Testing is a crucial part of .NET Core development and is essential for ensuring code quality. As a .NET Core developer, it is important to understand the strategies and techniques that can be used to effectively test applications.
So, let’s dive into the world of testing strategies for .NET Core developers. Discover how to code test in .NET Core, and ensure code quality with these strategies for testing .NET Core applications.
Importance of Testing in .NET Core Development
Testing is an essential part of developing .NET Core applications. It helps ensure that the code works as intended and meets the required specifications. Testing also plays a crucial role in maintaining code quality, reducing the likelihood of errors, and ensuring that applications are reliable and secure.
Without proper testing, developers run the risk of deploying code that may be plagued with bugs and other issues. This can lead to performance problems, security vulnerabilities, and other issues that can compromise the overall functionality of the application.
Fortunately, there are many strategies that .NET Core developers can employ to ensure that their applications are thoroughly tested. By following best practices and utilizing different testing techniques, developers can increase code quality and reduce the likelihood of errors in production.
Testing Strategies for .NET Core Applications
When it comes to testing .NET Core applications, there are several strategies that developers can employ to ensure code quality and application functionality. These strategies include:
- Unit testing: This strategy involves testing individual units of code in isolation to ensure that they work as intended. By testing code at the unit level, developers can quickly identify bugs and ensure that each unit functions correctly before they are combined into larger components.
- Integration testing: This strategy involves testing how different components of an application work together to ensure that they integrate correctly. By performing integration testing, developers can identify any issues that may arise when components are combined and ensure that each component works as intended when integrated with others.
- End-to-end testing: This strategy involves testing an application’s full functionality to ensure that it works as intended from start to finish. By performing end-to-end testing, developers can identify any issues that may not be caught by unit testing or integration testing and ensure that the application performs as expected in real-world scenarios.
Each of these testing strategies has its own benefits and use cases, and it is up to the developer to determine which strategy is best suited for their application and testing needs.
It is important to note that testing should not be seen as a one-time activity. Instead, developers should aim to integrate testing into their development process, continuously testing their code throughout the development cycle to ensure that it remains bug-free and functional.
Unit Testing Techniques for .NET Core
Unit testing is an essential component of ensuring code quality in .NET Core development. This testing technique involves testing individual units or components of code to ensure that they operate as intended.
One popular framework for unit testing in .NET Core is NUnit.
Let’s take a look at an example of how to write a unit test using NUnit:
Example:
[Test]
public void TestAddition()
{
//Arrange
int a = 5;
int b = 10;
//Act
int result = Calculator.Add(a, b);
//Assert
Assert.AreEqual(15, result);
}
In the example above, we define a test method called “TestAddition.” The [Test] attribute marks this method as a unit test to be run by NUnit.
The //Arrange section sets up the initial values for the variables a and b. The //Act section performs the addition operation using the Calculator.Add() method. Finally, the //Assert section checks whether the result of the operation matches the expected value using the Assert.AreEqual() method.
When running this test, NUnit will automatically generate a test report indicating whether the test passed or failed.
In addition to NUnit, another popular framework for unit testing in .NET Core is xUnit. The syntax for writing tests in xUnit is slightly different, but the concept is the same.
When writing unit tests, it’s important to follow best practices for organization and execution.
For example, you should group related tests into test suites and run them automatically as part of your software development process.
This ensures that your code is thoroughly tested and that bugs are caught early in the development cycle.
Integration Testing in .NET Core
Integration testing is an essential strategy for ensuring code quality in .NET Core applications. It involves testing the interaction between various components of an application to ensure that they work together seamlessly.
One popular framework for integration testing in .NET Core is NUnit, which allows developers to write tests that simulate how individual components will behave when integrated with the rest of the application. NUnit can be used to test various scenarios, such as database interactions, API calls, and user interface events.
