As developers continue to innovate and seek out new technologies to improve their projects, NoSQL databases have emerged as a popular choice for many. NoSQL databases offer unique advantages over traditional relational databases, making them an excellent option for .NET projects. In this guide, we will explore how to use NoSQL with .NET, integrating NoSQL databases in .NET projects and leveraging NoSQL for efficient data storage in .NET applications.
We will cover the benefits and considerations of using NoSQL with .NET and provide guidance on how to set up NoSQL databases in .NET frameworks with ORM libraries. We will dive into the practical aspects of working with NoSQL data in .NET, including CRUD operations and querying techniques specific to NoSQL databases. Additionally, we will explore techniques to maximise the benefits of NoSQL databases in .NET applications, including denormalization and sharding.
Throughout this guide, we will use .NET code examples to illustrate programming concepts or features of frameworks and reference industry trends or research if they are relevant. Whether you are a seasoned .NET developer or are just beginning to explore NoSQL databases, this guide will provide valuable insights into how to use NoSQL with .NET and make the most of its potential. Let’s get started!
Understanding NoSQL Databases
NoSQL databases are non-relational databases that store and manage data differently from traditional relational databases. Unlike relational databases, NoSQL databases do not use tables, rows, and columns to store data. Instead, they use different models such as document databases, key-value stores, column-family stores, and graph databases to store data.
NoSQL databases provide more scalability and flexibility in data storage than traditional relational databases. With NoSQL databases, data can be easily distributed across multiple servers, allowing for faster access and better performance in handling large volumes of data. NoSQL databases are also easier to set up and maintain, making them popular in modern applications that require flexible and scalable data storage.
NoSQL Database Options for .NET
When considering NoSQL databases for .NET projects, developers have several options to choose from, each with its own unique characteristics and advantages. Here, we will explore the four main types of NoSQL databases: document databases, key-value stores, column-family stores, and graph databases.
Document Databases
A document database stores data in a semi-structured format, typically using JSON or BSON documents. These databases are designed for storing and retrieving whole documents, making them a good fit for applications that handle complex or rapidly changing data structures.
Document Database Examples | Features |
---|---|
MongoDB | Highly scalable, supports dynamic schema, enables ad-hoc queries |
RavenDB | ACID compliant, includes a query engine and indexing, supports data replication |
Key-Value Stores
A key-value store is a simple database that stores data as a collection of key-value pairs. These databases are ideal for applications that require fast read and write operations, and are commonly used for caching and session management.
Key-Value Store Examples | Features |
---|---|
Redis | In-memory data storage, supports caching, queues, and pub/sub messaging |
Riak | Distributed data storage, high-availability, fault-tolerant |
Column-Family Stores
A column-family store organizes data into indexed columns, allowing for high scalability and performance. These databases are well-suited for applications that handle large amounts of structured or semi-structured data.
Column-Family Store Examples | Features |
---|---|
Apache Cassandra | Highly scalable, fault-tolerant, supports distributed data storage and replication |
HBase | Provides low-latency, random read/write access to large data sets, runs on top of Hadoop Distributed File System (HDFS) |
Graph Databases
A graph database stores data in nodes and edges, allowing for complex relationships between data points. These databases are ideal for applications that require complex data modeling, such as social networks and recommendation engines.
Graph Database Examples | Features |
---|---|
Neo4j | Highly scalable, supports transactional data storage and querying, provides real-time querying and indexing |
ArangoDB | Multimodal database, supports documents, key-values, and graphs, provides a unified query language |
By understanding the characteristics of each NoSQL database type, .NET developers can choose the most appropriate option for their specific project needs.
Setting Up NoSQL Databases in .NET
Before integrating NoSQL databases in .NET projects, developers need to set up and configure the databases and necessary tools. This involves selecting the appropriate .NET frameworks, ORM libraries, and NoSQL databases. Here, we will provide a step-by-step guide on how to set up NoSQL databases in .NET.
Choose the Right NoSQL Database Option
First, developers need to choose the right NoSQL database option for their project. There are various types of NoSQL databases, including document databases, key-value stores, column-family stores, and graph databases. Each type has its advantages and disadvantages regarding data storage, retrieval, and scalability. Developers need to consider their project requirements and choose the most suitable type for their application.
Select the Appropriate .NET Frameworks
After choosing the right NoSQL database option for their project, developers need to select appropriate .NET frameworks that support the selected NoSQL database. For instance, if working with MongoDB, developers can choose ASP.NET Core, which provides built-in support for MongoDB. If working with Apache Cassandra, developers can choose the Cassandra Driver for .NET.
