“Autofac – the secret ingredient to mastering .NET Core!” Does this statement pique your curiosity? Let’s explore the fascinating world of Autofac in .NET Core together.
This post will enlighten you on the relevance of Autofac, diverse opinions on its usage, strategic insights, and some exciting learning resources.
The Backstory: Understanding .NET Core and Autofac
To appreciate Autofac, we must first journey back to the beginnings of .NET Core. Introduced by Microsoft, .NET Core is a cross-platform, open-source framework for building modern, cloud-based, internet-connected applications.
It’s like the Lego blocks you played with as a kid – but instead of building toys, you’re creating software.
Autofac, on the other hand, is a popular Inversion of Control (IoC) container. Like the magic sorting hat from Harry Potter, it helps manage and organize the different components of your software efficiently. Using Autofac with .NET Core enhances your software’s performance, scalability, and maintainability.
Key Takeaways:
- Autofac as a Powerful Choice: Autofac in .NET Core provides advanced control and flexibility in managing dependencies, making it an ideal choice for complex applications.
- Understanding Registration: Different service types can be registered in Autofac, including open generic types and named or keyed services, providing significant flexibility.
- Integrations and Compatibility: Autofac smoothly integrates with ASP.NET Core Identity and Entity Framework Core, making it a robust choice for modern web applications.
- Problem-solving Techniques: Common issues faced when using Autofac, such as trouble with multiple implementations or property injection, can be effectively managed with the right strategies.
- Testing and Resolution: Autofac encourages the use of integration tests to ensure correct dependency registration and prevent runtime errors.
- Resources and Learning: A wealth of resources exists to learn more about Autofac, from books to online tutorials on platforms like Pluralsight, Udemy, and YouTube.
Why Use Autofac in .NET Core
Here’s why Autofac has become a darling among .NET Core developers:
- Flexibility: Autofac’s flexible architecture lets you decide how components interact and manage their lifetimes.
- Advanced features: Autofac offers features not available in the default .NET Core IoC container, such as property and method injection.
- Integration: Autofac integrates seamlessly with a range of frameworks and libraries used in .NET Core development.
Let’s dig deeper into the reasons why Autofac can be a valuable addition to your .NET Core development toolbox.
The .NET Core’s built-in IoC container serves the needs of many applications quite adequately. However, when your software’s needs grow in complexity, Autofac‘s extended features make it an enticing choice.
1. Flexible Configuration
Autofac provides multiple ways to configure the IoC container. You can either use the code-based registration, where you manually register each type, or the module-based registration, where types are grouped into modules and registered together.
Here’s an example of how you might register types manually in Autofac:
var builder = new ContainerBuilder();
builder.RegisterType<MyComponent>().As<IMyService>();
var container = builder.Build();
The equivalent configuration in module registration might look like this:
public class MyModule : Module
{
protected override void Load(ContainerBuilder builder)
{
builder.RegisterType<MyComponent>().As<IMyService>();
}
}
With these configurations, you’ve instructed Autofac to create an instance of MyComponent
anytime IMyService
is required.
2. Support for Advanced Scenarios
Autofac supports a variety of advanced scenarios that aren’t possible with the .NET Core’s built-in container.
For instance, Autofac provides property injection, which can be handy when you need to inject services into properties, such as in MVC controllers.
Here’s an example:
public class MyController : Controller { public IMyService MyService { get; set; } }
In this code snippet, Autofac will automatically inject an instance of IMyService
into the MyService
property when it creates MyController
.
Another powerful feature is method injection, which allows you to inject services directly into methods.
Here’s how you can do it:
public class MyComponent { public void Configure(IMyService service) { // Use 'service' here. } }
In this case, when Autofac creates MyComponent
, it will automatically call the Configure
method with an appropriate IMyService
instance.
3. Integration with Other Frameworks
Autofac provides seamless integration with a variety of frameworks used in .NET Core development. Whether you’re building MVC applications, working with Entity Framework, or creating SignalR hubs, Autofac has got you covered.
