If you’re wondering, “Can you run .NET Core on Mac?” the answer is a resounding yes! The open-source, cross-platform framework is fully compatible with macOS, allowing developers to create powerful applications on their Mac computers.
.NET Core
.NET Core is a free, open-source, cross-platform, and modular framework for building modern, high-performance, and cloud-based applications. It was first released by Microsoft in 2016 as a successor to the .NET Framework.
The main goal of .NET Core is to provide developers with a unified framework that can run on multiple platforms, including Windows, Linux, and macOS. It provides a set of APIs, tools, and runtime components that enable developers to create a wide range of applications, from web applications and microservices to desktop applications and gaming engines.
.NET Core is designed to be lightweight, modular, and scalable. It includes a set of core libraries that provide essential functionality for building applications, such as collections, IO, networking, and security. It also supports multiple programming languages, including C#, F#, and Visual Basic.
Overall, .NET Core is a powerful and flexible framework that can help developers build modern applications that can run anywhere.
Compatibility of .NET Core with Mac Operating Systems
One of the benefits of .NET Core is its cross-platform compatibility, which includes Mac operating systems. .NET Core can run on macOS, making it an excellent choice for developers who prefer Mac machines.
The latest version of .NET Core supports macOS 10.13 or higher, and the .NET Core runtime can be installed on macOS using a simple command-line interface. It is also compatible with Visual Studio Code, a popular integrated development environment (IDE) for Mac.
Keep in mind that some specific requirements and considerations may vary depending on the macOS version and the development tools used. For example, if you are using Visual Studio for Mac, you will need to ensure that your version supports the .NET Core SDK you plan to use.
Setting Up a .NET Core Development Environment on Mac
Before you can begin developing with .NET Core on your Mac, you need to set up your development environment. Here are the steps involved:
- Install Visual Studio Code: Visual Studio Code is a lightweight and versatile code editor that supports .NET Core development on Mac. Download and install Visual Studio Code from the official website.
- Install .NET Core SDK: The .NET Core SDK contains the necessary tools and libraries to build and run .NET Core applications. Download and install the latest version of .NET Core SDK from the official website.
- Install C# extension for Visual Studio Code: The C# extension for Visual Studio Code adds support for C# language features, debugging, and project templates. Install the extension from the Visual Studio Code Marketplace.
- Create a .NET Core project: Open Visual Studio Code and create a new folder for your project. In the terminal, navigate to the project folder and type dotnet new console to create a new .NET Core console application.
- Run the project: Type dotnet run in the terminal to build and run the project. You should see a message displayed in the console.
Once you have completed these steps, you are ready to start developing your .NET Core applications on your Mac. However, keep in mind that there may be additional software or tools required for specific types of applications or projects.
Creating Your First .NET Core Application on Mac
Now that we have set up our .NET Core development environment on our Mac, it’s time to create our first application. Let’s get started!
Open up your terminal and navigate to the directory where you want to create your application. Once you’re in the directory, type the following command:
dotnet new console -o myapp
This command creates a new console application named “myapp”.
Next, navigate into the “myapp” directory:
cd myapp
Now, let’s build and run our application:
dotnet run
You should see the following output in your terminal:
Hello World!
You have created your first .NET Core application on a Mac!
Let’s take a closer look at the code. Open the “Program.cs” file in your favorite text editor. You should see the following code:
using System; |
---|
namespace myapp |
{ |
class Program |
{ |
static void Main(string[] args) |
{ |
Console.WriteLine(“Hello World!”); |
} |
} |
} |
This code initializes a new console application with a single method, “Main”. This method executes the console application and prints “Hello World!” to the console using the “Console.WriteLine” method.
From here, you can start building more complex .NET Core applications on your Mac using your preferred text editor or integrated development environment (IDE). Happy coding!
Exploring the Power of .NET Core on Mac
One of the key benefits of using .NET Core on a Mac is its versatility. Its cross-platform compatibility allows developers to build and deploy applications for various operating systems. .NET Core on Mac also provides a wide range of tools and libraries for creating different types of applications, including web, desktop, and mobile applications.
