“Why .NET Core is better than .NET Framework?” is the golden question on the minds of many developers today.
What is .NET Core?
.NET Core is a cross-platform, open-source framework for building modern, cloud-based, and internet-connected applications. In other words, it’s your magic wand for creating applications that can run on Windows, Linux, and macOS, or even within Docker containers.
Why .NET Core is Important
The importance of .NET Core lies in its flexibility and versatility. It delivers high performance and scalability, with the bonus of side-by-side installations that minimize versioning conflicts. And let’s not forget its cross-platform prowess, which extends your applications’ reach beyond Windows.
What is .NET Framework?
The .NET Framework, a predecessor to .NET Core, is a software framework developed by Microsoft. It provides a controlled programming environment for Windows-based applications. In layman’s terms, it’s a toolkit that allows you to develop programs that run smoothly on Windows.
Why .NET Framework is Important
The .NET Framework’s importance is rooted in its extensive support for Windows applications, and its rich library for web, desktop, and services development. Despite its Windows-only focus, it provides a robust and mature environment for enterprise-level applications.
Frameworks in Programming: The Basics
In programming, a framework is essentially a pre-fabricated structure that developers can use as a shortcut, avoiding the need to build an application from scratch. It’s akin to a cookie-cutter for building houses; it provides a blueprint and tools to build efficiently and effectively.
The Significance of a Framework
Frameworks are crucial in programming because they simplify the development process, provide consistency across projects, and improve code readability and maintainability. In essence, they offer a common language for developers, boosting collaboration and reducing time to market.
Why Use .NET Core?
The choice to use .NET Core is typically driven by the need for versatility, high performance, and modern application architectures. The open-source nature of .NET Core allows for more significant community engagement, faster updates, and a broad spectrum of ideas and contributions.
.NET Core vs .NET Framework: When to Use Each?
Opt for .NET Core when you’re developing cross-platform applications, microservices, or Docker container applications. Choose .NET Framework when your app is heavily reliant on .NET technologies that aren’t available in .NET Core, or when you’re extending existing .NET Framework applications.
Is .NET Core More Secure Than .NET Framework?
Both .NET Core and .NET Framework have strong security features, but .NET Core shines with its cross-platform capabilities and side-by-side installations, reducing risks related to system-wide upgrades and outdated dependencies.
Why Switch to .NET Core?
If you’re looking for improved performance, cross-platform capabilities, or microservices architecture, it’s time to consider the switch to .NET Core. Its active development community and open-source nature mean it’s continually evolving and improving.
.NET Core vs .NET Framework: The Showdown
Is .NET Core faster than .NET Framework? Indeed, .NET Core has been shown to perform better than .NET Framework in various benchmark tests. It has been optimized for modern hardware and software designs, lending it a performance edge.
.NET Core vs .NET Framework: The Differences
Here’s a concise comparison table highlighting their key differences:
.NET Core | .NET Framework | |
---|---|---|
Cross-Platform|Yes|No| |Open Source|Yes|No| |Performance|High|Good| |Library|Smaller, modular|Extensive| |Runtime Environment|Side-by-side versions possible|Machine-wide versions|
Code Comparison Samples
Let’s compare a simple “Hello, World!” program in both:
.NET Framework
using System;
namespace HelloWorld
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
}
.NET Core
using System;
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
Notice the namespace definition in the .NET Framework code? .NET Core doesn’t require this, offering a simpler and more streamlined coding experience.
Expanding on Why .NET Core Outshines .NET Framework
Let’s delve deeper into why .NET Core claims the upper hand over .NET Framework, exploring several expert key points along with illustrative examples, code comparisons, and tables.
Seamless Cross-Platform Development
Arguably one of .NET Core’s most revolutionary features is its ability to facilitate seamless cross-platform development. Whether you’re targeting Windows, Linux, or macOS, .NET Core ensures your codebase remains consistent and performant.
Let’s further examine this trait and why it’s making waves in the development community.
Unification of Codebase
With .NET Core, developers can maintain a unified codebase for applications running on different platforms. It means less time spent managing and synchronizing separate codebases, resulting in lower costs and higher productivity. Your ASP.NET Core web application will run equally well on a Linux server as it would on a Windows one, without requiring any platform-specific alterations.
Docker and Containerization
In the era of cloud computing, containerization and microservices architecture have taken center stage. Here, .NET Core shows its strength. With its cross-platform capabilities, you can create Docker images that can run on any system that supports Docker.
