As the demands of software development continue to grow and evolve, developers and organizations are constantly looking for ways to increase productivity without sacrificing quality. One framework that has gained traction in recent years is .NET Core, an open-source, cross-platform framework that enables developers to build high-quality applications efficiently.
But, is .NET Core productive for software development? In this article, we will explore the various features and capabilities of .NET Core to assess its productivity potential. We will delve into the features that enhance developer efficiency, measure coding productivity, and compare .NET Core’s performance with other popular frameworks like Java and Python. Additionally, we will provide real-world case studies, practical tips, and industry trends to help developers leverage the full potential of .NET Core and enhance their productivity.
Join us as we take a deep dive into assessing the productivity of .NET Core development and exploring the features that enhance developer efficiency in .NET Core projects. Let’s get started!
Features that Enhance Developer Efficiency in .NET Core
When it comes to developing software, productivity is of utmost importance. And .NET Core, Microsoft’s free, open-source platform for building all sorts of applications, is no exception. In fact, it is specifically designed to enhance developer efficiency in several ways.
Cross-Platform Compatibility
One of the significant advantages of .NET Core is its ability to run on multiple platforms, including Windows, Linux, and macOS. This cross-platform compatibility allows developers to work on the same codebase across different environments and eliminates the need for creating separate versions of an application, ultimately improving productivity.
Dependency Injection
Dependency Injection, a design pattern that enables developers to write loosely coupled code, is baked into the .NET Core framework. This feature simplifies testing, promotes code reuse, and makes it easier to replace components when necessary.
Use of Modern Programming Languages
.NET Core supports and integrates with several modern programming languages, including C#, F#, and Visual Basic. These languages offer features such as functional programming and pattern matching, providing developers with more tools to improve their productivity.
Other features, such as the in-built command-line interface, the ability to create microservices, and the easy integration with cloud services, all contribute to making .NET Core a highly productive framework for software development.
Performance Comparison: .NET Core vs. Other Frameworks
When it comes to evaluating productivity in .NET Core, it’s important to compare it with other popular frameworks in the market. Let’s take a look at how .NET Core stacks up against two other widely-used frameworks – Java and Python.
Execution Speed
One of the key factors to evaluate when comparing the productivity of different frameworks is their execution speed. While Java is known for its high speed, .NET Core is not far behind. Research conducted by TechEmpower has shown that .NET Core is about 3-6 times faster than Python, depending on the benchmark. However, Java still leads the pack with up to 29 times higher throughput than .NET Core for certain workloads.
Memory Usage
Memory usage is another important factor to consider when comparing productivity. While Java and Python have a reputation for being memory-intensive, .NET Core is among the best in class for low memory usage. According to TechEmpower benchmarks, .NET Core uses less memory than both Java and Python, making it an ideal choice for resource-constrained environments.
Scalability
Scalability is yet another key parameter that impacts the productivity of software development teams. .NET Core excels in this regard with its support for horizontal and vertical scaling. It offers built-in support for containerization and cloud deployment, making it easy to scale up or down based on project requirements. Java is also known for its scalability, while Python lags behind in this aspect.
Code Examples
Framework | Code Example |
---|---|
.NET Core | var result = await httpClient.GetAsync(“https://jsonplaceholder.typicode.com/posts”); |
Java | HttpResponse response = httpClient.execute(httpGet); |
Python | response = requests.get(‘https://jsonplaceholder.typicode.com/posts’) |
When it comes to code examples, .NET Core offers developers the ability to use modern programming languages such as C# and F#. The example above shows the simplicity and readability of asynchronous code using the await keyword in .NET Core. In comparison, Java requires more verbose code to achieve the same result, while Python uses a different library altogether.
Overall, while .NET Core may not always be the fastest framework out there, it excels in other areas such as low memory usage and scalability. With a growing community and support for modern programming languages, .NET Core is definitely a productivity-enhancing tool for software development teams.
Coding Productivity in .NET Core Projects
Coding productivity in .NET Core projects can be measured using different tools, libraries, and frameworks that enhance developer efficiency. These tools allow developers to write clean and maintainable code, resulting in faster development and fewer bugs. Here are some of the ways developers can improve their coding productivity in .NET Core projects:
1. Use Extensions
Extensions are a powerful feature in .NET Core that allow developers to extend the functionality of built-in classes. They can help simplify code and reduce repetition. For example, consider the following code:
//Without Extension
var result = Enumerable.Range(1, 5)
.Where(i => i % 2 == 0)
.ToList();
With extension:
//With Extension
var result = Enumerable.Range(1, 5)
.EvenNumbers()
.ToList();public static class EnumerableExtensions
{
public static IEnumerable<int> EvenNumbers(this IEnumerable<int> numbers)
{
return numbers.Where(n => n % 2 == 0);
}
}
The second example uses an extension method to simplify the code and make it more readable.
