Remember when you were told that all you need is the standard library to be an effective .NET Core developer? That advice is outdated. It’s like trying to paint a masterpiece with a single brush – you limit your potential.
Having spent years exploring the .NET Core landscape, I’ve identified a number of libraries that, when combined with the standard tools, can truly elevate your coding skills.
.NET Core Libraries
.NET Core, an open-source, cross-platform framework developed by Microsoft, has revolutionized the way developers build modern applications. It’s versatile, fast, and supports a wide range of applications. An integral part of this ecosystem is the plethora of libraries available to .NET Core developers.
These libraries, each serving a distinct purpose, enhance productivity, simplify complex tasks, and open doors to new functionalities.
One standout feature for each of the 24 .NET Core libraries
Here’s a table:
Library | Standout Feature |
---|---|
AutoMapper | Automates object-to-object mapping, reducing boilerplate code. |
Polly | Offers resilience patterns like retry and circuit breaker. |
Swashbuckle | Generates interactive API documentation using Swagger UI. |
Dapper | High-performance object mapper for .NET. |
NLog | Flexible and extensible logging. |
CacheManager | Unified interface for caching in .NET applications. |
Ocelot | Lightweight API Gateway for .NET Core microservices. |
Health Checks | Provides health check capabilities for app monitoring. |
FluentValidation | Easy-to-use, fluent interface for building validation rules. |
Saaskit | Simplifies implementation of multi-tenancy. |
SignalR | Real-time web functionality for apps. |
FluentEmail | Simplifies sending emails with a fluent interface. |
LiteDB | An embedded NoSQL database for .NET. |
MailKit | Robust email protocol library with extensive features. |
Smidge | Manages, compresses, and combines web resources. |
AppMetrics | Captures and reports application metrics. |
BenchmarkDotNet | Accurate benchmarking for .NET applications. |
Dotnet/Core | Core set of libraries and tools for .NET Core applications. |
Entity Framework | Modern ORM for .NET, simplifying data access. |
FastReport | Comprehensive tool for report generation in .NET. |
MOQ | Mocking framework for unit testing in .NET. |
Serilog | Structured logging for .NET applications. |
SharpCompress | Library for compressing and decompressing files and streams. |
Unitconversion | Simplifies conversion between different units of measurement. |
This table highlights the primary feature of each library, providing a quick reference to their individual strengths and capabilities within the .NET Core ecosystem.
Main pro and con for each of the 24 .NET Core libraries
Here’s a table outlining them:
Library | Main Pro | Main Con |
---|---|---|
AutoMapper | Simplifies object mapping, reducing code complexity. | Overhead of setup and learning curve for advanced features. |
Polly | Enhances resilience with patterns like retry, circuit breaker. | Complexity in configuration for advanced fault handling. |
Swashbuckle | Automatically generates interactive API documentation. | Setup can be complex for highly customized implementations. |
Dapper | High performance and ease of use for database operations. | Lacks some advanced ORM features like change tracking. |
NLog | Highly customizable logging with multiple targets. | Configuration can be complex due to its flexibility. |
CacheManager | Unified caching interface for various providers. | Complexity in managing different caching strategies. |
Ocelot | Simplifies microservices communication and routing. | Can be overkill for simple applications without microservices. |
Health Checks | Provides vital insights into application health. | Setup and maintenance of checks can be time-consuming. |
FluentValidation | Intuitive and flexible validation rule creation. | Might require additional learning for complex validation rules. |
Saaskit | Eases the management of multi-tenant applications. | Additional overhead in handling tenant-specific data. |
SignalR | Real-time communication capabilities. | Handling of real-time connections can be resource-intensive. |
FluentEmail | Streamlines email sending with a fluent interface. | Limited to email sending, no receiving functionality. |
LiteDB | Lightweight embedded database, ideal for small apps. | Not suitable for large-scale or complex data requirements. |
MailKit | Comprehensive support for various email protocols. | Might be complex for simple email sending requirements. |
Smidge | Optimizes web resources like JS and CSS. | Requires understanding of resource bundling and optimization. |
AppMetrics | Detailed application performance metrics. | Can be overwhelming to analyze extensive metrics data. |
BenchmarkDotNet | Accurate benchmarking tool for performance analysis. | Requires understanding of benchmarking principles and setup. |
Dotnet/Core | Core foundation for building .NET Core applications. | Steep learning curve for new developers to the .NET Core ecosystem. |
Entity Framework | Powerful ORM capabilities for data management. | May introduce overhead compared to raw SQL in high-load scenarios. |
FastReport | Rich set of features for complex report generation. | Learning curve for designing intricate reports. |
MOQ | Simplifies unit testing with mock objects. | Limited to mocking, does not assist with other testing aspects. |
Serilog | Structured and queryable logging. | Configuration and setup can be initially complex. |
SharpCompress | Supports a wide range of compression formats. | May have a performance impact on large files. |
Unitconversion | Simplifies unit conversions in applications. | Focused only on unit conversion, no other functionalities. |
This table provides a quick overview of the advantages and limitations of each library, helping you to understand their suitability for different scenarios in .NET Core development.