This compatibility opens up a wide range of possibilities for development and deployment, including easier scaling, better resource usage, and platform independence.
Let’s compare Dockerfiles for an ASP.NET Core application for both Linux and Windows:
Dockerfile for Linux
FROM mcr.microsoft.com/dotnet/core/sdk:3.1 AS build-env
WORKDIR /app
COPY *.csproj ./
RUN dotnet restore
COPY . ./
RUN dotnet publish -c Release -o out
FROM mcr.microsoft.com/dotnet/core/aspnet:3.1
WORKDIR /app
COPY --from=build-env /app/out .
ENTRYPOINT ["dotnet", "YourApp.dll"]
Dockerfile for Windows
FROM mcr.microsoft.com/dotnet/core/sdk:3.1-nanoserver-1903 AS build-env
WORKDIR /app
COPY *.csproj ./
RUN dotnet restore
COPY . ./
RUN dotnet publish -c Release -o out
FROM mcr.microsoft.com/dotnet/core/aspnet:3.1-nanoserver-1903
WORKDIR /app
COPY --from=build-env /app/out .
ENTRYPOINT ["dotnet", "YourApp.dll"]
Observe how both Dockerfiles remain identical, except for the base image. This demonstrates .NET Core’s seamless cross-platform support.
Consistent Developer Experience
.NET Core not only provides a consistent runtime environment but also ensures a consistent developer experience, regardless of the platform. Whether you use Visual Studio on Windows, Visual Studio Code on Linux, or even CLI commands in a macOS terminal, you’ll find the development experience remains largely consistent.
Cross-Platform Libraries
.NET Core’s standard libraries are designed to be cross-platform, which means they work the same way regardless of the underlying operating system. You no longer need to use #ifdefs
or conditional compilation to write platform-specific code.
Leveraging the Ecosystem
Given its cross-platform nature, .NET Core allows developers to leverage the vast ecosystem of third-party libraries and tools that support multiple platforms. This includes everything from database connectors to cloud communication libraries.
In essence, .NET Core’s seamless cross-platform development capability brings about a fundamental shift in how we develop .NET applications. It allows us to write applications that are platform-agnostic while enabling us to tap into the capabilities and strengths of various platforms.
Microservices Architecture
With the rise of cloud-based applications and distributed systems, the microservices architectural style has gained traction. It allows you to break your application into small, loosely coupled services that can be developed, deployed, and scaled independently. .NET Core, with its lightweight and modular nature, is perfectly suited for this architectural style.
Imagine you’re developing an e-commerce application. With .NET Core, you could build independent services for user authentication, inventory management, and payment processing. This design makes it easier to update, scale, and maintain individual parts of your application.
Performance in .NET Core
When it comes to building efficient, high-performing applications, .NET Core indeed sets the bar high. Microsoft has been upfront about .NET Core’s performance enhancements, which result from architectural changes, library improvements, and a focus on modern hardware utilization.
So, let’s dissect how these changes reflect in .NET Core’s performance.
High Throughput
A prime factor contributing to .NET Core’s performance prowess is its ability to handle large volumes of requests concurrently. In high-load scenarios, like in a busy e-commerce website during a sale, .NET Core shines by serving numerous requests per second with minimal delays. The TechEmpower Benchmark showcases this with .NET Core consistently ranking at the top for high-throughput scenarios.
Improved Garbage Collection
In the world of .NET, garbage collection (GC) is a critical aspect of memory management. It is responsible for cleaning up unreferenced objects, freeing up memory. .NET Core has made significant improvements in its GC, leading to less frequent GC pauses, which translates into smoother and more efficient application performance.
JIT Compilation
Just-In-Time (JIT) Compilation is another performance booster in .NET Core. The .NET Core’s JIT compiler, called RyuJIT, provides faster startup and execution times due to its efficient compilation process.
For example, an application startup time can be noticeably faster with .NET Core compared to .NET Framework.
Span and Memory
Introduced in .NET Core, Span<T>
and Memory<T>
types are game-changers for managing memory efficiently. They allow direct access to memory in a type-safe manner without any allocations, dramatically improving the performance of applications working with large amounts of data or frequent memory operations.
Let’s compare the memory usage of a string manipulation operation in both .NET Framework and .NET Core:
.NET Framework
string largeString = GetLargeString();
string substring = largeString.Substring(10, 20);
In .NET Framework, the Substring
operation creates a new string object, leading to unnecessary memory allocation.