2. Use LINQ Queries
Language-Integrated Query (LINQ) provides a powerful syntax for querying data within .NET Core projects. It allows queries to be written in a way that is similar to SQL queries. Here’s an example:
var names = new List<string> { “Adam”, “Bob”, “Charles” };
var result = from name in names
where name.StartsWith(“A”)
select name;
In this example, we’re selecting names that start with the letter “A”. LINQ queries can be written in a single line or a more readable multi-line format.
3. Use Third-Party Libraries
There are many third-party libraries available that can help increase coding productivity in .NET Core projects. Some popular libraries include AutoMapper for object mapping, Newtonsoft.Json for JSON serialization and deserialization, and Serilog for logging.
4. Write Unit Tests
Unit tests help ensure that code is correct and maintainable. They can catch bugs early in the development process, making it easier to fix them before the code is deployed. Tools such as xUnit and NUnit can be used for unit testing in .NET Core projects.
By using these techniques and tools, developers can significantly increase their productivity when coding in .NET Core projects.
Increasing .NET Core Productivity with Code Examples
Developers can greatly enhance their productivity in .NET Core projects by implementing various programming concepts and using the framework’s unique features. Here are some code examples to illustrate these productivity-enhancing techniques:
Asynchronous Programming
One of the most significant improvements in .NET Core is the ability to write asynchronous code. This allows developers to run multiple tasks simultaneously, resulting in faster and more efficient processing. Here’s an example of asynchronous programming in .NET Core:
// Define an asynchronous method
async Task<string> DownloadFileAsync(string url)
{
<…>
}
In this example, the “DownloadFileAsync” method is defined as asynchronous by using the “async” keyword. This allows it to run concurrently with other tasks. As a result, the method can download a file from a URL without blocking the main thread, improving the performance of the entire application.
LINQ Queries
The Language-Integrated Query (LINQ) is a powerful productivity-enhancing feature in .NET Core that enables developers to write SQL-like queries in C#. Here’s an example of a LINQ query:
// Define a LINQ query
var customers = from c in context.Customers
where c.City == “Seattle”
select c;
This code will retrieve all customers from the “Customers” table in the database that have a “City” value of “Seattle”. The query is written in a SQL-like syntax that is easy to read and understand.
Leveraging Third-Party Libraries
.NET Core has a vast selection of third-party libraries that can greatly enhance developer productivity. One such library is the AutoMapper, which allows developers to map one object to another without writing repetitive code. Here’s an example:
// Install the AutoMapper library
Install-Package AutoMapper
// Define a mapping between two objects
var config = new MapperConfiguration(cfg => cfg.CreateMap<SourceObject, DestinationObject>());
In this example, the AutoMapper library is installed using the NuGet Package Manager. Then, a mapping is defined between a “SourceObject” and a “DestinationObject”. Once the mapping is defined, developers can easily map one object to another without writing a lot of repetitive code. This saves time and effort and improves productivity.
Industry Trends and Research on .NET Core Productivity
As .NET Core continues to gain popularity, industry experts have been closely monitoring trends and conducting research to evaluate the productivity potential of the framework.
According to recent reports, the adoption of .NET Core has been steadily increasing in industries such as finance, healthcare, and e-commerce. Developers cite the framework’s flexibility, cross-platform capabilities, and performance as key factors contributing to its productivity.
Research has also shown that .NET Core projects tend to have higher development speed and lower maintenance costs compared to traditional .NET Framework projects. This is mainly due to .NET Core’s modular design, which allows for faster compilation times and more efficient memory usage.
Another area of research is focused on the impact of the .NET Core on developer productivity. Studies show that developers who are well-versed in .NET Core technologies tend to produce higher quality code with fewer bugs and faster turnaround times. Additionally, the availability of third-party libraries and tools has further enhanced productivity in .NET Core development.
Looking ahead, industry experts predict that the productivity potential of .NET Core will only continue to grow, with advancements in areas such as machine learning, cloud computing, and IoT driving demand for the framework.
Tips for Improving Productivity in .NET Core Development
Developing software in .NET Core can be a smooth process with a little organization and the right tools. Here are some tips to help you boost productivity:
1. Use LINQ
LINQ (Language Integrated Query) is a powerful feature that allows you to query data from multiple sources in a single line of code. This saves time and makes your code more readable. For example, instead of writing a loop to iterate through a list of objects, you can use LINQ to filter and sort the list:
var sortedList = myList.Where(x => x.Age > 18).OrderByDescending(x => x.LastName);
2. Leverage Asynchronous Programming
Asynchronous programming in .NET Core allows you to perform multiple tasks simultaneously, improving overall performance. It’s especially useful for long-running operations like I/O-bound tasks. For instance, you can use the async and await keywords to make a web service call without blocking the main thread:
var result = await httpClient.GetAsync(“http://example.com”);
3. Use a Debugger
The Visual Studio debugger is a powerful tool for finding and fixing bugs in your code. It allows you to step through your code line-by-line, set breakpoints, and inspect variables. You can also use conditional breakpoints to pause your code when a specific condition is met.