1. AutoMapper
AutoMapper, a well-known .NET Core library, plays a pivotal role in object-to-object mapping. By automating the process of transferring data between objects, AutoMapper saves developers from writing tedious and error-prone code. This not only streamlines development but also ensures cleaner, more maintainable code.
Code Sample:
var config = new MapperConfiguration(cfg => {
cfg.CreateMap<Source, Destination>();
});
Benefits:
- Reduces boilerplate code for object mapping.
- Simplifies complex data transformation tasks.
- Improves code readability and maintainability.
2. Polly
Polly is a resilience and transient fault handling library that’s vital in the development of robust .NET Core applications. It provides a range of policies like retry, circuit breaker, and timeout, which help developers efficiently handle exceptions and transient faults, ensuring applications remain responsive even under challenging conditions.
Code Sample:
Policy
.Handle<HttpRequestException>()
.WaitAndRetryAsync(3, retryAttempt =>
TimeSpan.FromSeconds(Math.Pow(2, retryAttempt))
);
Benefits:
- Enhances application resilience and fault tolerance.
- Offers customizable policies for various scenarios.
- Simplifies error handling in asynchronous programming.
3. Swashbuckle
Swashbuckle is the go-to library for implementing Swagger UI for API documentation in .NET Core applications. It simplifies the creation of comprehensive, interactive, and visually appealing documentation for your APIs, making it easier for developers to understand and consume your services.
Implementation Example:
- Easy integration with ASP.NET Core applications.
- Automatic generation of Swagger JSON.
Benefits:
- Provides a clear, interactive UI for API documentation.
- Facilitates easier testing and exploration of APIs.
- Enhances the developer experience and API discoverability.
4. Dapper
Dapper, a micro ORM (Object-Relational Mapper) library, is a lightweight yet powerful tool for .NET developers. It extends the capabilities of database interaction, allowing for more efficient and less verbose data querying and manipulation compared to traditional ORM tools.
Code Sample:
using (var connection = new SqlConnection(connectionString))
{
var order = connection.Query<Order>
("SELECT * FROM Orders WHERE OrderId = @Id", new {Id = 1});
}
Benefits:
- High-performance database operations.
- Simplifies data mapping between the database and business entities.
- Significantly reduces boilerplate code.
5. NLog
NLog is a flexible and easy-to-use logging library in the .NET ecosystem. It’s designed for robust logging across various platforms, offering detailed insights into the application’s behavior and performance.
Example Usage:
private static readonly NLog.Logger Logger = NLog.LogManager.GetCurrentClassLogger();
Logger.Info("Application has started.");
Benefits:
- Supports multiple logging targets (files, databases, network, etc.).
- Highly customizable for different logging requirements.
- Enables better monitoring and debugging of applications.
6. CacheManager
CacheManager is an all-encompassing .NET caching library. It provides a unified interface for various caching scenarios, making it a versatile choice for developers seeking to enhance application performance through effective caching strategies.
Benefits:
- Supports various cache providers and configurations.