Choose an ORM Library
Next, developers need to choose an ORM library that can help simplify the process of working with NoSQL databases in .NET. Some popular options include NoRM, RavenDB, and Couchbase. ORM libraries handle the communication between the application and the database, mapping objects to documents and vice versa.
Configure the NoSQL Database
Once the appropriate NoSQL database, .NET framework, and ORM library have been chosen, developers need to configure the database and the necessary tools. Depending on the selected NoSQL database, developers can use command-line tools, graphical user interfaces, or APIs to configure the database.
For example, for MongoDB, developers can use the Mongo Shell to configure the database settings, create users, and set up authentication. For Apache Cassandra, developers can use CQL (Cassandra Query Language) shell to configure the database, create keyspaces, and set up replication.
Code Examples
Here’s an example of using MongoDB with .NET Core:
// Install the MongoDB.Driver NuGet package
using MongoDB.Driver;
// Connect to the MongoDB server
var client = new MongoClient(“mongodb://localhost:27017”);
// Get the database instance
var database = client.GetDatabase(“mydb”);
// Get the collection instance
var collection = database.GetCollection<User>(“users”);
// Insert a new document
var user = new User { Name = “John”, Age = 30 };
collection.InsertOne(user);
Here’s an example of using the Cassandra Driver for .NET:
// Install the CassandraCSharpDriver NuGet package
using Cassandra;
// Connect to the Cassandra cluster
var cluster = Cluster.Builder()
.AddContactPoint(“localhost”)
.Build();
// Get the session instance
var session = cluster.Connect(“mykeyspace”);
// Execute a query
var query = “SELECT * FROM users WHERE age > 20”;
var result = session.Execute(query);
Conclusion
Setting up NoSQL databases in .NET involves selecting the appropriate NoSQL database option, .NET frameworks, and ORM libraries. Once these components are selected, developers need to configure the database and necessary tools. With the right setup, developers can seamlessly integrate NoSQL databases in their .NET applications and take advantage of the benefits of NoSQL.
Working with NoSQL Data in .NET
Now that we have established the benefits of NoSQL in .NET applications, let’s dive into the practical aspects of working with NoSQL data. This section will cover essential operations like CRUD (create, read, update, delete) and querying techniques specific to NoSQL databases.
CRUD Operations
When working with NoSQL databases in .NET, CRUD operations are fundamental to database management. Let’s examine how to perform these operations using .NET code examples.
Create: To create a new document in a NoSQL database, we can use the CreateDocumentAsync method with a Document object. Here’s an example using the MongoDB .NET driver:
var collection = database.GetCollection<BsonDocument>("my_collection"); var document = new BsonDocument { { "name", "John Doe" }, { "age", 30 }, { "email", "johndoe@example.com" } }; await collection.InsertOneAsync(document);
Read: Reading documents from a NoSQL database is straightforward. For example, to retrieve a document from a MongoDB collection:
var collection = database.GetCollection<BsonDocument>("my_collection"); var filter = Builders<BsonDocument>.Filter.Eq("name", "John Doe"); var document = await collection.Find(filter).FirstOrDefaultAsync();
Update: Updating a document in a NoSQL database can be accomplished through the UpdateOne method in the MongoDB driver:
var collection = database.GetCollection<BsonDocument>("my_collection"); var filter = Builders<BsonDocument>.Filter.Eq("name", "John Doe"); var update = Builders<BsonDocument>.Update.Set("age", 31); await collection.UpdateOneAsync(filter, update);
Delete: Finally, deleting documents is as simple as deleting files. Here is how to delete a document from a MongoDB collection:
var collection = database.GetCollection<BsonDocument>("my_collection"); var filter = Builders<BsonDocument>.Filter.Eq("name", "John Doe"); await collection.DeleteOneAsync(filter);
Querying NoSQL Data
Querying data in NoSQL is different from a traditional relational database. NoSQL databases use their own query languages to retrieve data, which can vary between database models.
For example, MongoDB uses a JSON-based query language. Here is an example of a query that finds all documents in a collection where the age field is greater than 25:
var collection = database.GetCollection<BsonDocument>("my_collection"); var filter = Builders<BsonDocument>.Filter.Gt("age", 25); var documents = await collection.Find(filter).ToListAsync();
When working with NoSQL databases, it’s important to understand the query language and syntax for the specific database model you’re using.
In conclusion, working with NoSQL data in .NET applications involves using the appropriate methods and query languages specific to the NoSQL database being used. By understanding how to perform CRUD operations and queries, developers can effectively manage data in NoSQL databases.
