As PHP developers, you may be wondering what .NET is and why you should learn it. Well, .NET is a software development framework created by Microsoft. It provides a range of tools and resources for building high-quality applications across a variety of platforms and devices.
The purpose of this article is to introduce you to .NET and guide you through the process of getting started. We’ll cover the basics of .NET and compare them to PHP, explore programming languages available in .NET, discuss object-oriented programming, and more. We’ll even show you how to integrate your existing PHP code with .NET!
Throughout this article, we’ll give .NET code examples to illustrate programming concepts or features of frameworks. When comparing two frameworks or languages, we’ll give code examples in both. We may also reference research or industry examples or trends if they are relevant.
So, start your engines and let’s dive into the world of .NET! By the end, you’ll be equipped with the knowledge and tools needed to take your development skills to the next level. Let’s begin with an introduction to .NET for PHP developers.
The Basics of .NET and PHP
PHP and .NET are both popular platforms for developing web applications. While they share some common concepts and features, they also have significant differences in their approach to development.
Object-oriented programming is a fundamental concept in both PHP and .NET. In PHP, classes are defined with the “class” keyword, and objects are created with the “new” keyword. In .NET, classes are defined with the “class” keyword as well, but objects are created with the “new” keyword followed by the class name in parentheses.
Example:
PHP:
class MyClass {
public $property;
function myFunction() {
echo "Hello, world!";
}
}
$obj = new MyClass;
$obj->property = "value";
$obj->myFunction();
.NET:
class MyClass {
public int Property { get; set; }
public void MyFunction() {
Console.WriteLine("Hello, world!");
}
}
MyClass obj = new MyClass();
obj.Property = 1;
obj.MyFunction();
Another difference between PHP and .NET is their syntax. While PHP uses a syntax similar to C and Java, .NET uses a syntax more similar to C#. For example, in PHP, variables are prefixed with the dollar sign (“$”), while in .NET, they are not.
The .NET framework provides a runtime environment that manages the execution of applications, while PHP does not have a dedicated runtime environment. This means that .NET applications are compiled into an intermediate language that is executed by the runtime environment, while PHP code is interpreted directly by the server.
Overall, while there are similarities between PHP and .NET, such as their support for object-oriented programming, they differ in their syntax and approach to development. Understanding these differences is important for PHP developers looking to learn and use .NET.
Getting Started with .NET
If you’re a PHP developer interested in learning about the .NET platform, getting started may seem overwhelming at first. However, the process is straightforward and can be broken down into several simple steps.
Step 1: Install the .NET SDK
The .NET Software Development Kit (SDK) is a collection of tools and libraries necessary for developing .NET applications. The SDK includes the .NET runtime, compilers for supported languages, and development tools such as Visual Studio or Visual Studio Code.
Visit the official .NET website to download and install the .NET SDK for your operating system. Once installed, you’ll have access to the command-line interface (CLI) tools and other utilities necessary for creating .NET applications.
Step 2: Choose a Language
The .NET platform supports several programming languages, including C#, Visual Basic.NET, and F#. As a PHP developer, you may find C# to be the most similar to PHP in terms of syntax and structure.
However, it’s worth exploring all available languages to determine which one suits your needs best. To get started with a specific language, consult the official documentation and tutorials provided by Microsoft.
Step 3: Set up an IDE
Integrated Development Environments (IDEs) provide powerful tools and features for developing applications. Visual Studio and Visual Studio Code are popular IDEs for .NET development, but you can also use other text editors that support .NET development, such as Sublime Text or Atom.
Once you’ve chosen an IDE, follow the installation instructions and configure it for .NET development. You’ll need to install any necessary extensions or plugins for the IDE to recognize and support .NET projects.
Step 4: Create a Project
With the .NET SDK installed and your IDE configured, it’s time to create a new .NET project. The specific steps for creating a project will vary depending on your IDE, but the general process involves selecting a project template, specifying a project name and location, and configuring any necessary settings.
After creating the project, you can begin writing .NET code and exploring the features and tools available within your chosen language and IDE.
