The Relationship between C# .NET and C++ makes developers who are familiar with C++ wonder about the relationship between the two languages and how they compare. When it comes to programming languages, C# .NET and C++ are commonly used in software development.
Key Takeaways
- C# .NET and C++ are both popular programming languages used in software development.
- Developers who are familiar with C++ may want to explore C# .NET and learn about the similarities and differences between the two languages.
- This article will provide insights and recommendations for developers who are transitioning from C++ to C# .NET.
What is C# .NET?
C# .NET is a modern, high-level, object-oriented programming language that enables developers to build applications for the Microsoft .NET framework. It was developed by Microsoft in the early 2000s as a successor to C++, Java, and Visual Basic. C# .NET is widely used for building Windows desktop applications, web applications, and mobile applications.
C# .NET is similar to C++ in many ways, but it offers several improvements over C++, such as garbage collection and simplified syntax. Garbage collection automatically frees up memory that is no longer in use, making it easier for developers to manage memory. Simplified syntax means that C# .NET code is easier to read and write than C++ code.
Developers familiar with C++ will find that many of the programming concepts in C# .NET are similar, such as classes, objects, and inheritance. However, there are also some key differences, such as the use of delegates and events for handling events, which are not present in C++.
What is C++?
Before exploring the relationship between C# .NET and C++, it is important to understand what C++ is and its role in programming languages.
C++ is a general-purpose programming language that was developed as an extension of the C programming language. It was designed to provide features such as object-oriented programming, generic programming, and low-level memory manipulation capabilities.
C++ is a compiled language, meaning that the code is first translated into machine code by a compiler before being executed. It is widely used in developing operating systems, device drivers, video games, and other high-performance applications.
For developers familiar with C++, transitioning to C# .NET can provide benefits such as simplified syntax and automatic memory management. However, there are also differences between the two languages that must be taken into consideration.
Similarities between C# .NET and C++
Although C# .NET and C++ are different programming languages, they share many similarities. Here are some of the key similarities:
Similarity | Description |
---|---|
Object-oriented programming | Both languages support object-oriented programming (OOP) concepts such as encapsulation, inheritance, and polymorphism. |
Data types | Both languages support similar data types, including integers, floating-point numbers, characters, and Boolean values. |
Control structures | Both languages use similar control structures such as if-else statements, loops, and switch statements to control program flow. |
IDEs | Both languages have robust Integrated Development Environments (IDEs) that provide features like intellisense, debugging, and code refactoring. |
These similarities make it easier for developers who are familiar with C++ to transition to C# .NET.
Differences between C# .NET and C++
While C# .NET and C++ share many similarities, there are also significant differences between the two programming languages. These include:
C# .NET | C++ |
---|---|
Managed code | Native code |
Automatic memory management | Manual memory management |
Garbage collection | No garbage collection |
Object-oriented programming | Object-oriented programming |
Support for functional programming | Support for functional programming |
Less control over memory management | More control over memory management |
C# .NET is a managed code language, meaning that it runs within a virtual machine that provides automatic memory management and garbage collection. This allows developers to focus more on writing code and less on maintaining memory allocation and deallocation. C++ is a native code language, meaning that it directly interfaces with the underlying hardware, providing developers with more control over memory management and performance optimization.
C# .NET also has built-in support for object-oriented programming and functional programming, whereas C++ supports object-oriented programming but requires additional libraries for functional programming.
Additionally, C# .NET has simplified syntax that is generally easier to read and write than the syntax required for C++.
However, C++ provides more control over memory management and allows for direct access to hardware, making it a better choice for system-level programming. C++ also has better performance than C# .NET in certain scenarios, although C# .NET has greatly improved performance in recent years.
Transitioning from C++ to C# .NET
If you are an experienced C++ developer looking to transition to C# .NET, there are a few things to keep in mind to make the process smoother.
Firstly, while C# syntax is similar to C++, there are also some significant differences. Take time to familiarize yourself with the new syntax and language constructs. It’s also essential to have a solid understanding of object-oriented programming principles, as they are more central to C# development.
Secondly, memory management works differently in C# than in C++. C# has automatic memory management, which means you don’t need to manually allocate or deallocate memory. Instead, the .NET runtime handles memory management for you. This can make development easier and more efficient, but it also means you need to learn how to work with garbage collection.
Thirdly, the .NET framework provides a rich set of libraries and tools that can help you build powerful applications more quickly. Take the time to learn about the framework and explore the documentation to make the most of its capabilities.
Finally, don’t be afraid to ask for help from the C# community. There are many resources available, including forums, online tutorials, and user groups. Joining a community can be a great way to get support and learn from others who have already made the transition from C++ to C# .NET.
C# .NET Code Examples
To better understand the differences and similarities between C# .NET and C++, let’s take a look at some code examples.