Leveraging the Power of NoSQL in .NET
Once you’ve decided to incorporate a NoSQL database in your .NET project, it’s important to understand how to make the most of its unique features to improve scalability and performance optimization. Here are some strategies to consider:
Denormalization
Denormalization is the process of adding redundant data to a NoSQL database for faster access. By duplicating data across multiple tables or documents, you can avoid expensive join operations and speed up queries. For example, consider a document database that stores information about customers and their orders. In a traditional relational database, you might have a separate table for customers and orders, with a foreign key relationship linking the two. But in a document database, you could store all of the customer’s order information within the customer document itself. This way, when you query for a specific customer’s information, you can retrieve all of their order data in a single query without joining multiple tables.
Here’s an example of denormalization in MongoDB using C#:
// Customer document with embedded order information
{
“_id”: ObjectId(“1234”),
“name”: “John Smith”,
“orders”: [
{ “order_id”: 1, “total”: 100 },
{ “order_id”: 2, “total”: 200 }
]
}
// Query for customer with order information
var customer = db.Customers.FindOne( Query.EQ(“_id”, ObjectId(“1234”)));
Sharding
Sharding is the process of distributing data across multiple servers to improve scalability. NoSQL databases are designed to handle large amounts of data, but as your data grows, a single server may not be able to handle the load. By sharding your data, you can distribute it across multiple servers, allowing you to scale horizontally. Each server only handles a portion of the data, reducing the load on each individual server and improving overall performance.
Here’s an example of sharding in MongoDB using C#:
// Enable sharding on a database
sh.enableSharding(“mydb”);
// Create a shard key for the collection
sh.shardCollection(“mydb.mycol”, {“_id”: “hashed”});
Performance Optimization
When working with a NoSQL database, it’s important to optimize your queries to improve performance. NoSQL databases use a different data model than traditional relational databases, so it’s important to understand how to work with non-SQL data. For example, NoSQL databases typically use document or key-value stores instead of tables and rows. This means you’ll need to use different query techniques and indexing strategies to get the best performance.
Here’s an example of using indexes in MongoDB using C#:
// Create an index on the “name” field
db.Customers.EnsureIndex(“name”);
// Query for customers with a specific name
var customers = db.Customers.Find( Query.EQ(“name”, “John Smith”));
By leveraging denormalization, sharding, and performance optimization techniques, you can maximize the benefits of NoSQL databases in your .NET projects.
Considerations for Using NoSQL with .NET
When using NoSQL databases with .NET, there are several considerations that developers should keep in mind. These considerations can impact application design and development decisions, so it’s essential to understand them before integrating NoSQL databases into your projects.
Data Consistency
NoSQL databases often prioritize performance over strict data consistency, which can lead to data inconsistencies in certain scenarios. For example, in a distributed database environment, it’s challenging to maintain strict consistency across all nodes. As a result, developers must consider whether strict consistency is necessary for their project.
Transaction Support
Transaction support in NoSQL databases varies widely depending on the database type and implementation. Some NoSQL databases offer full ACID-compliant transaction support, while others offer only eventual consistency. Developers must understand the level of transaction support provided by the NoSQL database they’re using and adjust their application design accordingly.
Schema Flexibility
NoSQL databases offer greater schema flexibility than traditional relational databases, which can be advantageous in certain scenarios. However, this flexibility can also make it challenging to maintain data quality and consistency. Developers must consider the tradeoffs of schema flexibility when deciding whether to use NoSQL databases in their projects.
Data Modeling
Data modeling in NoSQL databases can be significantly different from traditional relational databases. Developers must understand the data modeling requirements and principles of their chosen NoSQL database and adjust their data modeling approach accordingly. For example, some NoSQL databases use denormalization as a technique to improve performance, which can impact data modeling decisions.
Real-World Examples of NoSQL in .NET Projects
NoSQL databases are gaining popularity in the .NET community due to their scalability, flexibility, and performance. Many industries have already leveraged the power of NoSQL databases in their .NET projects. In this section, we will showcase some real-world examples of NoSQL being used in .NET projects and highlight industry trends in this field.
NoSQL in eCommerce
Ecommerce websites require high scalability and fast response times to handle a large volume of traffic and transactions. NoSQL databases like MongoDB and Cassandra are utilized to store product catalogs, user profiles, and transactional data. They allow for fast data retrieval and offer horizontal scalability, ensuring that the website remains performant, even during peak traffic periods.
For example, Magento, a popular eCommerce platform, uses MongoDB as its default NoSQL database to store catalog and order information. The MongoDB driver for .NET provides seamless integration, making it easy for developers to use MongoDB in their .NET projects.