Let’s take a look at some of the features and capabilities of .NET Core on Mac:
Feature | Description |
---|---|
Cross-platform compatibility | .NET Core on Mac supports creating and running applications on different operating systems, including Windows, Linux, and macOS. |
Support for multiple languages | .NET Core on Mac supports a variety of programming languages, including C#, F#, and Visual Basic. |
High performance | With its just-in-time (JIT) compilation and runtime optimization, .NET Core on Mac offers high performance for running and executing applications. |
Open-source | .NET Core on Mac is open-source, which means developers can contribute to the community and customize the framework to meet their specific needs. |
Here are some examples of what you can achieve with .NET Core on Mac:
- Create web applications using ASP.NET Core and Razor Pages.
- Build desktop applications using Windows Presentation Foundation (WPF) and Universal Windows Platform (UWP).
- Develop mobile applications using Xamarin.Forms and NativeScript.
- Work with databases using Entity Framework Core and LINQ.
- Use dependency injection and middleware to manage application components and functionality.
Here is an example of how to create a simple web application using .NET Core on Mac:
using System;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
namespace MyWebApplication
{
public class Startup
{
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.Run(async (context) =>
{
await context.Response.WriteAsync("Hello, World!");
});
}
}
}
This code creates a minimal ASP.NET Core web application that responds with “Hello, World!” when run. The application can be built and run using the following commands in the Terminal:
dotnet new web -o MyWebApplication
cd MyWebApplication
dotnet run
Once the application is running, you can access it in a web browser at http://localhost:5000.
As you can see, .NET Core on Mac offers a wide range of tools and features for building modern applications. Whether you’re creating a web, desktop, or mobile application, .NET Core provides a powerful and flexible framework for meeting your development needs.
Comparing .NET Core with Other Frameworks on Mac
While .NET Core is a powerful and versatile framework for Mac development, it is not the only option available. Here, we will compare .NET Core with two popular frameworks: Java and Python.
Java
Java is a mature and robust programming language that offers many benefits for Mac developers. One key advantage of Java is its cross-platform compatibility, allowing developers to write code that can run on multiple operating systems, including macOS. Java also has a vast community of developers and resources, making it easy to find support and solutions to common problems.
One area where .NET Core surpasses Java is in speed. Thanks to its just-in-time (JIT) compilation and optimized runtime, .NET Core can offer faster performance and better memory management.
Let’s take a look at an example of reading and printing a file in Java:
import java.io.*; public class ReadFile
{ public static void main(String[] args)
{ try { File file = new File("example.txt");
BufferedReader br = new BufferedReader(new FileReader(file)); String st; while
((st = br.readLine()) != null) { System.out.println(st); } br.close(); }
catch (IOException e) { System.out.println("An error occurred.");
e.printStackTrace(); } } }
Python
Python is a popular programming language for Mac development, known for its simplicity and ease of use. Python has a vast library of modules and packages that can be easily integrated into projects, making it great for data analysis and scientific computing.
One advantage of .NET Core over Python is its stronger typing system and compile-time checks, which can help catch errors early and improve code quality. Additionally, .NET Core has better support for concurrency and asynchronous programming.
Here’s an example of reading and printing a file in Python:
file = open("example.txt", "r") print(file.read()) file.close()
As you can see, Python’s syntax is more concise and readable, making it great for small-scale scripting and prototyping.
Overall, choosing between .NET Core, Java, and Python will depend on the specific needs and requirements of your project. .NET Core offers fast performance and strong typing, while Java offers cross-platform compatibility and a robust community, and Python offers simplicity and flexibility.
Leveraging .NET Core Libraries and Packages on Mac
One of the key advantages of using .NET Core on a Mac is the extensive library and package ecosystem available to developers. These libraries and packages provide pre-built functionality and tools that can save time and effort when developing applications.
