. You can specify the build environment, the build tasks, and the output artifacts. Then, configure the pipeline to trigger whenever changes are made to your code.
Example:
- task: DotNetCoreCLI@2
displayName: 'Build project'
inputs:
command: 'build'
projects: '**/*.csproj'
arguments: '--configuration $(buildConfiguration)'
Release Management with Azure Release Pipelines
Azure Release Pipelines provide a powerful release management solution that enables you to deploy your code to any environment with ease. With Release Pipelines, you can create deployment pipelines that are triggered automatically when a build is completed.
To create a release pipeline, start by defining the release stages and the deployment tasks in a YAML file. You can specify the deployment environment, the deployment tasks for each stage, and the release artifacts. Then, configure the pipeline to trigger whenever a build is completed.
Example:
- stage: DeployToTest
displayName: 'Deploy to Test'
jobs:
- deployment: DeployWebApp
environment: 'Test'
strategy:
runOnce:
preDeploy:
- task: AzureWebApp@1
inputs:
azureSubscription: $(azureSubscription)
appName: 'mywebapp-test'
package: '$(System.DefaultWorkingDirectory)/**/*.zip'
With these Azure DevOps features, you can optimize your .NET Core development process and achieve greater efficiency and productivity.
Advanced Features and Integration with Azure Services
When optimizing .NET Core applications for Azure services, it’s important to explore the advanced features and integration options available. Azure App Service extensions, Azure Functions, Azure Storage, and Azure Cognitive Services are just a few of the many options available.
Azure App Service Extensions
Azure App Service extensions provide a way to customize the behavior of Azure App Service instances. These extensions can be used to add new functionality, enhance existing features, and configure settings for Azure App Service. For example, you can use the Application Insights extension to monitor the performance of your application, or the Azure Security Center extension to improve your application’s security posture.
Azure Functions
Azure Functions provide a serverless computing platform for building event-driven applications. They can be used to process data, integrate with other services, and trigger other Azure resources. Functions support a range of programming languages and provide a simple, pay-per-use pricing model. For example, you can use Azure Functions to process messages from an Azure Service Bus queue or to execute custom logic when a new file is added to Azure Storage.
Azure Storage
Azure Storage provides a scalable and highly available storage platform for applications. It supports a range of storage options, including blob storage, file storage, and table storage. Azure Storage can be used to store and retrieve data, host static websites, and provide shared access to files. For example, you can use Azure Storage to store and retrieve user data for your application or to host static assets like images and videos.
Azure Cognitive Services
Azure Cognitive Services provide prebuilt AI and machine learning capabilities that can be easily integrated into applications. They allow developers to add features like language understanding, image analysis, and speech recognition to their applications without requiring any AI or machine learning expertise. For example, you can use Azure Cognitive Services to analyze customer sentiment in social media posts or to transcribe audio from a video.
By leveraging these advanced features and integration options, .NET Core applications can be optimized for Azure services and provide new and enhanced functionality for users.
Testing and Debugging Strategies for .NET Core on Azure
Testing and debugging are crucial components of any software development process. In the context of .NET Core applications on Azure, it’s important to have solid strategies in place to ensure the application is functioning as expected and to identify and fix any issues that arise.
Unit Testing
Unit testing involves testing individual components or modules of the application to ensure they are functioning correctly. In .NET Core, unit tests can be written using the built-in testing framework, Xunit. Xunit provides a simple and easy-to-use API for creating unit tests.
Here’s an example:
// myclass.cs
public class MyClass
{
public int Add(int x, int y)
{
return x + y;
}
}
// myclass_test.cs
public class MyClassTest
{
[Fact]
public void Add_ReturnsCorrectSum()
{
// Arrange
var myclass = new MyClass();
int x = 5;
int y = 7;
// Act
int result = myclass.Add(x, y);
// Assert
Assert.Equal(12, result);
}
}
Integration Testing
Integration testing involves testing how various components of the application interact with each other. In .NET Core on Azure, integration tests can be automated using Azure DevOps. Azure DevOps provides a suite of tools for continuous integration and delivery, including the ability to run tests automatically.