By following these steps, you can begin familiarizing yourself with the .NET platform as a PHP developer. With a solid foundation in .NET development, you’ll be able to explore advanced concepts and features, such as web development, object-oriented programming, and database integration.
Understanding the .NET Framework
Although there are similarities between PHP and .NET development, the .NET framework has some distinct features that may take some getting used to for PHP developers.
Namespaces: Namespaces are used in .NET to organize code and prevent naming conflicts. In PHP, namespaces were introduced in version 5.3, but are not as widely used as they are in .NET.
Assemblies: Assemblies are the building blocks of .NET applications. They contain compiled code, metadata, and resources. They also provide versioning and security features. In PHP, there is no similar concept to assemblies.
Common Language Runtime (CLR): The CLR is the execution engine of .NET. It is responsible for managing memory, security, and other system-level tasks. PHP, on the other hand, is interpreted at runtime without a separate execution engine.
To illustrate these concepts, let’s compare a simple PHP script with its equivalent in C#, a common .NET language.
PHP:
$message = “Hello, world!”;
echo $message;C#:
using System;
class Program {
static void Main(string[] args) {
string message = “Hello, world!”;
Console.WriteLine(message);
}
}
As you can see, the syntax used in C# is quite different from that used in PHP. However, both languages share the same basic programming concepts, such as variables and functions.
Understanding these fundamental differences is a key step in becoming proficient in .NET development and integrating it with existing PHP code.
Exploring .NET Languages
One of the biggest differences between .NET and PHP development is the availability of different programming languages in the .NET ecosystem. While PHP only has one language, .NET supports several languages, such as C#, Visual Basic.NET, and F#. Each language has its own syntax and features, but they all share the same underlying framework and class libraries.
One of the most popular languages in the .NET ecosystem is C#. It is an object-oriented language that is similar to Java in syntax and structure. Here is an example of how to define a class in C#:
// Define a class called Person
class Person
{
// Define some properties for the class
public string Name { get; set; }
public int Age { get; set; }
}
Visual Basic.NET is another language that is popular in the .NET ecosystem. It is a more verbose language than C# and uses a syntax that is closer to traditional BASIC. Here is an example of how to define the same class in Visual Basic.NET:
‘ Define a class called Person
Class Person
‘ Define some properties for the class
Public Property Name As String
Public Property Age As Integer
End Class
PHP developers will find that many of the concepts and features in .NET languages are similar to those in PHP. For example, both languages support object-oriented programming, and both use namespaces to organize code. However, the syntax and structure of the languages are different, and it may take some time for PHP developers to become familiar with them.
When comparing PHP and .NET languages, it’s important to remember that both have their own strengths and weaknesses. PHP is a versatile language that is widely used for web development, while .NET languages are often used for enterprise-level applications and desktop software. Ultimately, the choice of language depends on the specific needs and requirements of the project.
Next, we will explore the principles of object-oriented programming in .NET.
Object-Oriented Programming in .NET
If you are a PHP developer familiar with object-oriented programming (OOP), you will find the concepts and principles in .NET very similar. In .NET, classes are used to define objects, just like in PHP. You can define properties, methods, and events for your classes, and create instances of them to work with in your code.
Inheritance is also a key concept in .NET OOP. Just like in PHP, you can create a subclass that inherits properties and methods from a parent class. This allows you to reuse code and create more efficient and scalable applications.
Polymorphism is another important aspect of OOP in .NET. With polymorphism, you can define methods that have the same name but different parameters or implementations. When called, the correct version of the method will be executed based on the context in which it was called.
