Are you hesitant to dip your toes into the waters of .NET Core Kubernetes because of the skepticism surrounding it? Fear no more.
Let’s bust the myths and set the stage for best practices in .NET Core Kubernetes.
Key Takeaways:
- Embrace Automation: From managing configurations to deploying applications, automating routine tasks is key in Kubernetes. Tools like Helm can significantly simplify your .NET Core Kubernetes journey.
- Data Persistence Matters: In the ephemeral world of Kubernetes, ensure data longevity using Persistent Volumes (PV) and Persistent Volume Claims (PVC). They help create a persistent disk that lives independently from your containers.
- High Availability & Resilience: Leverage Kubernetes features like Probes, Rolling Updates, and Horizontal Pod Autoscalers to build highly available and resilient .NET Core applications. Kubernetes is not just about running your applications; it’s about running them well.
Why Care About .NET Core Kubernetes Best Practices?
We live in the era of microservices and containers. Kubernetes is leading the charge, but with .NET Core thrown into the mix, some developers raise their eyebrows. Why?
There’s skepticism around whether .NET Core, known for its prowess in building high-performing, modern, cloud-based applications, can play nice with Kubernetes, the open-source platform king of automating deployment, scaling, and managing containerized applications.
So, let’s clear the air, demystify .NET Core Kubernetes, and highlight its potential.
The Dawn of .NET Core Kubernetes: A Historical Context
.NET Core was introduced by Microsoft with a promise: To build apps that run on any platform. But as the apps grow in complexity, managing them becomes an uphill task. Here enters Kubernetes, adding value to .NET Core, simplifying the complexity with its orchestration capabilities.
Over the years, Kubernetes has emerged as the de facto standard for orchestrating containers, thanks to its robustness and versatility. Still, some developers remain skeptical about the symbiotic relationship between Kubernetes and .NET Core.
Why the Skepticism Around .NET Core Kubernetes?
Let’s admit it. Most of the skepticism stems from the fear of unknown or complexity, coupled with rumors. Some developers think Kubernetes is ‘overkill’ for .NET Core, while others fret about the learning curve. But once you taste the power of .NET Core Kubernetes, there’s no turning back.
Dispelling the Myths
Contrary to what some might think, Kubernetes complements .NET Core beautifully. Kubernetes takes care of the tedious tasks of deploying and scaling your apps, freeing you to focus on building amazing .NET Core apps.
As for the learning curve, there’s a thriving community of .NET Core Kubernetes developers to guide you. Plus, resources abound online!
Expectations from .NET Core Kubernetes
Once you’ve got a grip on .NET Core Kubernetes, expect streamlined app deployment, automated scaling, and seamless management. Plus, with best practices under your belt, you can optimize resources, boost performance, and enhance security.
The Nitty-Gritty of .NET Core Kubernetes: Best Practices in Action
Pardon the interruption as I pull up my sleeves and don my developer hat. With experience in the .NET development trenches and many a battle with Kubernetes, I’m here to deep dive into the world of .NET Core Kubernetes best practices.
1. Craft Your Container Strategy Mindfully
Before you unleash the power of Kubernetes, you need to carefully design your .NET Core applications to live in containers. It’s not about stuffing your existing application into a container, but redesigning your application for a containerized world.
Pro Tip: Embrace a microservices architecture. Break down your .NET Core application into small, independent services. Each service can be containerized and managed individually.
// An example of a simple microservice in .NET Core public class Startup { public void ConfigureServices(IServiceCollection services) { services.AddControllers(); } public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { app.UseHttpsRedirection(); app.UseRouting(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } }
This simple microservice can be containerized and managed individually in Kubernetes.
2. Master Kubernetes Configurations
Kubernetes configurations, written in YAML, can be a daunting maze. Spend quality time understanding and mastering them. It’s worth it!
Pro Tip: Use Helm, the package manager for Kubernetes. Helm charts can help you manage your Kubernetes applications – define, install, and upgrade even the most complex Kubernetes application.
# Sample Helm Chart for a .NET Core application
apiVersion: apps/v1
kind: Deployment
metadata:
name: {{ .Values.app.name }}
labels:
app: {{ .Values.app.name }}
spec:
replicas: {{ .Values.replicaCount }}
selector:
matchLabels:
app: {{ .Values.app.name }}
template:
metadata:
labels:
app: {{ .Values.app.name }}
spec:
containers:
- name: {{ .Values.app.name }}
image: "{{ .Values.image.repository }}:{{ .Values.image.tag }}"
ports:
- containerPort: {{ .Values.app.containerPort }}
3. Leverage Kubernetes Namespaces
Namespaces in Kubernetes allow you to separate and isolate resources. It’s like having multiple virtual clusters in one physical cluster. Namespaces can help you manage your .NET Core apps efficiently.
Pro Tip: Use namespaces for different environments (dev, test, prod) or teams.
apiVersion: v1
kind: Namespace
metadata:
name: dev
This simple command creates a “dev” namespace, which can isolate your development environment from others.
4. Prioritize Security
Never compromise on security. With .NET Core Kubernetes, make sure to follow stringent security best practices.
