Are you looking to take your .NET Core development to the next level? Integrating artificial intelligence (AI) capabilities into your projects could be the key. Using AI with .NET Core can enhance the performance and functionality of your applications and provide new opportunities for innovation.
AI-driven enhancements can be utilized in various ways in .NET Core development, such as improving predictive analytics, natural language processing, or image recognition, among other possibilities. Incorporating AI features into .NET Core projects will not only make your applications smarter but also future-proof them for the evolving technology landscape.
Keep reading to learn how to use AI with .NET Core and take your applications to the next level!
AI in .NET Core
Artificial Intelligence (AI) has become an essential aspect of modern software development. AI-driven enhancements can help developers to build smarter and more efficient applications that can learn and optimize their performance over time. As .NET Core continues to gain popularity among developers, integrating AI capabilities with .NET Core development has become a crucial consideration.
AI-driven enhancements are designed to add more intelligence to software applications. These enhancements utilize various machine learning algorithms to enable software to learn and optimize their behavior based on patterns in data. In the context of .NET Core development, AI can be used to enhance a range of features, including data processing and analytics, natural language processing, image recognition, and more.
Integrating AI with .NET Core development offers several benefits, including enhanced performance, increased efficiency, and more intelligent decision-making. With the right AI technologies integrated into .NET Core applications, developers can build applications that offer improved functionality, better user experiences, and more innovative features.
AI-Powered Enhancements in .NET Core Development
AI technologies are incredibly versatile, and there are many different ways to integrate them with .NET Core development.
Some of the most common AI-driven enhancements in .NET Core development include:
- Using machine learning algorithms to analyze and process large volumes of data more efficiently.
- Integrating natural language processing (NLP) capabilities to enable applications to interact more intelligently with users.
- Implementing computer vision algorithms to enable applications to identify and analyze visual data.
By incorporating these and other AI-driven enhancements into .NET Core development, developers can create smarter, more efficient, and more effective applications that can improve many different aspects of performance and run more efficiently with less resource usage.
Getting Started with AI in .NET Core
Integrating AI features into .NET Core projects can seem daunting, but with proper guidance, it’s easier than it seems. Before diving into AI-driven enhancements, there are some prerequisites to cover to ensure a seamless integration.
The first step is installing the necessary libraries and frameworks. Microsoft’s ML.NET and TensorFlow.NET are two popular options. Both libraries are built specifically for .NET Core and provide extensive documentation and support. Once installed, developers can begin using AI features, such as image recognition or natural language processing, by referencing these libraries in their code.
For example, to use the image recognition features provided by ML.NET, developers can add the following code to their project:
//Load data
var data = mlContext.Data.LoadFromEnumerable(images);
//Transform data
var imageData = mlContext.Transforms.LoadImages(outputColumnName: "image", imageFolder: "./images", inputColumnName: nameof(ImageData.ImagePath))
.Append(mlContext.Transforms.ResizeImages(outputColumnName: "image", imageWidth: ImageNetSettings.imageWidth, imageHeight: ImageNetSettings.imageHeight, inputColumnName: "image"))
.Append(mlContext.Transforms.ExtractPixels(outputColumnName: "input", inputColumnName: "image"));
//Load model
var model = mlContext.Model.Load(modelPath, out var schema);
//Predict using model
var predictionEngine = mlContext.Model.CreatePredictionEngine(model);
foreach (var image in images)
{
var imagePrediction = predictionEngine.Predict(image);
Console.WriteLine($"{image.Filename} predicted as
{imagePrediction.PredictedLabelValue} with score {imagePrediction.Score.Max()}");
}
This code loads and transforms the image data, loads the model, and makes a prediction using the model. With just a few lines of code, developers can leverage AI to classify images.
Overall, incorporating AI features into .NET Core projects can have a significant impact on both performance and functionality. With the right resources and tools, developers can easily harness the benefits of AI in .NET Core development.
AI Frameworks for .NET Core
When integrating AI capabilities into .NET Core applications, developers have access to a wide range of AI frameworks to choose from. Each framework has unique features and capabilities, making it important to understand which one is best suited for a specific project or application.
TensorFlow
TensorFlow is an open-source machine learning framework created by Google. It is designed for building and training deep neural networks. TensorFlow provides APIs for several programming languages, including Python, C++, and, of course, .NET Core. TensorFlow for .NET Core, also known as TensorFlow.NET, is a wrapper library that allows .NET developers to use TensorFlow’s APIs in their .NET Core applications.