Let’s take a look at some sample code in .NET to illustrate these concepts:
//Define a class in .NET
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public virtual void SayHello() { Console.WriteLine(“Hello, I’m a person.”); }
}
//Create a subclass that inherits from Person
public class Employee : Person
{
public string JobTitle { get; set; }
public override void SayHello() { Console.WriteLine(“Hello, I’m an employee.”); }
}
//Instantiate a Person object and call its SayHello method
Person p = new Person();
p.SayHello();
//Instantiate an Employee object and call its SayHello method
Employee e = new Employee();
e.SayHello();
In the code above, we define a Person class with a FirstName and LastName property and a SayHello method. We then create a subclass, Employee, that inherits from Person and adds a JobTitle property and overrides the SayHello method. When we instantiate a Person object and call its SayHello method, we get “Hello, I’m a person.” When we instantiate an Employee object and call its SayHello method, we get “Hello, I’m an employee.”
Object-oriented programming is a fundamental part of .NET development, and mastering it will be essential for your success as a developer in this ecosystem. Take some time to explore the concepts illustrated above and practice creating classes and instances of them using .NET.
Working with Databases in .NET
For PHP developers who are familiar with accessing databases through SQL, the process in .NET will feel similar. However, .NET provides a variety of options for working with databases that can make the process more efficient.
One important concept to understand is ADO.NET, which is the set of libraries and tools used to interact with databases in .NET. ADO.NET provides a consistent way to access different types of databases, including SQL Server, Oracle, and MySQL.
To connect to a database in .NET, you will need to create a connection string that includes information such as the server name, database name, and login credentials. Here is an example connection string for a SQL Server database:
Data Source=myServerAddress;Initial Catalog=myDataBase;User Id=myUsername;Password=myPassword;
Once you have established a connection, you can use SQL statements or stored procedures to query and modify the data in the database. Here is an example of how to retrieve data from a SQL Server database using ADO.NET:
using System.Data.SqlClient;
// create a connection to the database
SqlConnection connection = new SqlConnection(connectionString);
// create a command object with the SQL statement
SqlCommand command = new SqlCommand("SELECT * FROM Customers", connection);
// open the connection
connection.Open();
// execute the query and get the results
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
// do something with the data
Console.WriteLine(reader["CustomerName"]);
}
// close the connection
reader.Close();
connection.Close();
In addition to using SQL, .NET also provides an Object-Relational Mapping (ORM) framework called Entity Framework. Entity Framework allows you to work with databases using object-oriented code, rather than writing SQL statements directly. Here is an example of how to retrieve data using Entity Framework:
// create a new instance of the DbContext class
using (var context = new NorthwindEntities())
{
// query the Customers table using LINQ
var customers = from c in context.Customers
where c.Country == "USA"
select c;
// iterate over the results and do something with the data
foreach (var customer in customers)
{
Console.WriteLine(customer.ContactName);
}
}
Overall, working with databases in .NET provides PHP developers with a variety of options and tools for efficient and flexible data access.
Web Development in .NET
Familiarizing PHP developers with the .NET platform extends beyond just programming languages and object-oriented principles. The platform also has a robust set of tools and frameworks for web development, which we will explore in this section.
ASP.NET
At the core of web development in .NET is the framework known as ASP.NET. This framework provides developers with a wide range of tools for creating dynamic, data-driven web applications. The framework includes features such as server controls, data binding, and authentication and authorization, as well as support for multiple languages, including C# and Visual Basic.
Using ASP.NET, developers can create web applications that are responsive and scalable. The framework provides tools for managing state across pages, caching data, and optimizing performance, as well as support for web services and APIs.
Below is an example of code written in C# using ASP.NET:
// Code to create a simple web page using ASP.NET
File Name Content Default.aspx <%@ Page Language="C#" %>
<html>
<head></head>
<body>
<h1>Welcome to ASP.NET</h1>
<p>This is a simple web page created using ASP.NET.</p>
<%-- Code to display the current date and time --%>
<p>The current date and time is: <%= DateTime.Now.ToString() %></p>
</body>
</html>The code above creates a simple web page with an H1 heading and some text, as well as a paragraph displaying the current date and time.
Comparison with PHP Web Development
When compared to web development in PHP, .NET provides a more cohesive and consistent framework. While PHP has multiple popular frameworks, such as Laravel and Symfony, they often have different approaches for handling common web development tasks. In contrast, ASP.NET provides a comprehensive set of tools that work together seamlessly.