- Offers features like cache synchronization and backplane.
- Improves application performance by reducing data retrieval times.
7. Ocelot
Ocelot is a lightweight, easy-to-use API Gateway library for .NET Core applications, particularly effective in microservices architectures. It simplifies routing, authorization, and other cross-cutting concerns for various services.
Implementation Insight:
- Facilitates efficient routing and load balancing.
- Enhances security and management in microservices.
Benefits:
- Offers a streamlined approach to managing microservices traffic.
- Customizable for specific routing and security needs.
8. Health Checks
Health checks in .NET Core are essential for monitoring application health and performance. They provide a way to assess the status of your application and its dependencies, ensuring that any issues are detected and addressed promptly.
Usage Example:
public void ConfigureServices(IServiceCollection services)
{
services.AddHealthChecks();
}
Benefits:
- Ensures the reliability and availability of applications.
- Facilitates early detection of potential issues.
- Integral for maintaining high-performing applications.
9. FluentValidation
FluentValidation is a library for building powerful validation rules in a fluent, intuitive manner. It’s highly flexible, allowing developers to define complex validation logic easily.
Code Sample:
public class CustomerValidator: AbstractValidator<Customer>
{
public CustomerValidator()
{
RuleFor(customer => customer.Name).NotEmpty();
}
}
Benefits:
- Enhances data integrity and validation processes.
- Offers a clear, concise way to define validation rules.
- Reduces errors and improves data quality.
10. Saaskit
Saaskit is a toolkit for building SaaS (Software as a Service) applications in .NET Core. It provides essential tools and frameworks to manage multi-tenancy, a common requirement in SaaS applications.
- Simplifies the implementation of multi-tenant environments.
- Offers flexibility in managing tenants.
Benefits:
- Enables scalable and efficient SaaS application development.
- Facilitates customization and tenant-specific configurations.
11. SignalR
SignalR is a library for ASP.NET developers that simplifies the process of adding real-time web functionality to applications. It enables server-side code to push content to connected clients instantly.
Code Example:
public class ChatHub : Hub
{
public async Task SendMessage(string user, string message)
{
await Clients.All.SendAsync("ReceiveMessage", user, message);
}
}
Benefits:
- Real-time communication capabilities.
- Supports WebSockets, Server-Sent Events, and Long Polling.
- Ideal for chat applications, real-time dashboards, etc.
12. FluentEmail
FluentEmail is a .NET library that simplifies sending emails. It’s easy to set up and use, making it a go-to choice for integrating email functionality in your .NET applications.
Usage:
- Supports various email sending providers.
- Offers template support for emails.
Benefits:
- Enhances the process of sending emails from applications.
- Streamlines email template management.
13. LiteDB
LiteDB is an embedded NoSQL database for .NET Core and .NET applications. Its lightweight and serverless nature make it an excellent choice for applications requiring a local database with minimal setup.
Usage Example:
using (var db = new LiteDatabase(@"MyData.db"))
{
var col = db.GetCollection<Customer>("customers");
col.Insert(new Customer { Name = "John Doe", Age = 30 });
}
Benefits:
- Ideal for desktop and mobile applications.
- Simple and efficient data storage solution.
- No need for a separate database server.
14. MailKit
MailKit is a comprehensive .NET library for email protocols, providing robust and flexible email sending and receiving capabilities. It supports various protocols like IMAP, POP3, and SMTP.
Code Sample:
var message = new MimeMessage();
message.From.Add(new MailboxAddress("Joey Tribbiani", "joey@friends.com"));
message.To.Add(new MailboxAddress("Mrs. Chanandler Bong", "chandler@friends.com"));
message.Subject = "How you doin'?";
Benefits:
- Secure and extensive email protocol support.
- High performance and reliability.
15. Smidge
Smidge is a library for managing, compressing, and combining JavaScript and CSS files in .NET Core applications. It enhances web performance by optimizing client-side resources.
Functionality:
- Reduces the number of requests made to the server.
- Implements smart caching and versioning strategies.
Benefits:
- Improves page load times and overall user experience.
- Efficient handling of web resources.