Pros | Cons |
---|---|
|
|
Here is an example of how to use TensorFlow.NET to train a simple image classification model:
var model = new Sequential(); model.Add(new Dense(64, activation: "relu", inputShape:
new Shape(784))); model.Add(new Dense(10, activation: "softmax"));
model.Compile(optimizer: "rmsprop", loss: "categorical_crossentropy", metrics: new[]
{ "accuracy" }); model.Fit(xTrain, yTrain, batch_size: 128, epochs:
20, validation_data: new Tuple(xTest, yTest));
ML.NET
ML.NET is a free, open-source, and cross-platform machine learning framework created by Microsoft. It is specifically designed for .NET developers to build custom machine learning models using C# or F# without requiring expertise in machine learning. ML.NET supports a range of scenarios, including binary classification, regression, and anomaly detection.
Pros | Cons |
---|---|
|
|
Here is an example of how to use ML.NET to build and train a linear regression model:
var pipeline = new LearningPipeline();
pipeline.Add(new TextLoader(dataPath).CreateFrom());
pipeline.Add(new ColumnCopier(("Salary", "Label")));
pipeline.Add(new CategoricalOneHotVectorizer("Gender"));
pipeline.Add(new ColumnConcatenator("Features", "YearsExperience", "Gender",
"Industry")); pipeline.Add(new LinearRegression()); var model = pipeline.Train();
var prediction = model.Predict(new SalaryData { YearsExperience = 8.3f, Gender =
"Male", Industry = "Engineering" });
As you can see, both TensorFlow and ML.NET have their own strengths and weaknesses, making it important to understand the specific needs of your project before choosing an AI framework. By choosing the right framework, you can ensure that your .NET Core application is equipped with the necessary AI capabilities to drive value for your end-users.
AI Algorithms and Models in .NET Core
AI algorithms and models are the backbone of AI systems, providing the intelligence needed to perform complex tasks. In .NET Core, developers can leverage a wide range of AI algorithms and models to power their applications.
One commonly used AI algorithm is the k-nearest neighbors (KNN) algorithm, which is often used in data mining and pattern recognition applications. With .NET Core, developers can easily implement KNN with the Accord.NET framework, which provides a rich set of data analysis and machine learning features.
The following code snippet demonstrates how to use KNN in .NET Core with Accord.NET:
// Load the dataset
var iris = new Iris();
var inputs = iris.Instances
.Select(i => i.Input)
.ToArray();
// Create the KNN algorithm
var knn = new KNearestNeighbors(k: 5);
// Train the algorithm
knn.Learn(inputs);
// Use the algorithm to predict
var prediction = knn.Decide(inputs[0]);
Another powerful AI model is the artificial neural network (ANN), which can be used for tasks such as image recognition and natural language processing. The ML.NET framework provides a neural network implementation that can be used within .NET Core applications.
The following code snippet demonstrates how to use the neural network model in ML.NET:
// Load the dataset
var data = new List();
// ...
// Build the pipeline
var pipeline = mlContext.Transforms.Conversion
.MapValueToKey(outputColumnName: "Label")
.Append(mlContext.Transforms.LoadRawImageBytes(
outputColumnName: "Image",
imageFolder: "images",
inputColumnName: nameof(ImageData.ImagePath)))
.Append(mlContext.Transforms.ResizeImages(
outputColumnName: "Image",
imageWidth: 224,
imageHeight: 224,
inputColumnName: "Image"))
.Append(mlContext.Transforms.ExtractPixels(
outputColumnName: "Image",
colorsToExtract: ImagePixelExtractingEstimator.Colors.Red,
offsetImage: 117))
.Append(mlContext.Model.LoadTensorFlowModel(
modelLocation: "model.pb",
inputColumnNames: new[] { "input" },
outputColumnNames: new[] { "output" }))
.Append(mlContext.Transforms.Conversion.MapKeyToValue(
outputColumnName: "PredictedLabel",
inputColumnName: "Prediction"));
// Train the model
var model = pipeline.Fit(dataView);
// Use the model to predict
var prediction = model.CreatePredictionEngine(mlContext)
.Predict(new ImageData { ImagePath = "test.jpg" });
These are just a few examples of the AI algorithms and models that can be used with .NET Core. By leveraging these powerful tools, developers can create intelligent applications that can analyze data, recognize patterns, and make predictions with ease.
AI-Powered Applications with .NET Core
AI-powered applications are becoming increasingly prevalent across industries as businesses look to leverage the power of machine learning and natural language processing to automate processes, enhance customer experiences, and gain valuable insights from data. With .NET Core, developers have a powerful platform for building and deploying AI-powered applications with ease and simplicity.
One example of an AI-powered application built with .NET Core is a chatbot. Chatbots can be used for a variety of purposes, such as customer service, lead generation, and product recommendations. By integrating natural language processing and machine learning algorithms, a chatbot built with .NET Core can interpret user input and provide personalized responses in real-time.
Another example of an AI-powered application is a recommendation engine. By analyzing user behavior and preferences, recommendation engines can suggest products, services, or content that are most relevant to individual users. With .NET Core, developers can utilize neural networks and other AI algorithms to build powerful recommendation engines that enhance the overall user experience.