Take a look at this example of how Autofac integrates with ASP.NET Core MVC:
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
// Add Autofac
var containerBuilder = new ContainerBuilder();
containerBuilder.RegisterModule<MyModule>();
containerBuilder.Populate(services);
this.ApplicationContainer = containerBuilder.Build();
return new AutofacServiceProvider(this.ApplicationContainer);
}
In this example, Autofac is set up to work in harmony with ASP.NET Core‘s built-in IoC container. By calling Populate
, you’re telling Autofac to copy registrations from the built-in container, allowing both to coexist.
Autofac Hacks
Having worked with Autofac and .NET Core for quite a while, here’s a little hack that can save you loads of time: use Autofac’s assembly scanning feature.
Instead of manually registering every type in your assembly, you can scan the assembly and register types that meet certain conditions:
var dataAccess = Assembly.GetExecutingAssembly(); builder.RegisterAssemblyTypes(dataAccess) .Where(t => t.Name.EndsWith("Repository")) .AsImplementedInterfaces();
In this code snippet, Autofac is scanning the current assembly and registering all types whose names end with “Repository” as their implemented interfaces. This can be a massive time saver as your project grows!
Autofac is an excellent tool for .NET Core developers who need more flexibility and control over their IoC container. Whether you’re building a simple application or a complex system, Autofac has the features and integrations to make your life easier.
The Great Debate: To Autofac or Not to Autofac
Despite its many benefits, Autofac’s usage in .NET Core remains a topic of healthy debate. One school of thought prefers sticking to the built-in .NET Core IoC container for its simplicity and adequacy for most scenarios.
On the other hand, proponents of Autofac vouch for its advanced capabilities, making it indispensable for complex applications. They view Autofac as a powerful enhancement to .NET Core’s built-in features.
Like choosing between Superman and Batman, the decision to use Autofac often boils down to personal preference, project requirements, and complexity.
Let’s dive further into the ongoing debate: “To Autofac or Not to Autofac?”
As a .NET developer, I’ve seen various perspectives about this decision. The choice often hinges on several factors like project complexity, team experience, and specific application requirements.
The Built-In IoC Container Camp
The default Inversion of Control (IoC) container in .NET Core is more than sufficient for many developers and applications. It supports the fundamental dependency injection (DI) patterns and is straightforward to use.
Developers often favor this option for its simplicity and tight integration with the .NET Core ecosystem.
Here’s a basic sample of .NET Core’s built-in IoC container:
public void ConfigureServices(IServiceCollection services) { services.AddTransient<IMyService, MyService>(); services.AddControllers(); }
In this snippet, the IMyService
interface is registered with its corresponding MyService
implementation. Each time IMyService
is requested, a new MyService
instance is created, thanks to AddTransient
.
The Autofac Camp
However, proponents of Autofac argue that the .NET Core’s built-in IoC container lacks the advanced features that Autofac provides, especially for larger, more complex applications.
Let’s revisit the method injection feature of Autofac, not available in .NET Core’s built-in container:
public class MyComponent { public void SetService(IMyService service) { // Use 'service' here. } }
In this example, Autofac will call the SetService
method with an appropriate IMyService
instance when MyComponent
is created.
Finding The Middle Ground
There are also developers who, like Switzerland, prefer neutrality in this debate. They maintain that both .NET Core’s built-in container and Autofac have their place in the .NET ecosystem. The choice should be dictated by the project’s needs and not based on subjective opinions.
For example, a simple CRUD application might not need the extra features that Autofac provides. In this scenario, .NET Core’s built-in IoC container would suffice.
However, for complex applications with intricate dependencies or those needing property or method injection, Autofac might be the better choice.
Here’s a nifty trick for those who want the best of both worlds: you can use Autofac alongside .NET Core’s built-in container!
public IServiceProvider ConfigureServices(IServiceCollection services) { services.AddControllers(); var builder = new ContainerBuilder(); builder.Populate(services); builder.RegisterType<MyService>().As<IMyService>().InstancePerLifetimeScope(); var container = builder.Build(); return new AutofacServiceProvider(container); }
In this example, we use the Populate
method to add the registrations from .NET Core’s built-in container to Autofac. This way, you can use both the built-in services and Autofac’s advanced features.