When working with .NET Core on a Mac, developers can take advantage of popular libraries such as Entity Framework Core for data access, SignalR for real-time communication, and AutoMapper for object mapping. These libraries are easily accessible through NuGet, a package manager for .NET.
Developers can also create their own libraries and packages based on their application’s unique needs. These custom libraries and packages can be shared and reused among different projects, further increasing efficiency and productivity.
Using NuGet to Manage Packages
NuGet is a package manager that makes it easy to find, install, and manage .NET Core libraries and packages. It is integrated with Visual Studio for Mac, making it even easier to add packages to a project.
To add a package using NuGet in Visual Studio for Mac:
- Select the project where the package will be added
- In the Solution explorer, right-click on the project and select “Add” -> “Add NuGet Packages”
- Search for the package by name or browse available packages
- Select the desired package and click “Add Package”
Once a package is added, its functionality can be accessed through the appropriate namespaces and classes in the code.
Example: Using Entity Framework Core
Entity Framework Core is a popular library used for data access in .NET Core applications. To use Entity Framework Core in a .NET Core project on a Mac:
- Add the Entity Framework Core package using NuGet, as described above
- Create a DbContext class that represents the database and its entities
- Add DbSet properties to the DbContext for each entity
- Use LINQ queries to access data from the database
Here is an example of using Entity Framework Core to retrieve all products from a database:
// Create a DbContext for the database
public class ProductContext : DbContext
{
public DbSet<Product> Products { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
=> optionsBuilder.UseSqlite("Data source=products.db");
}
// Retrieve all products using LINQ query
using (var context = new ProductContext())
{
var products = from p in context.Products select p;
// Use products list
}
Using Entity Framework Core and other libraries and packages can greatly simplify development and increase productivity in .NET Core applications on a Mac.
Best Practices for .NET Core Development on Mac
If you want to ensure efficient development and optimal performance when working with .NET Core on a Mac, it’s important to follow some best practices. Here are some tips to help you get started:
1. Use Dependency Injection
Dependency injection is a powerful feature of .NET Core that enables you to easily manage dependencies and make your code more modular. It’s a good practice to use dependency injection whenever possible, as it can improve the maintainability and testability of your code.
2. Use Async/Await
Asynchronous programming is a key feature of .NET Core that can significantly improve the performance of your applications. Make sure to use the Async/Await keywords whenever possible, as they can help you create more responsive and scalable applications.
3. Optimize Performance
When working with .NET Core on a Mac, it’s important to optimize the performance of your code to ensure that your applications run smoothly. This can involve techniques such as minimizing memory usage, improving caching, and reducing I/O operations.
4. Follow Naming Conventions
Adopting a consistent naming convention is essential for writing clean and maintainable code. Make sure to follow the standard naming conventions for .NET Core when naming your classes, variables, and methods.
5. Debug Effectively
Debugging is an essential part of the software development process, and it’s important to know how to debug effectively when working with .NET Core on a Mac. Make sure to leverage the built-in debugging tools in Visual Studio or Visual Studio Code, and learn how to use breakpoints, watch windows, and other debugging features.
6. Stay Up to Date
The .NET Core framework is constantly evolving, and it’s important to stay up to date with the latest developments and updates. Make sure to regularly check for updates, read the release notes, and take advantage of new features and improvements.
By following these best practices, you can ensure that your .NET Core applications on a Mac are efficient, reliable, and performant. Keep in mind that these are just guidelines, and you should always adapt them to your specific project and requirements.
Troubleshooting Common Issues in .NET Core on Mac
Working with .NET Core on a Mac can come with its fair share of challenges. Here are some of the most common issues developers may face and troubleshooting tips to solve them:
1. Installation Errors
One common issue developers encounter is errors during installation. This can occur for various reasons, such as missing dependencies or conflicting software. To resolve this, ensure that your system meets the minimum requirements for .NET Core, and try installing it again. If problems persist, check the error logs for more information, and consult the .NET Core documentation or community forums for guidance.