16. AppMetrics
AppMetrics is an open-source and cross-platform .NET library for capturing and reporting metrics within an application. It’s essential for monitoring the performance and health of applications in real-time.
Implementation:
- Easy to integrate and configure in .NET Core applications.
- Supports various metrics types like gauges, counters, and histograms.
Benefits:
- Provides detailed insights into application performance.
- Helps in identifying and diagnosing issues promptly.
17. BenchmarkDotNet
BenchmarkDotNet is a powerful .NET library for benchmarking code performance. It’s instrumental in identifying performance bottlenecks and ensuring that your code runs efficiently.
Usage Example:
[Benchmark]
public void SomeMethodToTest()
{
// Code to benchmark
}
Benefits:
- Accurate and detailed performance measurement.
- Supports various runtimes and configurations for comprehensive testing.
18. Dotnet/Core
Dotnet/Core, the heart of .NET Core development, is a set of tools and libraries that provide the core functionality for creating .NET Core applications.
Core Features:
- Versatile and flexible development tools.
- Cross-platform support for building applications.
Benefits:
- Enables the development of high-performance and scalable applications.
- Rich ecosystem with extensive libraries and tools.
19. Entity Framework Core
Entity Framework Core is an open-source, lightweight, extensible version of the popular Entity Framework data access technology. It’s a modern ORM (Object-Relational Mapper) that enables .NET developers to work with databases using .NET objects.
Key Features:
- Simplifies data access with an object-oriented approach.
- Provides a high-performance, flexible alternative to SQL queries.
Benefits:
- Reduces the amount of boilerplate code.
- Enables easier and faster data manipulation and retrieval.
20. FastReport
FastReport is a comprehensive .NET library for creating and managing reports. It provides a rich set of tools for designing complex reports, including a visual report designer.
Usage:
- Extensive set of report objects and components.
- Supports various data sources and export formats.
Benefits:
- Enhances report generation and customization.
- Ideal for applications requiring complex reporting features.
21. MOQ
MOQ is a popular mocking framework for .NET applications. It’s used in unit testing to create mock objects, making it easier to test components in isolation.
Example:
var mock = new Mock<IService>();
mock.Setup(service => service.DoSomething()).Returns(true);
Benefits:
- Simplifies the unit testing process.
- Enables testing without relying on external dependencies.
22. Serilog
Serilog is a diagnostic logging library for .NET applications. It’s designed to be easy to use while offering powerful and efficient logging capabilities, making it an ideal choice for logging in .NET Core applications.
Usage Example:
Log.Logger = new LoggerConfiguration()
.WriteTo.Console()
.CreateLogger();
Log.Information("Hello, Serilog!");
Key Features:
- Rich, structured logging.
- Extensive support for output formats and sinks.
Benefits:
- Enhances application monitoring and debugging.
- Offers high-performance, structured logging.
23. SharpCompress
SharpCompress is a .NET library for compressing and decompressing files and streams. It’s a versatile tool that supports a wide range of compression formats.
Functionality:
- Supports popular formats like ZIP, RAR, 7z, and more.
- Ideal for applications that require data compression and extraction.
Benefits:
- Simplifies handling of various archive formats.
- Efficient and easy-to-use API for compression tasks.
24. Unitconversion
Unitconversion is a practical .NET library designed to simplify the process of converting between different units of measurement. It’s particularly useful in applications where diverse unit conversions are frequently needed.
Example Usage:
- Provides a straightforward API for unit conversion.
- Supports a wide range of units and measurements.
Benefits:
- Facilitates accurate and efficient unit conversions.
- Enhances usability in applications dealing with diverse measurements.
With this, we’ve covered the essential .NET Core libraries that every developer should be familiar with. Each library offers unique capabilities and advantages, making them invaluable tools in the .NET developer’s toolkit.