The “To Autofac or Not to Autofac” debate ultimately comes down to understanding your project’s needs, your team’s familiarity with the tool, and the specific requirements of the application.
Both Autofac and .NET Core’s built-in IoC container have their strengths, and knowing when to use each can make you a more effective .NET developer.
Autofac Strategies and Tactics
The best way to win any battle, including navigating the world of .NET Core and Autofac, is to have a well-planned strategy. Here are a few:
- Start simple: Begin with the basic features before diving into advanced functionalities.
- Follow best practices: Always adhere to Autofac and .NET Core’s recommended best practices to avoid common pitfalls.
- Stay updated: Keep up with the latest developments in Autofac and .NET Core to take advantage of new features and improvements.
Learning Resources: Books and YouTube Channels
Ready to dive deeper into Autofac? Here are a few resources to explore:
- Books: Check out “”Pro ASP.NET Core 3: Develop Cloud-Ready Web Applications Using MVC, Blazor, and Razor Pages” by Adam Freeman and “Dependency Injection in .NET” by Mark Seemann.
- YouTube Channels: For visual learners, channels like “IAmTimCorey”, and “Gunnar Peipman’s Programming Blog” offer comprehensive tutorials on Autofac.
Remember, the best learning strategy involves a balance of reading, watching, and doing. Read the books, watch the tutorials, then get your hands dirty by practicing with real code!
5 Common problems developers face when using Autofac with .NET Core and their potential solutions:
1. Trouble with Registering Multiple Implementations
Problem: A common issue occurs when registering multiple implementations of the same interface. The .NET Core built-in IoC container resolves the last registered implementation by default.
builder.RegisterType<MyService1>().As<IMyService>(); builder.RegisterType<MyService2>().As<IMyService>();
Solution: To solve this issue, Autofac’s Resolve<IEnumerable<T>>
method can be used to obtain all registered services of a particular interface:
var myServices = container.Resolve<IEnumerable<IMyService>>();
2. Lifetimes and Scope Issues
Problem: A common pitfall is the incorrect configuration of component lifetimes and misunderstanding the scoping mechanism in Autofac.
Solution: Ensure you use the right lifetime manager (InstancePerDependency
, SingleInstance
, InstancePerLifetimeScope
) according to your needs.
For example, SingleInstance
creates a singleton, while InstancePerLifetimeScope
creates a new instance per request in ASP.NET Core.
3. Lack of Autofac Intellisense
Problem: Developers often bemoan the lack of Intellisense when configuring the IoC container.
Solution: Unfortunately, this issue isn’t easily resolved as it’s inherent to how reflection-based DI containers work. However, it’s often helpful to use a consistent naming convention for your modules and group them logically to avoid confusion and errors.
4. Trouble with Property Injection
Problem: Some developers struggle with property injection, especially when trying to implement it in controllers.
Solution: By default, Autofac doesn’t inject properties. However, it can be configured to do so using the PropertiesAutowired
method:
builder.RegisterType<MyController>().PropertiesAutowired();
With this configuration, Autofac will fill all the writable properties of MyController
that it can resolve.
5. Dependency Resolution Exceptions
Problem: A common issue developers face is dependency resolution exceptions at runtime, often due to missing or incorrect registrations.
Solution: These exceptions can be mitigated by writing integration tests to ensure all required dependencies can be resolved. Autofac’s ContainerBuilder
can be utilized to mimic the application’s startup process, thus ensuring that all dependencies are correctly registered.
var builder = new ContainerBuilder();
// Register all your dependencies here
// ...
var container = builder.Build();
// The following line will throw an exception if any dependency can't be resolved
container.Resolve<MyController>();
By addressing these common problems, developers can utilize Autofac in .NET Core more effectively. It’s worth noting that while these issues may pose initial challenges, the enhanced control and flexibility that Autofac offers can be worth it for complex applications.