2. Debugging Issues
Debugging is an essential aspect of software development, and it can be frustrating when it doesn’t work as expected. One common issue is breakpoints not hitting, which can happen due to various reasons, such as incorrect configurations or build settings. To resolve this, check that you have set up your debug environment correctly, and try cleaning and rebuilding your solution. If problems persist, try running your application with the dotnet run command instead of using your IDE’s built-in debugger.
3. Performance Problems
Performance is critical for any application, and slow performance can frustrate users and damage your application’s reputation. One way to optimize performance is to use the dotnet publish command to compile your application for a specific runtime and architecture. You can also use profiling tools to identify performance bottlenecks and optimize your code accordingly. Additionally, avoid using inefficient algorithms or loops, and ensure that you are using the correct data structures for your application’s needs.
4. Compatibility Issues
Another common issue is compatibility problems between .NET Core and other software or libraries. This can happen due to version mismatches or conflicting dependencies. To resolve this, ensure that you are using the correct versions of all required software and libraries, and watch out for any deprecation notices or breaking changes. Additionally, keep your system up-to-date with the latest patches and updates, and consult the documentation or community forums for guidance on troubleshooting compatibility issues.
5. Cross-Platform Issues
While .NET Core is cross-platform, there may still be issues when running your application on different operating systems or architectures. One way to ensure cross-platform compatibility is to write platform-agnostic code and use libraries that support multiple platforms. Additionally, test your application on different platforms and architectures to ensure that it works as expected. If problems persist, consult the documentation or community forums for guidance on troubleshooting cross-platform issues.
By keeping these common issues and troubleshooting tips in mind, you can minimize the frustration and downtime of working with .NET Core on a Mac and ensure a smoother development experience.
Running .NET Core on a Mac has become increasingly popular among developers due to its compatibility and versatility.
External Resources
https://learn.microsoft.com/en-us/dotnet/core/install/macos
FAQ
FAQ 1: How to Install .NET Core on a Mac?
Q: Can I install .NET Core on a Mac, and if so, how?
A: Yes, you can install .NET Core on a Mac. You typically download and install it from Microsoft’s official website or use a package manager like Homebrew.
Code Sample:
# Using Homebrew to install .NET Core
brew install --cask dotnet
FAQ 2: How to Create a New .NET Core Project on a Mac?
Q: How do I create a new .NET Core project on a Mac?
A: Use the .NET CLI to create a new project. Open the Terminal and use the dotnet new
command.
Code Sample:
# Create a new console application
dotnet new console -o MyConsoleApp
cd MyConsoleApp
FAQ 3: How to Run a .NET Core Application on a Mac?
Q: How do I run a .NET Core application on a Mac?
A: After creating a .NET Core application, use the dotnet run command in the Terminal to run your application.
Code Sample:
# Navigate to your project directory
cd MyConsoleApp
# Run the application
dotnet run
FAQ 4: How to Build a .NET Core Application on a Mac?
Q: What is the process for building a .NET Core application on a Mac?
A: Use the dotnet build command in the Terminal to build your .NET Core application.
Code Sample:
# Navigate to your project directory
cd MyConsoleApp
# Build the application
dotnet build
FAQ 5: How to Add NuGet Packages to a .NET Core Project on a Mac?
Q: How can I add NuGet packages to my .NET Core project on a Mac?
A: Use the dotnet add package command to add NuGet packages to your project.
Code Sample:
# Navigate to your project directory
cd MyConsoleApp
# Add a NuGet package, e.g., Newtonsoft.Json
dotnet add package Newtonsoft.Json
These FAQs cover the basics of getting started with .NET Core on a Mac, from installation to building and running applications, as well as managing dependencies with NuGet packages.
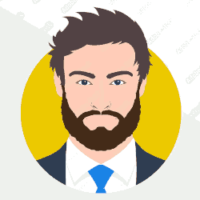
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.