NoSQL in Healthcare
The healthcare industry generates a vast amount of data, from patient records to medical research. NoSQL databases like Couchbase and Riak are utilized to store and manage this data effectively. They offer high availability and fault tolerance, ensuring that the data remains accessible at all times.
For example, the Center for Disease Control and Prevention (CDC) uses Couchbase as its NoSQL database for its disease tracking system. Couchbase’s built-in caching mechanism allows for fast data retrieval, making it ideal for the CDC’s real-time data tracking needs.
NoSQL in Gaming
Gaming applications require fast response times and efficient data storage for a seamless user experience. NoSQL databases like Redis and Neo4j are utilized to store game data such as user profiles, leaderboards, and game statistics. They allow for fast data retrieval and efficient data modeling, making them ideal for gaming applications.
For example, Xbox uses Redis as its NoSQL database for its gaming platform. Redis provides fast data retrieval, making it ideal for real-time gaming applications.
Industry Trends
The use of NoSQL databases in .NET projects is on the rise, and this trend is expected to continue. According to a survey conducted by JetBrains, MongoDB is the most popular NoSQL database among .NET developers, followed by Redis and Couchbase. Additionally, many NoSQL databases now offer .NET-specific drivers and libraries, making it easy for .NET developers to add NoSQL to their applications.
In conclusion, NoSQL databases offer many benefits in terms of scalability, performance, and flexibility, making them ideal for .NET projects across various industries. As more organizations adopt NoSQL, we can expect to see more innovative use cases and success stories in the future.
Future of NoSQL in .NET
NoSQL databases have rapidly gained popularity due to their scalability, flexibility, and performance optimization. With the rise of cloud computing and big data, NoSQL is becoming an increasingly attractive option for many businesses and developers to manage and store their data.
The future of NoSQL in the .NET ecosystem looks promising. As advancements in NoSQL technology continue to develop, integration with .NET frameworks and libraries will become even more seamless. Many experts predict that NoSQL will become the primary choice for data storage in the near future.
One of the important trends in NoSQL development is compatibility with other databases. Many NoSQL databases are now offering SQL compatibility, which enables developers to use SQL statements to query data stored in NoSQL databases. This trend is likely to continue in the future, making NoSQL databases more accessible and easier to use.
Another trend in NoSQL development is the rise of multi-model databases. These databases incorporate multiple data models, such as document databases, key-value stores, graph databases, and column-family stores, allowing developers to choose the optimal data model for their specific use case.
Industry research also indicates that NoSQL databases will continue to gain traction in different industries. For example, a recent study conducted by TechValidate found that 63% of surveyed organizations used NoSQL databases to handle their big data needs.
As NoSQL databases become more prevalent and popular, it’s essential for developers to stay up to date with the latest advancements and trends. This will enable them to make informed decisions on which NoSQL database to use and how to integrate it seamlessly with their .NET projects.
Leveraging the Power of NoSQL in .NET
Now that we’ve explored the benefits and considerations of using NoSQL with .NET applications, let’s dive into how to maximize those benefits. There are several strategies developers can use to improve scalability and performance optimization when working with NoSQL data.
Denormalization is a technique commonly used in NoSQL databases to optimize query performance. It involves duplicating data across multiple tables or documents to avoid costly joins. This can result in faster query times at the expense of increased storage space. Developers should carefully consider their data modeling and how denormalization can be used effectively.
Sharding is another technique that can improve scalability in distributed NoSQL databases. It involves distributing data across multiple servers based on a chosen shard key, with each server responsible for a subset of the data. This can greatly increase the throughput of read and write operations, but can also add complexity to the system and require careful planning.
When working with NoSQL data in .NET, it’s important to utilize the appropriate .NET frameworks and ORM libraries for the chosen NoSQL database. For example, if using a document database like MongoDB, the MongoDB .NET driver is a popular choice for interacting with the database. This ensures that developers are taking full advantage of the features and optimizations available through the database’s specific APIs.
By implementing these strategies and utilizing appropriate frameworks and libraries, developers can fully leverage the scalability and flexibility benefits of NoSQL databases in their .NET applications.
Let’s take a look at a code example for denormalization in MongoDB:
Normalized Data | Denormalized Data |
---|---|
Users:
Orders:
| Users:
|
In this example, normalized data is split into two tables (Users and Orders) with a foreign key connecting them. In the denormalized data, each user document includes an array of their orders. This can simplify queries for orders by user, at the cost of increased storage space.