AI-powered applications can also be used for predictive analytics, fraud detection, and data visualization. By leveraging the capabilities of .NET Core and AI technologies, developers can create applications that can process large amounts of data quickly and accurately, providing actionable insights for businesses.
Example: Building a Chatbot with .NET Core
Building a chatbot with .NET Core is relatively simple, thanks to the availability of powerful AI frameworks such as Microsoft Bot Framework and IBM Watson. Here is an example of how to build a basic chatbot using the Microsoft Bot Framework:
Step | Code |
---|---|
1. Create a new bot project | dotnet new bot |
2. Add the Microsoft Bot Builder SDK package | dotnet add package Microsoft.Bot.Builder |
3. Implement the bot logic in C# | public async Task OnTurnAsync(ITurnContext turnContext, CancellationToken cancellationToken) { // Your bot logic goes here } |
4. Train the bot using Microsoft LUIS | Follow the instructions provided by the Microsoft Bot Framework documentation for integrating with LUIS. |
With just a few lines of code and the integration of LUIS, a chatbot can be up and running in no time.
Example: Building a Recommendation Engine with .NET Core
Building a recommendation engine with .NET Core involves utilizing AI algorithms such as collaborative filtering and content-based filtering. Here is an example of how to build a basic recommendation engine using the ML.NET library:
Step | Code |
---|---|
1. Define the data model | public class ProductEntry { public float Label; public float UserID; public float ProductID; public float Rating; } |
2. Load the data from a CSV file | var data = mlContext.Data.LoadFromTextFile(dataPath, separator: ‘,’); |
3. Split the data into training and testing sets | var trainTestSplit = mlContext.Data.TrainTestSplit(data, testFraction: 0.2); |
4. Define the pipeline for the recommendation engine | var pipeline = mlContext.Transforms.Conversion.MapValueToKey(outputColumnName: “UserKey”, inputColumnName: “UserID”) .Append(mlContext.Transforms.Conversion.MapValueToKey(outputColumnName: “ProductKey”, inputColumnName: “ProductID”)) .Append(mlContext.Recommendation().Trainers.MatrixFactorization(“UserKey”, “ProductKey”, labelColumnName: “Label”, numberOfIterations: 20)); |
5. Train the recommendation engine | var model = pipeline.Fit(trainTestSplit.TrainSet); |
6. Evaluate the model | var metrics = mlContext.Regression.Evaluate(model.Transform(trainTestSplit.TestSet)); |
With the ML.NET library, building a recommendation engine with .NET Core is made simpler and more efficient.
AI-powered applications are just one example of how the capabilities of .NET Core and AI technologies can be combined to create powerful and innovative solutions. With the flexibility and versatility of .NET Core, developers have endless possibilities for incorporating AI-driven enhancements into their own applications.
Best Practices for AI Integration in .NET Core
Integrating AI capabilities into .NET Core applications can greatly enhance their functionality and performance. However, there are several best practices that should be followed to ensure a seamless integration.
Here are some tips and insights for effective AI integration in .NET Core:
- Understand the problem you are trying to solve: Before incorporating AI technologies into your .NET Core project, it is important to have a clear understanding of the problem you are trying to solve. This will help determine the most appropriate AI algorithms and models to use and ensure that the integration is effective and efficient.
- Preprocess your data: High-quality data is essential for effective AI integration in .NET Core. Therefore, it is important to preprocess your data to ensure that it is accurate, relevant, and properly formatted. This will help improve the accuracy and reliability of your AI-driven enhancements.
- Choose the right AI framework: There are several AI frameworks that are compatible with .NET Core, each with their own unique features and capabilities. When choosing an AI framework, it is important to consider factors such as the complexity of your project, the size of your data, and the accuracy requirements of your application.
- Train and evaluate your model: Once you have chosen an AI algorithm and model, it is important to train and evaluate it to ensure that it is accurate and effective. This involves dividing your data into training and testing sets and using the training set to train your model while using the testing set to evaluate its performance.
- Consider deployment considerations: Finally, it is important to consider deployment considerations when integrating AI technologies into your .NET Core project. This includes factors such as scalability, security, and performance. Make sure that your deployment environment is optimized for AI-driven enhancements, and that you have the necessary infrastructure in place to support them.
By following these best practices, you can ensure a smooth and efficient integration of AI technologies into your .NET Core project.
Conclusion
Incorporating AI capabilities into .NET Core projects has become increasingly important in today’s technology landscape. As we have explored in this article, the benefits of integrating AI technologies with .NET Core applications can be significant, including enhanced performance, increased precision, and improved user experience.
External Resources
https://dotnet.microsoft.com/en-us/
https://dotnet.microsoft.com/en-us/apps/machinelearning-ai/ml-dotnet
FAQ
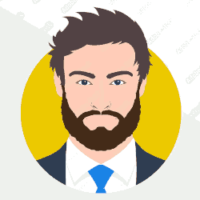
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.