4. Adopt Clean Code Practices
Writing clean and maintainable code is crucial for productivity in the long run. Best practices include naming variables and functions descriptively, breaking down large functions into smaller ones, and using comments to document your code. For example:
// Calculate the sum of two numbers
int sum(int a, int b)
{
return a + b;
}
5. Use Productivity-Enhancing Tools
There are numerous tools and extensions available for .NET Core development that can improve productivity. For example, ReSharper is a popular productivity tool that provides code analysis, refactoring, and code completion features. Visual Studio also offers a wide range of extensions and add-ons to help you automate repetitive tasks.
By adopting these tips and practices, you can increase your productivity and efficiency when developing in .NET Core.
Conclusion: The Productivity Potential of .NET Core
In conclusion, evaluating productivity in .NET Core has revealed that this framework offers a range of features that can enhance developer efficiency and coding productivity. From cross-platform compatibility to dependency injection and the ability to use modern programming languages, .NET Core offers a competitive advantage for software development teams.
Comparing .NET Core productivity with other frameworks such as Java and Python has shown that this framework can provide faster execution speed, lower memory usage, and greater scalability. Additionally, the many tools, libraries, and frameworks available in .NET Core can significantly improve coding efficiency.
Numerous industry examples and case studies have highlighted the successful use of .NET Core for productive software development. These success stories illustrate the unique features and approaches adopted by organizations and companies to achieve high productivity levels.
By providing practical tips and strategies for improving productivity in .NET Core development, this article has demonstrated how developers can take full advantage of the framework’s features. Code examples, such as asynchronous programming and LINQ queries, have illustrated programming concepts or features of frameworks.
Research related to .NET Core productivity and latest industry trends have provided insights into the future of .NET Core development. Overall, .NET Core offers considerable potential for software development teams to achieve greater productivity in their projects.
In conclusion, it is evident that .NET Core is a productive framework that can enhance coding efficiency and developer productivity. With the continual development of new features and tools, the future of .NET Core looks bright and promising for software development teams.
FAQ
Q: Is .NET Core productive for software development?
A: .NET Core offers numerous features and tools that enhance developer efficiency and productivity. Its cross-platform compatibility, dependency injection, and support for modern programming languages contribute to a productive development experience.
Q: What features in .NET Core enhance developer efficiency?
A: .NET Core incorporates various features that improve developer efficiency, including cross-platform compatibility, dependency injection, and the ability to use modern programming languages.
Q: How does .NET Core productivity compare to other frameworks?
A: When comparing the performance and productivity of .NET Core to other frameworks like Java and Python, factors such as execution speed, memory usage, and scalability are analyzed to assess productivity.
Q: How can coding productivity in .NET Core projects be measured?
A: Coding productivity in .NET Core projects can be measured by evaluating the use of tools, libraries, and frameworks that enhance coding efficiency, as well as following best practices for clean and maintainable code.
Q: Are there any examples of organizations achieving high productivity with .NET Core?
A: Yes, there are numerous case studies of organizations that have achieved high productivity using .NET Core. These examples showcase the success stories and highlight the unique approaches adopted by these organizations.
Q: Can you provide examples of code that enhance productivity in .NET Core?
A: Certainly! Code examples in .NET Core can demonstrate how developers can improve productivity. Examples may include asynchronous programming, LINQ queries, and utilizing third-party libraries.
Q: What are the latest industry trends and research on .NET Core productivity?
A: The latest industry trends and research on .NET Core productivity shed light on the topic and provide insights into the future of .NET Core development. These studies, surveys, or reports offer valuable information for software development teams.
Q: Are there any tips for improving productivity in .NET Core development?
A: Yes, there are several practical tips and strategies for developers to enhance their productivity in .NET Core projects. These may include code organization, debugging techniques, and leveraging productivity-enhancing tools.
Q: What are the concluding thoughts on the productivity potential of .NET Core?
A: In conclusion, .NET Core has a significant productivity potential due to its features, tools, and community support. Future developments in the framework will continue to enhance productivity for software development teams.
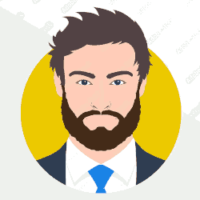
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.