Pro Tip: Use Role-Based Access Control (RBAC) to manage access to Kubernetes API. Kubernetes Secrets is also a secure way to manage sensitive data like passwords, tokens, or keys.
# An example of an RBAC configuration
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: default
name: pod-reader
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "watch", "list"]
This example configures a role that can read pod data in the default namespace.
5. Invest in Monitoring and Logging
No matter how robust your application, things can go sideways. Monitoring and logging tools can alert you before things get out of hand.
Pro Tip: Leverage tools like Prometheus for monitoring and Elasticsearch for logging.
6. Design for Failure
When building .NET Core applications for Kubernetes, it’s crucial to design for failure. With containers being ephemeral, it’s inevitable that they’ll stop or crash. Kubernetes will try to reschedule them, but your application should also be resilient.
Pro Tip: Implement health checks in your application. Liveness and Readiness Probes are Kubernetes’ way of understanding the health of your containers.
apiVersion: v1
kind: Pod
metadata:
name: myapp-pod
labels:
app: myapp
spec:
containers:
- name: myapp-container
image: myapp
livenessProbe:
httpGet:
path: /health
port: 8080
readinessProbe:
httpGet:
path: /ready
port: 8080
In this YAML file, Kubernetes will periodically check the /health
and /ready
endpoints of your application. If they don’t return a successful response, Kubernetes knows something’s wrong.
7. Zero-Downtime Deployments
One of Kubernetes’ significant benefits is its ability to ensure zero-downtime deployments, a significant advantage when dealing with .NET Core applications.
Pro Tip: Use rolling updates, a Kubernetes feature that gradually replaces old pods with new ones.
kind: Deployment
metadata:
name: myapp
spec:
replicas: 3
strategy:
type: RollingUpdate
rollingUpdate:
maxUnavailable: 1
maxSurge: 1
...
With this configuration, Kubernetes will ensure that at least one replica is always available during updates, thus guaranteeing zero downtime.
8. Scaling Like a Pro
.NET Core applications can be efficiently scaled in Kubernetes, ensuring your applications meet the demand without overutilizing resources.
Pro Tip: Kubernetes supports two types of scaling – horizontal and vertical. Horizontal Pod Autoscaler (HPA) is one tool you should get familiar with.
apiVersion: autoscaling/v1
kind: HorizontalPodAutoscaler
metadata:
name: myapp-hpa
spec:
scaleTargetRef:
kind: Deployment
name: myapp
minReplicas: 1
maxReplicas: 10
targetCPUUtilizationPercentage: 50
This configuration will ensure that your application scales based on CPU utilization, maintaining a balance between demand and resource utilization.
9. Optimize Resource Usage
Kubernetes allows setting resource requests and limits, which can be utilized to optimize resource usage for your .NET Core applications.
Pro Tip: Set appropriate resource requests and limits based on the nature of your application.
apiVersion: v1
kind: Pod
metadata:
name: myapp-pod
spec:
containers:
- name: myapp-container
image: myapp
resources:
requests:
memory: "64Mi"
cpu: "250m"
limits:
memory: "128Mi"
cpu: "500m"
By defining requests and limits, Kubernetes can make better decisions about scheduling pods and can prevent one application from monopolizing resources.
10. Embrace CI/CD
Continuous Integration and Continuous Deployment (CI/CD) is essential in modern application development. Kubernetes
works hand in hand with various CI/CD tools.
Pro Tip: Jenkins, CircleCI, and GitLab are some popular choices for implementing CI/CD for your .NET Core Kubernetes applications.
These are some of the many best practices that you can leverage when building .NET Core applications for Kubernetes. Remember, the goal is to fully utilize Kubernetes’ power while creating resilient, scalable, and efficient applications.
The road might be bumpy, but equipped with the right knowledge and an open mind, you’ll be navigating the landscape like a pro in no time.
Demystifying the Challenges of .NET Core Kubernetes Best Practices
Even with all the advantages that .NET Core Kubernetes brings to the table, it’s not uncommon to hit a few bumps in the road. Having braved the .NET landscape for over a decade and a half, I’ve got a keen eye for these common problems, their consequences, and the solutions.
Problem 1: Complex Configuration Management
One of the main challenges developers often encounter with .NET Core Kubernetes is the complexity of managing configurations, leading to increased error risk and deployment difficulties.
Consequence: Misconfigurations can lead to service disruptions, application failures, and even severe security issues.
Solution: Automation and a solid understanding of Kubernetes configuration files can help overcome this problem. Consider using tools like Helm to simplify the process.
Pro Tip: Helm allows you to package your Kubernetes applications, making it easier to deploy and manage them.
# A simplified Helm chart example
apiVersion: v2
name: myapp
version: 1.0.0
In this example, we’ve created a basic Helm chart. With Helm charts, you can manage your Kubernetes application deployments more effectively.
Problem 2: Handling Persistent Data
Dealing with persistent data in Kubernetes is another challenge that .NET Core developers face. Remember, containers are ephemeral and stateless by nature.
Consequence: Without a proper strategy, data can be lost when a container is terminated or replaced, impacting your application’s reliability.