When performing integration testing in .NET Core, it’s important to ensure that all dependencies are properly configured and that the environment is set up correctly. This can be achieved by using tools like Docker and Kubernetes, which help create consistent testing environments.
Integration testing can be challenging, but it remains an integral part of the testing process in .NET Core development. By thoroughly testing the interaction between various components, developers can ensure that their application works as intended.
Challenges of Integration Testing in .NET Core
One of the main challenges of integration testing in .NET Core is the complexity of the application. As applications become more complex, the number of components that need to be tested increases, and this can make integration testing a time-consuming and difficult process.
Another challenge of integration testing is ensuring that all components are properly configured. If even one component is misconfigured, the entire test can fail, making it difficult to pinpoint the source of the problem.
Despite these challenges, integration testing remains a crucial part of the testing process in .NET Core development. By testing the interaction between various components, developers can ensure that their application works as intended and that any bugs are caught early in the development process.
End-to-End Testing in .NET Core
End-to-end (E2E) testing is a comprehensive testing approach that evaluates the entire system’s functionality from end-to-end. It involves testing the application’s workflow, data flow, and integration with other systems. E2E testing simulates real-world scenarios to ensure that the application behaves as expected.
E2E testing is essential to detect critical issues, such as data loss, data corruption, and system crashes, that may occur due to the application’s complex interactions. It also helps ensure that the application meets the user’s requirements and expectations.
In .NET Core, E2E testing can be conducted using frameworks like Selenium or Cypress. These frameworks simulate user actions, such as clicking buttons, filling forms, and navigating through pages, to test the application’s workflow and UI.
Here’s an example of how to write an E2E test for a .NET Core application using Selenium:
// Navigate to the application URL
driver.Navigate().GoToUrl("https://myapp.com");
// Find the username and password input fields
var usernameField = driver.FindElement(By.Id("username"));
var passwordField = driver.FindElement(By.Id("password"));
// Enter the username and password values
usernameField.SendKeys("myusername");
passwordField.SendKeys("mypassword");
// Find and click the login button
var loginButton = driver.FindElement(By.Id("loginButton"));
loginButton.Click();
// Verify that the user is redirected to the dashboard page
Assert.AreEqual("https://myapp.com/dashboard", driver.Url);
E2E testing can be time-consuming and resource-intensive, as it requires setting up a testing environment that simulates the production environment, including databases, servers, and other systems. However, it is a crucial step in ensuring the application’s quality and reliability.
Code Coverage and Code Quality in .NET Core
Code coverage is an essential metric to ensure code quality in .NET Core development. It measures the amount of code that is executed during testing and gives insights into the parts of the code that have not been tested yet. Code coverage tools such as dotCover, Visual Studio, or JetBrains ReSharper are commonly used to measure code coverage in .NET Core applications.
However, code coverage should not be the only metric for measuring code quality. It is possible to achieve high code coverage while still having poor quality code. To improve code quality, developers should employ best practices such as adhering to coding standards, writing clean and readable code, and performing code reviews.
Moreover, integrating code quality checks into the CI/CD pipeline can automate the process of ensuring code quality. Tools like SonarQube, Code Climate, or ReSharper offer features such as static code analysis, code smells detection and metrics visualization to improve code quality in .NET Core applications.
Automated Testing in .NET Core
Automated testing is a vital strategy for any .NET Core developer looking to ensure code quality and minimize errors in production. With automated testing, developers can write scripts to automatically test their code and quickly identify any issues or bugs.
The most commonly used automated testing frameworks in .NET Core are NUnit, xUnit, and Azure DevOps. These frameworks allow developers to write tests for their code and run them automatically, without the need for manual intervention.
One of the key benefits of automated testing is its ability to save time and increase efficiency. By automating the testing process, developers can run tests much faster and more frequently than if they were done manually. This means that issues can be detected and fixed much earlier in the development cycle, reducing the time and effort required for debugging and troubleshooting.