Next, let’s take a look at a code example for sharding in MongoDB:
sh.enableSharding("mydatabase")
sh.shardCollection("mydatabase.mycollection", { "shardKey": 1 })
In this example, the shardCollection
command is used to start sharding on the mydatabase.mycollection collection, using the shardKey
field as the shard key.
In summary, by using denormalization, sharding, appropriate frameworks and libraries, and other scalability and performance optimization techniques, developers can effectively leverage the power of NoSQL databases in their .NET applications.
Now that we’ve explored the practical aspects of using NoSQL with .NET, let’s take a look at some real-world examples and industry trends.
Additional Resources
Now that you have a better understanding of how NoSQL databases can be incorporated into .NET projects, it’s time to explore further resources and deepen your knowledge. Below are some recommendations for books, articles, websites, and .NET libraries and frameworks related to NoSQL.
Books
1. Seven Databases in Seven Weeks: A Guide to Modern Databases and the NoSQL Movement by Luc Perkins, Jim Wilson, and Eric Redmond
2. NoSQL Distilled: A Brief Guide to the Emerging World of Polyglot Persistence by Martin Fowler and Pramod Sadalage
3. Learning NoSQL: A Beginner’s Guide to Building Efficient and High-Performance Non-Relational Databases by Gaurav Vaish
Articles and Websites
1. NoSQL.org – an online community for NoSQL enthusiasts, featuring news, articles, and resources
2. MongoDB blog – a blog dedicated to MongoDB, a popular document-based NoSQL database
3. Couchbase blog – a blog dedicated to Couchbase, a NoSQL database that combines key-value and document-oriented data models
.NET Libraries and Frameworks
1. RavenDB – a document-based NoSQL database that works seamlessly with .NET frameworks such as ASP.NET and Entity Framework
2. Couchbase .NET SDK – a .NET library for working with Couchbase NoSQL databases
3. Redisson – a Redis-based NoSQL data access framework for .NET, allowing for distributed caching, and more
By exploring these resources, you can gain invaluable insights into the world of NoSQL and deepen your understanding of how it can be leveraged with .NET in your projects.
FAQ
Q: How can I use NoSQL with .NET?
A: You can use NoSQL with .NET by integrating NoSQL databases into your .NET projects. This involves choosing the appropriate NoSQL database option and configuring it to work with .NET frameworks and ORM libraries.
Q: What are the benefits of using NoSQL with .NET?
A: Using NoSQL with .NET can provide efficient data storage, scalability, and flexibility in your applications. It allows you to leverage the power of NoSQL databases for improved performance and handling of large amounts of data.
Q: What are the considerations when using NoSQL with .NET?
A: When using NoSQL with .NET, you should consider data consistency, transaction support, schema flexibility, and data modeling. These factors can impact your application design and development decisions.
Q: What are the types of NoSQL databases available for .NET?
A: The types of NoSQL databases available for .NET include document databases, key-value stores, column-family stores, and graph databases. Each type has its characteristics and use cases, allowing you to choose the most suitable option for your .NET projects.
Q: How do I set up NoSQL databases in .NET?
A: To set up NoSQL databases in .NET, you need to choose the appropriate .NET frameworks and ORM libraries and configure them to work with your selected NoSQL database. Code examples and best practices can help you in the process.
Q: How do I work with NoSQL data in .NET?
A: To work with NoSQL data in .NET, you need to perform CRUD operations (create, read, update, delete) and use querying techniques specific to NoSQL databases. Relevant .NET code examples can guide you in working with NoSQL data.
Q: How can I leverage the power of NoSQL in .NET?
A: You can maximize the benefits of NoSQL in .NET by focusing on scalability and performance optimization. Techniques such as denormalization and sharding can help improve the performance of your NoSQL-enabled .NET applications.
Q: What are some real-world examples of NoSQL in .NET projects?
A: Real-world examples of NoSQL in .NET projects showcase how NoSQL databases are being used in various industries. These examples highlight the practical applications and benefits of NoSQL in .NET development.
Q: What does the future hold for NoSQL in .NET?
A: The future of NoSQL in .NET is marked by advancements, trends, and compatibility. As technology evolves, NoSQL will continue to play a crucial role in the .NET ecosystem, enabling developers to build scalable and efficient applications.
Q: Where can I find additional resources on NoSQL in .NET?
A: For further exploration, we recommend checking out NoSQL-related books, articles, websites, and relevant .NET libraries and frameworks. These resources can help you deepen your understanding of NoSQL and its integration with .NET.
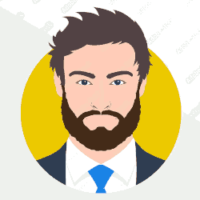
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.