.NET Core
string largeString = GetLargeString();
ReadOnlyMemory<char> substring = largeString.AsMemory().Slice(10, 20);
In .NET Core, Slice
operation on Memory<char>
doesn’t create a new string, reducing memory allocation and improving performance.
Benchmarks
BenchmarkDotNet, an open-source .NET benchmarking tool, demonstrates the performance improvements in .NET Core. Let’s take a look at a simplified version of such a benchmark:
[Benchmark]
public string FrameworkSubstring()
{
return largeString.Substring(10, 20);
}
[Benchmark]
public ReadOnlyMemory<char> CoreSlice()
{
return largeString.AsMemory().Slice(10, 20);
}
The CoreSlice
method shows significantly better performance and less memory allocation compared to the FrameworkSubstring
method.
The enhanced performance of .NET Core doesn’t mean .NET Framework is lackluster. But, if you’re looking for faster execution times, higher throughput, and more efficient memory usage, .NET Core is the clear winner.
And with Microsoft’s continued commitment to performance improvements in .NET Core, it’s poised to raise the bar even higher.
High-Density Hosting
For businesses, this could be a significant point. If you’re hosting multiple applications, .NET Core’s ability to run applications side-by-side with different versions leads to fewer conflicts and easier upgrades. This high-density hosting capability can result in substantial cost savings.
Open-Source Nature
Being an open-source platform, .NET Core benefits from community engagement and contributions. This results in faster innovation and updates, a wider range of libraries and tools, and enhanced security due to many eyes scrutinizing the code.
Code Comparison
Now, let’s look at a code comparison that highlights the simplicity and modern approach of .NET Core. We’ll create a simple API:
.NET Framework
public class ValuesController : ApiController
{
public IEnumerable<string> Get()
{
return new string[] { "value1", "value2" };
}
public string Get(int id)
{
return "value";
}
}
.NET Core
[ApiController]
[Route("[controller]")]
public class ValuesController : ControllerBase
{
[HttpGet]
public ActionResult<IEnumerable<string>> Get()
{
return new string[] { "value1", "value2" };
}
[HttpGet("{id}")]
public ActionResult<string> Get(int id)
{
return "value";
}
}
In .NET Core, the use of Route
and HttpGet
attributes offers a more explicit mapping of methods to HTTP verbs and URLs, contributing to readability and maintainability.
The Future is .NET Core
With Microsoft’s decision to merge .NET Core and .NET Framework into a single framework (.NET 5 and onward), it’s evident that the future of .NET development lies in the path set by .NET Core. Thus, making the switch to .NET Core now would set you on the right path for future development.
The Verdict
While the .NET Framework has served us well for many years, it’s clear that .NET Core is the way forward. Its cross-platform support, high performance, open-source nature, and alignment with modern software architectures make it a compelling choice over .NET Framework.
Remember, though, that every project has unique needs and constraints, so choose the technology that best fits your specific circumstances. Still, it’s hard to deny that the factors mentioned above make a strong case for why .NET Core is better than .NET Framework.
The Verdict: Which is Better?
In the battle between .NET Core and .NET Framework, the former takes the trophy for its cross-platform capabilities, improved performance, and open-source dynamism. However, .NET Framework still holds relevance for Windows-focused applications.
Conclusion
If the question, “why .NET Core is better than .NET Framework” has been a thorn in your side, I hope this post provided some clarity. Although .NET Core edges out in this comparison, remember to choose based on your project needs. Happy coding!
I encourage you to share your thoughts or experiences on this topic. Let’s keep the conversation going.
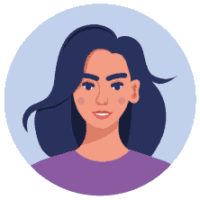
Jessica is a highly accomplished technical author specializing in scientific computer science. With an illustrious career as a developer and program manager at Accenture and Boston Consulting Group, she has made significant contributions to the successful execution of complex projects.
Jessica’s academic journey led her to CalTech University, where she pursued a degree in Computer Science. At CalTech, she acquired a solid foundation in computer systems, algorithms, and software engineering principles. This rigorous education has equipped her with the analytical prowess to tackle complex challenges and the creative mindset to drive innovation.
As a technical author, Jessica remains committed to staying at the forefront of technological advancements and contributing to the scientific computer science community. Her expertise in .NET C# development, coupled with her experience as a developer and program manager, positions her as a trusted resource for those seeking guidance and best practices. With each publication, Jessica strives to empower readers, spark innovation, and drive the progress of scientific computer science.