7 FAQs about using Autofac in .NET Core
1. How do I register open generic types in Autofac?
Open generic types are often used with repositories or similar patterns.
Here’s how to register an open generic type:
builder.RegisterGeneric(typeof(MyRepository<>)).As(typeof(IMyRepository<>));
This code will make MyRepository<T>
available whenever IMyRepository<T>
is requested, for any T
.
2. How do I use Autofac with ASP.NET Core Identity?
To use Autofac with ASP.NET Core Identity, first, add the required services to the IServiceCollection:
services.AddIdentity<ApplicationUser, IdentityRole>() .AddEntityFrameworkStores<ApplicationDbContext>() .AddDefaultTokenProviders();
Then, when configuring Autofac, populate the services from the IServiceCollection:
var builder = new ContainerBuilder(); builder. Populate(services);
3. How do I configure Autofac to resolve a specific instance?
To resolve a specific instance of a class, use the RegisterInstance
method:
var myService = new MyService();
builder.RegisterInstance(myService).As<IMyService>();
4. How do I register a class that requires parameters in the constructor?
To register a class that has constructor parameters, use the Register
method with a lambda:
builder.Register(c => new MyService("parameter")).As<IMyService>();
5. How can I resolve services dynamically at runtime?
You can use the IComponentContext
interface to resolve services dynamically at runtime:
public class MyService : IMyService { private readonly IComponentContext _context; public MyService(IComponentContext context) { _context = context; } public void DoSomething() { var myOtherService = _context.Resolve<IMyOtherService>(); myOtherService.Execute(); } }
6. How can I use named or keyed services with Autofac?
To register named or keyed services, use the Named
or Keyed
methods:
builder.RegisterType<MyService>().Named<IMyService>("service1"); builder.RegisterType<MyOtherService>().Named<IMyService>("service2");
To resolve a named or keyed service, use the ResolveNamed
or ResolveKeyed
methods:
var myService = container.ResolveNamed<IMyService>("service1");
7. How do I integrate Autofac with Entity Framework Core?
To integrate Autofac with EF Core, register the DbContext using the RegisterType
method:
builder.RegisterType<MyDbContext>() .WithParameter("options", new DbContextOptionsBuilder() .UseSqlServer(connectionString) .Options) .InstancePerLifetimeScope();
In the above example, MyDbContext
will be created per HTTP request in an ASP.NET Core application, ensuring the same DbContext is used throughout the entire request lifecycle.
Wrapping up: Embracing Autofac in .NET Core
In a nutshell, using Autofac in .NET Core is like upgrading your Lego set – you get more pieces to play with, and thus, can build bigger, more complex structures. Still, the decision to use Autofac should be based on your project’s needs and your comfort level with the tool.
Do you have personal experiences with Autofac in .NET Core? Or maybe a favorite resource you’d like to share? Drop your thoughts in the comment section below. And don’t forget to share this post with your fellow .NET Core enthusiasts.
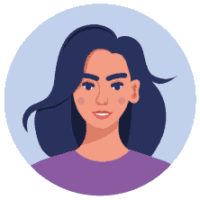
Jessica is a highly accomplished technical author specializing in scientific computer science. With an illustrious career as a developer and program manager at Accenture and Boston Consulting Group, she has made significant contributions to the successful execution of complex projects.
Jessica’s academic journey led her to CalTech University, where she pursued a degree in Computer Science. At CalTech, she acquired a solid foundation in computer systems, algorithms, and software engineering principles. This rigorous education has equipped her with the analytical prowess to tackle complex challenges and the creative mindset to drive innovation.
As a technical author, Jessica remains committed to staying at the forefront of technological advancements and contributing to the scientific computer science community. Her expertise in .NET C# development, coupled with her experience as a developer and program manager, positions her as a trusted resource for those seeking guidance and best practices. With each publication, Jessica strives to empower readers, spark innovation, and drive the progress of scientific computer science.