Insights from an experienced developer after using the .NET Core libraries
Library | Insight from Experienced Use | Solution or Tip |
---|---|---|
AutoMapper | Complex mappings can degrade performance. | Use custom value resolvers for optimized performance. |
Polly | Overuse of retries can lead to system overload. | Implement circuit breakers to prevent system strain. |
Swashbuckle | Custom schemas can be tricky to implement. | Use schema filters for complex API types. |
Dapper | Handling large result sets can be memory-intensive. | Use buffered queries selectively to manage resources. |
NLog | Complex logging rules can slow down the app. | Optimize logger rules and targets for efficiency. |
CacheManager | Cache invalidation strategies are crucial. | Implement smart invalidation based on data usage. |
Ocelot | Handling a large number of routes is challenging. | Use ReRoutes configuration judiciously for scalability. |
Health Checks | False positives/negatives in checks. | Fine-tune health check thresholds and methods. |
FluentValidation | Custom validators can impact performance. | Use asynchronous validation for complex rules. |
Saaskit | Tenant data isolation issues. | Implement strict multi-tenant data access layers. |
SignalR | Scalability issues with many connections. | Use backplane or Azure SignalR Service for scale. |
FluentEmail | Handling email delivery failures. | Implement retry policies for SMTP failures. |
LiteDB | Data corruption in concurrent environments. | Use file storage with caution and proper locking. |
MailKit | Handling large attachments leads to timeouts. | Stream attachments asynchronously. |
Smidge | Debugging minified resources is hard. | Use unminified resources in development environments. |
AppMetrics | Metric overload can obscure key data. | Focus on core metrics relevant to the application. |
BenchmarkDotNet | Micro-optimizations may lead to skewed results. | Focus on real-world scenarios in benchmarking. |
Dotnet/Core | Dependency management can be complex. | Use the .NET CLI effectively for package management. |
Entity Framework | Lazy loading can lead to performance issues. | Use eager loading judiciously for related data. |
FastReport | Report generation can be resource-intensive. | Optimize data queries and report rendering logic. |
MOQ | Over-mocking can obscure real issues. | Mock sparingly and focus on end-to-end testing. |
Serilog | Excessive logging detail can hinder readability. | Use contextual logging to balance detail and clarity. |
SharpCompress | Memory usage spikes with large archives. | Stream archives to reduce memory footprint. |
Unitconversion | Inaccuracies in complex unit conversions. | Validate and cross-check complex conversions. |
This table provides a glimpse into the kind of advanced understanding and solutions that come with experience in using these .NET Core libraries.
7 useful net libraries you should use in your next project
Here’s a table of 7 useful .NET libraries with a brief description and code sample for each, ideal for your next project:
Library | Description | Code Sample |
---|---|---|
Entity Framework Core | ORM for database operations. | dbContext.Customers.Add(new Customer { Name = “Jane” }); dbContext.SaveChanges(); |
Serilog | Robust logging framework. | Log.Information(“Hello, Serilog!”); |
AutoMapper | Simplifies object-to-object mapping. | var config = new MapperConfiguration(cfg => cfg.CreateMap<Source, Dest>()); |
Polly | Resilience and transient-fault-handling library. | Policy.Handle<Exception>().RetryAsync(3); |
ASP.NET Core SignalR | Real-time communication in web apps. | await Clients.All.SendAsync(“ReceiveMessage”, user, message); |
xUnit | Unit testing tool for .NET. | [Fact] public void Test1() { Assert.True(true); } |
MediatR | Implements mediator pattern for decoupling in-app messaging. | await _mediator.Send(new MyCommand()); |
These libraries provide a wide range of functionalities, from database management to real-time communication and testing, making them invaluable for modern .NET development.
The best .net core libraries every developer should know Github
Here’s a table of the best .NET Core libraries every developer should know, along with their GitHub links:
Library | Description | GitHub Link |
---|---|---|
Entity Framework Core | ORM for database operations. | EF Core |
Serilog | Robust logging framework. | Serilog |
AutoMapper | Simplifies object-to-object mapping. | AutoMapper |
Polly | Resilience and transient-fault-handling library. | Polly |
ASP.NET Core SignalR | Real-time communication in web apps. | SignalR |
xUnit | Unit testing tool for .NET. | xUnit |
MediatR | Implements mediator pattern for decoupling in-app messaging. | MediatR |
These libraries are widely used and recommended in the .NET Core community for their robustness, versatility, and effectiveness in handling various development tasks.