Example 1: Hello World
Here’s how to print “Hello World” in C# .NET:
using System; class Program { static void Main(string[] args) { Console.WriteLine("Hello World!"); } }
Here’s the equivalent code in C++:
#include <iostream> using namespace std; int main() { cout << "Hello World!"; return 0; }
As you can see, the syntax is slightly different between the two languages, but the concept is the same.
Example 2: Adding Numbers
Here’s how to add two numbers in C# .NET:
int num1 = 5; int num2 = 10; int sum = num1 + num2; Console.WriteLine(sum);
Here’s the equivalent code in C++:
int num1 = 5; int num2 = 10; int sum = num1 + num2; cout << sum;
Once again, the concepts are the same, but the syntax is slightly different.
By examining these examples, developers transitioning from C++ to C# .NET can gain insights into the similarities and differences between the two programming languages.
Advantages of C# .NET over C++
While C++ has been around longer and is still used extensively in software development, C# .NET offers several advantages over its predecessor. These benefits include:
Simplified Syntax
C# .NET has a simpler syntax than C++, making it easier for developers to write and read code. With features like automatic memory management, there is less need for manual memory allocation and deallocation. C# .NET also has a more intuitive syntax for functions, making it easier to learn and use.
Automatic Memory Management
One of the biggest advantages of C# .NET over C++ is automatic memory management. C# .NET uses garbage collection to automatically free up memory that is no longer needed, eliminating the need for manual memory allocation and deallocation.
This feature greatly reduces the risk of memory leaks and buffer overflows.
Rich Framework Ecosystem
C# .NET has a rich framework ecosystem that includes a vast library of pre-built code, making it easier to develop applications quickly.
These libraries provide features for everything from basic I/O and networking to database access and web development. C++ also has libraries, but they are often not as extensive or user-friendly as those in C# .NET.
Cross-Platform Compatibility
C# .NET code can be run on multiple platforms with minimal modifications, including Windows, Linux, and macOS. This makes it easier for developers to create applications that can be used on a variety of devices.
In contrast, C++ code can be more difficult to port to different platforms, requiring significant modifications for each platform.
C# .NET | C++ |
---|---|
Simplified Syntax | Complex Syntax |
Automatic Memory Management | Manual Memory Management |
Rich Framework Ecosystem | Less Extensive Libraries |
Cross-Platform Compatibility | Platform-Specific Code Modifications Required |
While C++ is still a highly regarded programming language, C# .NET offers several advantages that make it a compelling choice for developers. Its ease of use, automatic memory management, and rich framework ecosystem make it an excellent choice for software development projects of all sizes and complexity levels.
Use Cases for C# .NET and C++
While C# .NET and C++ have similarities, they also have different strengths that make them suited for different use cases. Here are some scenarios where each language can excel:
Use Cases for C# .NET:
- Web development: C# .NET is ideal for web development due to its compatibility with the ASP.NET platform.
- Desktop applications: C# .NET allows for the creation of user-friendly and visually appealing desktop applications.
- Mobile development: C# .NET is compatible with Xamarin, which allows for the creation of mobile applications for iOS, Android, and Windows.
- Data analysis: With C# .NET’s rich framework ecosystem, it is suitable for data analysis and machine learning tasks.
Use Cases for C++:
- System software: C++ is a low-level language and is ideal for system software development such as operating systems, device drivers, and firmware.
- Game development: C++ is often used for game development due to its performance capabilities and ability to access low-level hardware.
- High-performance computing: With its ability to efficiently manage memory and access hardware, C++ is ideal for high-performance computing tasks such as scientific simulations and rendering.
- Operating systems and embedded systems: Due to its low-level functionality, C++ is often used for developing operating systems and embedded systems.
When choosing between C# .NET and C++, consider the specific needs of your project and what language will best suit those needs. With its ease of use and compatibility with the ASP.NET platform, C# .NET is a good choice for web development and desktop applications. However, if you need the performance capabilities and low-level functionality required for game development or operating systems, C++ may be a better choice.
Tools and Resources for Learning C# .NET
For developers familiar with C++ transitioning to C# .NET, there are numerous tools and resources available to help you get started. Whether you prefer online courses, documentation, or community forums, there is something for everyone.
Here are some recommended resources to help you navigate the transition process:
Microsoft Learn
Microsoft Learn is an online resource that provides comprehensive tutorials and learning paths for C# .NET developers. With courses ranging from beginner to advanced, you can find the right level of learning for your needs. Plus, Microsoft Learn is free and requires no prior experience with C# .NET or any other programming language.
StackOverflow
StackOverflow is a popular community forum where developers can ask and answer questions about C# .NET. With a vast network of experienced developers, you can get insights and guidance on specific coding challenges or general programming concepts. Plus, the site’s search feature makes it easy to find answers to common questions.
Pluralsight
Pluralsight is an online learning platform that offers courses on a wide range of tech topics, including C# .NET. With expert instructors and interactive learning experiences, you can quickly gain practical skills and knowledge to help you transition from C++. The platform also offers a free trial period to test out their courses.