Solution: Kubernetes provides Persistent Volumes (PV) and Persistent Volume Claims (PVC) to handle persistent data storage.
Pro Tip: With PV and PVC, you can create a persistent disk that lives independently from your containers.
kind: PersistentVolumeClaim
apiVersion: v1
metadata:
name: myapp-pvc
spec:
accessModes:
- ReadWriteOnce
resources:
requests:
storage: 1Gi
In this example, a PVC is created, requesting 1Gi of storage. It can be mounted into your .NET Core application, ensuring data persistence.
Problem 3: Ensuring High Availability and Resilience
Ensuring your .NET Core applications are highly available and resilient in a Kubernetes environment can be tricky.
Consequence: Without proper design, your application might face downtime or performance issues, leading to poor user experience.
Solution: Embrace Kubernetes features like Probes, Rolling Updates, and Horizontal Pod Autoscalers to increase your application’s availability and resilience.
Pro Tip: Liveness and Readiness Probes help Kubernetes understand your application’s health and readiness.
apiVersion: v1
kind: Pod
metadata:
name: myapp-pod
spec:
containers:
- name: myapp-container
image: myapp
livenessProbe:
httpGet:
path: /health
port: 8080
readinessProbe:
httpGet:
path: /ready
port: 8080
With these probes, Kubernetes can ensure that your application is running smoothly and take corrective actions if anything goes amiss.
Understanding and addressing these challenges head-on can significantly enhance your .NET Core Kubernetes journey.
Remember, the key lies in understanding Kubernetes’ intricacies and leveraging its powerful features to your advantage. With a little patience and a lot of practice, you’ll be avoiding these issues in no time.
Addressing the Skepticism
Despite all the advantages, some skepticism will persist. That’s all right. But remember, innovation often comes wrapped in skepticism. The key is to start small, understand the processes, and progressively take on bigger challenges.
Remember, the .NET Core Kubernetes ecosystem is thriving and continuously evolving. So, keep learning and adapting.
Five frequently asked questions and their answers related to .NET Core Kubernetes best practices.
1. How can I securely store sensitive information for my .NET Core application in Kubernetes?
Expert Key Point: Kubernetes has a feature called Secrets to store sensitive data like passwords, API keys, or tokens.
Pro Tip: Never put sensitive data in your code or Dockerfile. Instead, use Kubernetes Secrets.
apiVersion: v1
kind: Secret
metadata:
name: myapp-secret
type: Opaque
data:
connectionstring: [base64 encoded string]
2. How can I auto-scale my .NET Core application based on custom metrics in Kubernetes?
Expert Key Point: Kubernetes supports autoscaling based not only on CPU and memory usage but also on custom metrics like HTTP requests per second.
Pro Tip: Use the Kubernetes Metrics Server and the custom metrics API for complex scaling needs.
apiVersion: autoscaling/v2beta2
kind: HorizontalPodAutoscaler
metadata:
name: myapp-hpa
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: myapp
minReplicas: 1
maxReplicas: 10
metrics:
- type: Pods
pods:
metric:
name: http_requests
target:
type: AverageValue
averageValue: 200
3. How can I effectively manage multiple environments (Dev, QA, Prod) for my .NET Core application in Kubernetes?
Expert Key Point: You can use Kubernetes Namespaces to segregate different environments.
Pro Tip: Consider using Helm for templating your Kubernetes objects, making it easier to manage different environment configurations.
apiVersion: v1
kind: Namespace
metadata:
name: dev
4. How can I manage traffic and implement load balancing for my .NET Core application in Kubernetes?
Expert Key Point: Kubernetes has a Service object which provides network access to a set of pods and can also distribute network traffic.
Pro Tip: Use Kubernetes Ingress along with an Ingress Controller like Nginx for more complex traffic routing.
apiVersion: v1
kind: Service
metadata:
name: myapp-service
spec:
selector:
app: myapp
ports:
- protocol: TCP
port: 80
targetPort: 8080
5. How can I ensure my .NET Core application recovers quickly from failures in Kubernetes?
Expert Key Point: Kubernetes has built-in self-healing features like Probes and ReplicaSets.
Pro Tip: Leverage Kubernetes Deployments for managing the desired state of your application, ensuring quick recovery from failures.
apiVersion: apps/v1
kind: Deployment
metadata:
name: myapp
spec:
replicas: 3
...
These are just a few of the many questions that .NET Core developers might have while adopting Kubernetes. By understanding these best practices and applying them in your Kubernetes journey, you’ll be well-equipped to build robust, scalable, and efficient applications.
Wrapping It Up
.NET Core and Kubernetes can make a powerful team when handled properly. They can transform the way you build, deploy, and manage apps. So, embrace the best practices, start small, keep learning, and you’ll find your sweet spot in this promising landscape.
Ready to get started with .NET Core Kubernetes? Don’t forget to share your thoughts and experiences as you embark on this journey. And keep challenging the status quo.
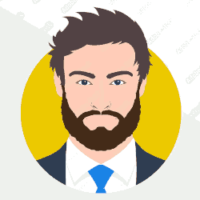
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.