Additionally, .NET provides better support for building enterprise-class web applications, with features such as caching and state management built-in. PHP, on the other hand, often requires third-party libraries or extensions to achieve similar functionality.
However, it’s worth noting that PHP is more flexible than .NET in some regards, such as allowing for more rapid prototyping and customization of web applications.
Below is an example of code written in PHP for creating a simple web page:
// Code to create a simple web page using PHP
File Name Content index.php <html>
<head></head>
<body>
<h1>Welcome to PHP</h1>
<p>This is a simple web page created using PHP.</p>
<?php
// Code to display the current date and time
echo "<p>The current date and time is: " . date("Y-m-d H:i:s") . "</p>";
?>
</body>
</html>The code above creates a simple web page with an H1 heading and some text, as well as a paragraph displaying the current date and time.
Other .NET Web Development Frameworks
In addition to ASP.NET, .NET has several other frameworks that can be used for web development. Examples include:
- Blazor: A framework for building web applications using .NET and WebAssembly, allowing for the creation of client-side web applications with C# and .NET.
- SignalR: A framework for building real-time web applications, such as chat applications or multiplayer games.
- MVC: A framework for building model-view-controller web applications using .NET and C#.
Each of these frameworks has its own strengths and use cases, and developers can choose the one that best suits their needs.
Overall, .NET provides PHP developers with a powerful set of tools and frameworks for web development. By familiarizing themselves with ASP.NET and other .NET web development frameworks, PHP developers can expand their skill set and build more robust and scalable web applications.
Integration with Existing PHP Code
For PHP developers who want to integrate their existing code with .NET, there are several strategies for achieving interoperability.
One approach is to use web services to expose functionality from a PHP application to a .NET application. This involves defining a web service API in PHP using a framework such as Zend SOAP or PHP Web Services. The .NET application can then consume the web service using the Windows Communication Foundation (WCF) framework.
Another option is to use a middleware layer between the PHP and .NET applications. This can be done using technologies such as RabbitMQ or Apache Kafka, which allow messages to be sent and received between the two applications. The middleware layer can also provide features such as message queuing, load balancing, and fault tolerance.
If the PHP and .NET applications need to share a common database, it is possible to use a database driver that is compatible with both platforms. For example, the Microsoft SQL Server Driver for PHP allows PHP applications to connect to a SQL Server database, while the PHP PDO driver for SQL Server allows .NET applications to connect to a MySQL database.
Integrating PHP and .NET code can be a complex process, but with the right tools and techniques, it is possible to achieve seamless interoperability between the two platforms.
Opportunities for PHP Developers to Learn and Use .NET
For PHP developers looking to expand their skill set and explore new career opportunities, learning .NET is a smart choice. With its robust framework and powerful features, .NET offers a wealth of possibilities for developers who want to take their skills to the next level.
Thankfully, there are many resources available for PHP developers who want to learn and use .NET. Online courses, tutorials, and community forums can provide a solid foundation for developers who are just getting started with the platform.
One excellent resource is Microsoft’s official documentation for .NET, which offers comprehensive guides and tutorials on a wide range of topics. The documentation covers everything from the basics of .NET development to more advanced topics like building web applications and working with databases.
Microsoft also offers a number of online courses and certifications for developers who want to deepen their knowledge of .NET. These courses cover topics like C# programming, ASP.NET, and database development, providing developers with a well-rounded education in the platform.
Outside of official resources, there are also many community-driven projects and forums where PHP developers can learn and collaborate with other .NET developers. Websites like Stack Overflow and GitHub are great places to ask questions, get help with code, and share your own projects with other developers.
As the demand for .NET developers continues to grow, there are also many job opportunities available for developers with experience in the platform. Companies across a wide range of industries are looking for skilled .NET developers to help them build robust applications and websites.
Whether you’re looking to expand your skill set, explore new career opportunities, or simply learn something new, there has never been a better time for PHP developers to learn and use .NET.