C# Yellow Book
The C# Yellow Book is a free online book that provides a comprehensive introduction to C# .NET programming. Written by Rob Miles, a Microsoft MVP, the book is designed for beginners but can also be useful for experienced developers transitioning from C++. The book is available for download in PDF format.
Github
Github is a code-sharing and collaboration platform that hosts thousands of code repositories for C# .NET programming. You can find examples of real-world projects, learn from other developers’ coding styles, and contribute to open-source projects. Github also offers a repository search feature to help you find the specific code you need.
With these tools and resources, you can successfully make the transition from C++ to C# .NET. Embrace the new advantages and enjoy the learning process.
Conclusion – Relationship between C# .NET and C++
It is clear that C# .NET and C++ are both popular and powerful programming languages that have their unique strengths and weaknesses. Understanding the relationship between these two languages can be valuable for developers who are familiar with one and interested in transitioning to the other.
While there are similarities between C# .NET and C++, there are also notable differences. Developers should consider factors such as syntax, memory management, and platform compatibility when deciding which language to use for a particular project.
Learning a new language can be challenging, but there are many resources available for developers who are interested in learning C# .NET. Hire .Net Developer is a leading outsourcing company that specializes in software development and can provide valuable insights and assistance to developers interested in transitioning to C# .NET.
Ultimately, the decision to use C# .NET or C++ will depend on the specific needs and preferences of each developer and project. However, understanding the relationship between these two languages can help developers make informed decisions and create high-quality software more efficiently and effectively.
About Hire .Net Developer
Hire .Net Developer is a leading software outsourcing company with expertise in providing top-notch services to global clients. Our team comprises experienced developers who specialize in .NET technologies and are well-versed in the latest trends and updates in the software development industry.
We understand the relationship between C# .NET and C++ and offer our services to clients who are seeking to transition from C++ to C# .NET. Our team of experts provides valuable insights and recommendations to ensure a smooth transition process for developers familiar with C++.
We also offer our services to clients who are seeking to explore both languages based on their individual needs and preferences.
If you are interested in our services or have any queries, please feel free to contact us here. Our team will be happy to assist you with your software development needs.
FAQs – Relationship between C# .NET and C++
Q: What is the relationship between C# .NET and C++?
A: C# .NET and C++ are both programming languages used for software development. While they have some similarities, they also have distinct differences in terms of syntax, memory management, and platform targeting.
Q: What is C# .NET?
A: C# .NET is a programming language developed by Microsoft. It is part of the .NET framework and is commonly used for building Windows applications, web services, and other software solutions.
Q: What is Hire .Net Developer?
A: Hire .Net Developer is a software outsourcing company that specializes in providing skilled .NET developers for various software development projects. If you are interested in their services or have any questions, you can contact them at [contact information].
Q: What is C++?
A: C++ is a general-purpose programming language that is widely used for system and application development. It allows for low-level programming and offers more control over memory management compared to C# .NET.
Q: What are the similarities between C# .NET and C++?
A: Both C# .NET and C++ share similarities in terms of their syntax, object-oriented programming capabilities, and support for libraries and frameworks. They also both offer high-performance capabilities for creating complex software applications.
Q: What are the differences between C# .NET and C++?
A: There are several differences between C# .NET and C++. C# .NET has automatic memory management (garbage collection) while C++ requires manual memory management. C# .NET is platform-independent, while C++ can be used to create platform-specific applications. Additionally, C# .NET offers a more simplified syntax compared to C++.
Q: How can I transition from C++ to C# .NET?
A: Transitioning from C++ to C# .NET requires familiarizing yourself with the syntax and concepts of C# .NET. It may also involve learning about the differences in memory management and platform targeting. Taking online courses or seeking guidance from experienced C# .NET developers can help facilitate a smooth transition.
Q: Can you provide some C# .NET code examples?
A: Certainly! Here are a few examples of common programming tasks implemented in C# .NET:
– Creating a class and defining its properties and methods
– Implementing inheritance and interfaces
– Reading and writing files
– Making HTTP requests
– Working with databases
Q: What are the advantages of using C# .NET over C++?
A: Some advantages of using C# .NET over C++ include automatic memory management, a rich framework ecosystem, and simplified syntax. C# .NET also offers increased productivity and faster development times compared to C++.
Q: In which use cases is C# .NET preferred over C++?
A: C# .NET is often preferred in scenarios where rapid development, platform independence, or ease of use are important. It is commonly used for building web applications, desktop applications, and enterprise software solutions. C++, on the other hand, is often employed for system-level programming, game development, and performance-critical applications.
Q: Where can I find resources for learning C# .NET?
A: There are several online resources available for learning C# .NET, including:
– Online courses and tutorials
– Official Microsoft documentation
– Community forums and discussion groups
– Online coding platforms and practice websites
– Books and ebooks on C# .NET programming
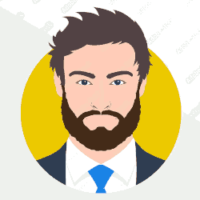
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.