When implementing automated testing in .NET Core, it’s essential to follow best practices such as separating test code from production code, using clear and concise test names, and ensuring that the tests are repeatable and independent of each other.
Overall, automated testing is an essential strategy for any .NET Core developer looking to ensure code quality and minimize errors in production. By incorporating automated testing into their development process, developers can save time and reduce the risk of errors and bugs, resulting in a more robust and reliable application.
Continuous Integration and Testing in .NET Core
Continuous integration (CI) is a practice that involves regularly integrating code changes into a shared repository and constantly testing these changes to detect and resolve issues early on in the development process. By incorporating testing into a CI process, .NET Core developers can ensure that their code meets quality standards and is stable.
To set up a CI pipeline with .NET Core, there are various tools and services available, including Jenkins, Travis CI, and Azure Pipelines. The process usually involves the following steps:
- Defining a build environment with the necessary dependencies
- Compiling the code and generating artifacts
- Running tests against the code and generating test reports
- Deploying the code to a staging environment for testing and quality assurance
- Deploying the code to production once it passes all tests and quality checks
By automating the testing process through a CI pipeline, .NET Core developers can detect and resolve issues early, which is crucial in ensuring code quality and reducing development cycle time.
One of the main benefits of incorporating testing into a CI process is that it helps developers catch issues early on, when they are easier to fix. This reduces the likelihood of bugs making it to production and potentially causing issues for end-users.
Additionally, by automating the testing process, .NET Core developers can save time and resources that would otherwise be spent on manual testing, and focus on delivering new features and improvements instead.
Overall, incorporating testing into a continuous integration process is an effective way for .NET Core developers to ensure code quality and stability.
By taking advantage of the various tools and services available, developers can easily set up a CI pipeline and automate their testing process, saving time and resources and delivering better software.
Performance Testing in .NET Core
Performance testing is a critical aspect of ensuring the quality of .NET Core applications. It involves measuring how well the application performs under various loads, identifying potential bottlenecks, and optimizing the code to improve performance.
There are several strategies for conducting performance testing in .NET Core applications. One approach is to use load testing tools like Apache JMeter or Visual Studio Load Testing to simulate real-world user traffic and measure the application’s response time. Another approach is to use profiling tools like dotTrace or ANTS Performance Profiler to identify performance bottlenecks in the code.
When conducting performance testing, it’s important to define the performance metrics that are most relevant to your application. These could include factors like response time, CPU usage, memory usage, and throughput. By measuring these metrics, you can identify areas where the application can be optimized to improve performance.
One common performance-related issue in .NET Core applications is inefficient database queries. By optimizing database queries and using caching techniques, you can significantly improve the performance of your application.
Ultimately, the goal of performance testing is to ensure that your application performs well under heavy load and provides a good user experience. By incorporating performance testing into your testing strategy, you can identify and address performance issues before they impact your users.
Security Testing in .NET Core
Ensuring the security of .NET Core applications is critical in today’s digital landscape. Cyber attacks and data breaches are becoming more frequent, and developers must take proactive steps to protect their applications. Security testing involves identifying vulnerabilities in an application’s code and verifying that security mechanisms are functioning as expected.
One of the most common security vulnerabilities in .NET Core applications is cross-site scripting (XSS). This vulnerability enables an attacker to inject malicious code into a web page, potentially compromising user data. To test for XSS vulnerabilities, developers can use tools such as OWASP’s Zed Attack Proxy (ZAP) or Microsoft’s Security Code Scan.
Another common vulnerability is SQL injection (SQLi), which occurs when an attacker inserts malicious SQL queries into an application’s input fields. To test for SQLi vulnerabilities, developers can use tools such as SQLMap or OWASP’s SQL Injection Testing Tool.
Additionally, developers should perform regular vulnerability scans using tools such as Nessus or OpenVAS to identify potential security issues. They should also follow security best practices, such as implementing secure authentication and authorization mechanisms, encrypting sensitive data, and maintaining up-to-date software and security patches.