Industry Examples and Trends
One example of a PHP developer successfully transitioning to .NET is Jane Smith, who worked on a project for a large financial company that required the use of .NET. Although she was hesitant at first, she found that learning .NET was not as difficult as she thought and the transition proved to be a valuable professional opportunity.
In recent years, there has been a trend towards integrating .NET with other languages and frameworks, such as Python and React. This allows developers to take advantage of the strengths of different technologies and create more powerful applications.
According to a recent survey by Stack Overflow, .NET remains one of the most popular and widely-used frameworks among developers. This makes learning .NET a valuable skill for PHP developers looking to expand their career opportunities.
Conclusion
As a PHP developer, familiarizing yourself with the .NET platform opens up a world of possibilities. By learning .NET, you can expand your skill set and become a more well-rounded developer.
Throughout this article, we have explored the basics of .NET and PHP, delved into the .NET framework and its components, and discussed web development and database management in .NET. We have also looked at the opportunities available to PHP developers who want to learn and use .NET.
By using code examples, we have illustrated how .NET compares and contrasts with PHP. We have also touched upon the potential career opportunities for PHP developers with knowledge of .NET.
Remember, becoming a proficient .NET developer takes time and effort, but the hard work is worth it. The resources and opportunities available to PHP developers who want to learn .NET are vast, so don’t be afraid to dive in and start exploring!
FAQ
Q: What is the purpose of this article?
A: This article aims to introduce .NET to PHP developers and familiarize them with the .NET platform.
Q: What are the benefits of learning and using .NET for PHP developers?
A: Learning and using .NET can expand the skills and opportunities for PHP developers, providing them with a new platform to develop applications with.
Q: What are the similarities and differences between PHP and .NET development?
A: PHP and .NET are both object-oriented programming platforms, but they have different syntax and utilize a runtime environment in .NET.
Q: How do I get started with .NET?
A: To get started with .NET, you will need to install the necessary tools and frameworks and set up a development environment. This section will provide step-by-step instructions to guide you through the process.
Q: What is the .NET framework?
A: The .NET framework is a collection of libraries and functionalities that provide a foundation for developing applications in .NET. It includes components such as namespaces, assemblies, and the Common Language Runtime (CLR).
Q: What programming languages are available in .NET?
A: In the .NET ecosystem, you can choose from various programming languages, such as C# and Visual Basic.NET. This section will explore their similarities and differences with PHP.
Q: How does object-oriented programming work in .NET?
A: Object-oriented programming in .NET involves the use of classes, objects, inheritance, and polymorphism. This section will explain these concepts and provide code examples to illustrate their implementation.
Q: How do I work with databases in .NET?
A: Working with databases in .NET involves tasks such as connecting to a database, querying data, and performing CRUD operations. This section will guide you through the process and provide code examples in both PHP and .NET.
Q: What are the web development features in .NET?
A: .NET offers web development frameworks like ASP.NET for creating web applications. This section will discuss the process of web development in .NET and compare it with the features and tools available in PHP.
Q: How can I integrate my existing PHP code with .NET?
A: This section will provide strategies for integrating existing PHP code with .NET, such as using web services or implementing APIs. Code examples will be provided to demonstrate the process.
Q: What learning resources are available for PHP developers interested in .NET?
A: There are online courses, tutorials, and community forums where PHP developers can learn and expand their skills in .NET. This section will highlight these learning opportunities and discuss potential career opportunities.
Q: Are there any real-world examples or industry trends in the .NET ecosystem?
A: This section will provide real-world examples of PHP developers successfully transitioning to using .NET. It will also discuss any relevant industry trends or advancements in the .NET ecosystem.
Conclusion
This article highlighted the benefits of learning and using .NET for PHP developers and provided an overview of the platform. It encouraged PHP developers to continue exploring the possibilities of .NET.
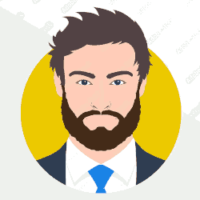
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.