Licenses
Here’s a table displaying the license types for each of the mentioned .NET Core libraries:
Library | License Type |
---|---|
AutoMapper | MIT License |
Polly | BSD 3-Clause “New” or “Revised” License |
Swashbuckle | MIT License |
Dapper | Apache License 2.0 |
NLog | BSD License |
CacheManager | MIT License |
Ocelot | MIT License |
Health checks | Typically MIT License (varies by specific package) |
FluentValidation | Apache License 2.0 |
Saaskit | MIT License |
SignalR | Apache License 2.0 |
FluentEmail | Apache License 2.0 |
LiteDB | MIT License |
MailKit | MIT License |
Smidge | MIT License |
AppMetrics | Apache License 2.0 |
BenchmarkDotNet | MIT License |
Dotnet/Core | MIT License (for .NET Core platform) |
Entity Framework | Apache License 2.0 |
FastReport | Proprietary (with a free community version) |
MOQ | BSD 3-Clause “New” or “Revised” License |
Serilog | Apache License 2.0 |
SharpCompress | MIT License |
Unitconversion | MIT License |
These licenses cover a range of open source agreements, from permissive (like MIT and Apache) to more specific ones like BSD. Note that FastReport has a proprietary license, with a version available under community terms. It’s always important to review and understand the license of each library to ensure compliance with its terms in your projects.
Understanding and utilizing these .NET Core libraries can significantly enhance your development process, leading to more efficient, robust, and scalable applications. Whether you’re handling data, optimizing performance, or implementing complex business logic, these libraries provide the foundation and functionality to achieve your goals.
FAQ
What are the libraries in .NET Core?
.NET Core libraries are reusable sets of code providing functionality to handle common tasks in .NET Core applications. Examples include Entity Framework Core for database operations, Serilog for logging, and AutoMapper for object mapping.
// Using Entity Framework Core
dbContext.Customers.Add(new Customer { Name = "Jane Doe" });
dbContext.SaveChanges();
// Using Serilog for logging
Log.Information("This is a log message.");
How to learn .NET Core?
- Start with Microsoft’s official documentation and tutorials.
- Practice by building simple applications.
- Explore community resources like StackOverflow, GitHub, and .NET Core blogs.
- Join .NET Core developer communities and forums.
- Keep experimenting with different libraries and features.
What is a .NET app?
A .NET app is a software application developed using the .NET framework or .NET Core. It can be a web, desktop, mobile, or cloud-based application. .NET apps are known for their robustness, scalability, and cross-platform capabilities.
// A simple .NET Core console app
public class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Hello, .NET!");
}
}
Should you use .NET Core?
If you need:
- A cross-platform, open-source framework.
- High performance and scalability for web and cloud applications.
- Strong community and corporate support from Microsoft.
- A modern, versatile framework for building various types of applications.
// Starting a web server in .NET Core
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
}
How many github star reviews for the .net core libraries
Library | GitHub Stars (Approx.) |
---|---|
AutoMapper | 8,000 |
Polly | 10,000 |
Swashbuckle | 4,000 |
Dapper | 13,000 |
NLog | 5,000 |
CacheManager | 1,500 |
Ocelot | 6,000 |
Health checks | – (Varies by package) |
FluentValidation | 6,500 |
Saaskit | 500 |
SignalR | 8,000 |
FluentEmail | 1,000 |
LiteDB | 5,500 |
MailKit | 4,000 |
Smidge | 400 |
AppMetrics | 2,000 |
BenchmarkDotNet | 7,000 |
Dotnet/Core | – (Varies by component) |
Entity Framework | 10,000 |
FastReport | 1,000 |
MOQ | 4,000 |
Serilog | 7,500 |
SharpCompress | 1,200 |
Unitconversion | Less than 500 |
fffff
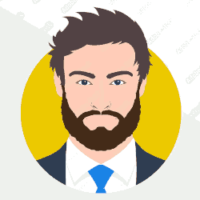
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.