Overall, security testing should be an integral part of the testing process for .NET Core applications. By identifying and addressing vulnerabilities, developers can ensure that their applications are secure and protect users’ data.
Final Thoughts
Testing is a critical aspect of .NET Core development and is essential for ensuring code quality and reducing the likelihood of errors in production. By employing effective testing strategies and techniques, .NET Core developers can identify bugs early on and optimize the performance and security of their applications.
For .NET Core developers, it is essential to prioritize testing at every stage of the development process. By writing high-quality unit tests, integration tests, and end-to-end tests, developers can catch potential issues before they become real problems.
Moreover, automated testing, code coverage analysis, and continuous integration can streamline the testing process and ensure that applications are robust, performant, and secure.
External Resources
https://en.wikipedia.org/wiki/Unit_testing
https://en.wikipedia.org/wiki/Integration_testing
FAQ
1. How do I write a basic unit test in .NET Core?
Code Sample:
using System;
using Xunit;
namespace MyProject.Tests
{
public class BasicTests
{
[Fact]
public void TestAddition()
{
// Arrange
int a = 5;
int b = 3;
// Act
int result = a + b;
// Assert
Assert.Equal(8, result);
}
}
}
This sample uses xUnit
, a popular testing framework for .NET Core. The Fact
attribute denotes a basic test that does not take parameters.
2. How can I test a method that throws an exception in .NET Core?
Code Sample:
using System;
using Xunit;
namespace MyProject.Tests
{
public class ExceptionTests
{
[Fact]
public void TestForException()
{
// Arrange
var myObject = new MyObject();
// Act & Assert
Assert.Throws<InvalidOperationException>(
() => myObject.MethodThatThrows());
}
}
}
This test checks if the specified method throws an InvalidOperationException
.
3. How do I write a data-driven test using InlineData
in .NET Core?
Code Sample:
using Xunit;
namespace MyProject.Tests
{
public class DataDrivenTests
{
[Theory]
[InlineData(5, 5, 10)]
[InlineData(3, -3, 0)]
public void TestAddition(int a, int b, int expected)
{
// Act
int result = a + b;
// Assert
Assert.Equal(expected, result);
}
}
}
The Theory
attribute indicates a data-driven test. InlineData
provides different sets of data for the test.
4. How do I mock dependencies in .NET Core unit tests?
Code Sample:
using Moq;
using Xunit;
using MyProject;
namespace MyProject.Tests
{
public class MockTests
{
[Fact]
public void TestMethodWithDependency()
{
// Arrange
var dependencyMock = new Mock<IMyDependency>();
dependencyMock.Setup(d => d.GetValue()).Returns(5);
var myObject = new MyClass(dependencyMock.Object);
// Act
int result = myObject.UseDependency();
// Assert
Assert.Equal(5, result);
}
}
}
This sample uses Moq
to mock an interface IMyDependency
. It’s useful for isolating tests from external dependencies.
5. How can I test asynchronous code in .NET Core?
Code Sample:
using System.Threading.Tasks;
using Xunit;
namespace MyProject.Tests
{
public class AsyncTests
{
[Fact]
public async Task TestAsyncMethod()
{
// Arrange
var myObject = new MyAsyncClass();
// Act
var result = await myObject.GetAsyncValue();
// Assert
Assert.Equal("ExpectedValue", result);
}
}
}
This test demonstrates how to write a unit test for asynchronous methods using async
and await
.
These examples cover various aspects of testing in .NET Core, from basic unit tests to more advanced scenarios involving exceptions, data-driven testing, mocking, and asynchronous code. Remember to install the necessary packages like xUnit
, Moq
, or others depending on your testing needs.
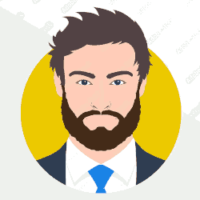
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.