Here’s an example of an integration test:
// integration_test.cs
public void IntegrationTest()
{
// Arrange
var client = new HttpClient();
client.BaseAddress = new Uri("http://localhost:5000/");
// Act
HttpResponseMessage response = await client.GetAsync("api/values");
response.EnsureSuccessStatusCode();
string responseString = await response.Content.ReadAsStringAsync();
// Assert
Assert.Contains("value1", responseString);
Assert.Contains("value2", responseString);
}
Performance Testing
Performance testing involves testing the application’s response time and throughput under various load conditions. In .NET Core on Azure, performance testing can be automated using tools like Apache JMeter or Visual Studio Load Testing. Here’s an example of a performance test in Apache JMeter:
Thread Group | HTTP Request | Assertions |
---|---|---|
50 Users, Ramp-Up Period 10 seconds | GET /api/values | Response Time: Less than 1 second |
100 Users, Ramp-Up Period 20 seconds | GET /api/values | Response Time: Less than 1.5 seconds |
200 Users, Ramp-Up Period 30 seconds | GET /api/values | Response Time: Less than 2 seconds |
Azure Debugging Tools
Azure provides a suite of tools for debugging .NET Core applications, including Application Insights and Log Analytics. These tools allow developers to monitor application performance in real-time, identify and diagnose issues, and analyze system logs. Here’s an example of using Application Insights:
public void SomeMethod()
{
try
{
// Some code that may throw an exception
}
catch(Exception ex)
{
var telemetry = new TelemetryClient();
telemetry.TrackException(ex);
}
}
Implementing these testing and debugging strategies can help ensure that your .NET Core applications on Azure are performing optimally and delivering the user experience expected.
Implementing Azure best practices for .NET Core applications is crucial for optimizing performance on Azure services. By choosing the right Azure services based on application requirements and developing scalable and resilient architectures, developers can ensure their applications run smoothly and efficiently on the cloud.
External Resources
FAQ
FAQ 1: How to Implement Azure AD Authentication in .NET Core Apps?
Q: What is a best practice for implementing Azure Active Directory (AD) authentication in a .NET Core application?
A: Utilizing the Microsoft Identity platform (Azure AD) for authentication is a common best practice. This involves configuring your .NET Core app to use Azure AD for user authentication.
Code Sample:
// Configuration in Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthentication(AzureADDefaults.AuthenticationScheme)
.AddAzureAD(options => Configuration.Bind("AzureAd", options));
}
FAQ 2: Best Practices for Managing App Settings and Secrets in .NET Core on Azure?
Q: How should I manage application settings and secrets in a .NET Core application hosted on Azure?
A: Use Azure Key Vault for managing application secrets and Azure App Configuration for settings. This ensures security and flexibility in configuration management.
Code Sample:
// Retrieve a secret from Azure Key Vault
var secretValue = Configuration["MySecret"];
FAQ 3: How to Optimize Azure SQL Database Performance in .NET Core?
Q: What are best practices for optimizing Azure SQL Database performance in a .NET Core application?
A: Ensure efficient database interactions by using Entity Framework Core’s best practices, such as async operations, efficient querying, and connection pooling.
Code Sample:
// Async query example using Entity Framework Core
public async Task<List<MyEntity>> GetEntitiesAsync()
{
return await _context.MyEntities.ToListAsync();
}
FAQ 4: Best Practices for Azure Service Bus Integration in .NET Core?
Q: What is the recommended way to integrate Azure Service Bus in a .NET Core application?
A: Use the Azure.Messaging.ServiceBus library for robust and scalable messaging between your services. Handle exceptions and ensure message processing idempotency.
Code Sample:
// Sending a message to an Azure Service Bus queue
var client = new ServiceBusClient(connectionString);
var sender = client.CreateSender(queueName);
await sender.SendMessageAsync(new ServiceBusMessage("Hello, World!"));
FAQ 5: How to Ensure Scalability and Resilience in .NET Core Azure Apps?
Q: What are the key considerations for scalability and resilience in .NET Core applications on Azure?
A: Implement Azure’s scalability features like Azure App Service Auto-Scaling and Azure Load Balancer. Use resilience patterns like retries, circuit breakers, and bulkheads.
Code Sample:
// Implementing a retry policy with Polly
var retryPolicy = Policy.Handle<HttpRequestException>()
.WaitAndRetryAsync(retryCount: 3, sleepDurationProvider: _ => TimeSpan.FromSeconds(2));
await retryPolicy.ExecuteAsync(async () =>
{
// HTTP request or other operation
});
These FAQs and code samples provide a snapshot of best practices for integrating various Azure services and features in a .NET Core application, focusing on security, performance, messaging, and scalability.
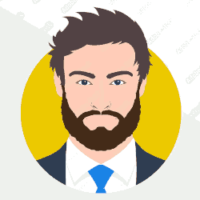
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.