As mobile app development continues to grow, so does the need to enhance the functionality of these apps. .NET APIs provide a powerful solution to this challenge, offering a wide range of tools and features that can be leveraged to create robust and user-friendly mobile apps.
Are .NET APIs used with Android and iOS apps? The answer is yes In fact, many popular mobile apps today use .NET APIs to enhance their functionality and improve user experience.
.NET APIs for Mobile App Development
When it comes to developing mobile apps, there are various tools and frameworks that developers can use to create robust and functional applications. One such tool is .NET APIs. .NET APIs are a set of programming interfaces that developers can use to enhance the functionality of their mobile apps.
For those unfamiliar with .NET, it is a free, open-source, cross-platform development framework that allows developers to create apps for a wide range of platforms, including Windows, Android, and iOS. .NET provides a variety of APIs that developers can use to build different types of applications, including web, desktop, and mobile apps.
When it comes to mobile app development, .NET APIs are particularly useful because they provide an easy way to access and manipulate data. For example, .NET APIs can be used to retrieve data from external sources, such as a web service or a database, or to manipulate data within the app. Using .NET APIs, developers can create powerful and efficient mobile apps that meet their users’ needs.
Examples of .NET APIs for Mobile App Development
There are various .NET APIs that developers can use in mobile app development, depending on the specific needs of their app. Here are some examples:
- HttpClient: This API enables developers to send HTTP requests and receive HTTP responses from a web service. It provides an easy way to access external data sources and incorporate them into the app.
- XmlSerializer: This API allows developers to convert XML data to .NET objects and vice versa, making it easier to manipulate the data within the app.
- DataContractJsonSerializer: This API enables developers to serialize and deserialize JSON data, which is a common format used by web services. It simplifies the process of integrating external data sources into the app.
These are just a few examples of the many .NET APIs available for mobile app development. By using these APIs, developers can simplify coding and improve the performance and functionality of their apps.
Compatibility of .NET APIs with Android and iOS
One of the advantages of .NET APIs is their compatibility with both Android and iOS platforms. While each platform has its own unique features and requirements, .NET APIs can seamlessly integrate with both.
When integrating .NET APIs in Android development, developers can take advantage of the Android Native Development Kit (NDK) to access native libraries and achieve better performance. The following code example demonstrates how to use .NET APIs in an Android app:
// Import the necessary packages
using System;
using Android.App;
using Android.Widget;
using Android.OS;
namespace MyAndroidApp
{
[Activity(Label = "My App", MainLauncher = true)]
public class MainActivity : Activity
{
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
// Set our view from the "main" layout resource
SetContentView(Resource.Layout.Main);
// Use a .NET API to display a message
Console.WriteLine("Hello World!");
}
}
}
On the other hand, when integrating .NET APIs in iOS development, developers can use Xamarin.iOS to create native iOS apps with C#. The following code example demonstrates how to use .NET APIs in an iOS app:
// Import the necessary packages
using System;
using Foundation;
using UIKit;
namespace MyiOSApp
{
public partial class ViewController : UIViewController
{
public ViewController(IntPtr handle) : base(handle)
{
}
public override void ViewDidLoad()
{
base.ViewDidLoad();
// Use a .NET API to display a message
Console.WriteLine("Hello World!");
}
}
}
While there may be some differences in integrating .NET APIs in Android and iOS, the compatibility of .NET APIs with both platforms allows developers to take advantage of the strengths of each platform and create high-quality mobile apps.
Benefits of Using .NET APIs in Android App Development
Integrating .NET APIs in Android app development has been gaining popularity among developers due to its numerous benefits. Let’s take a closer look at some of the major advantages of using .NET APIs:
- Improved performance: .NET APIs are designed to optimize the performance of mobile apps, resulting in faster load times and smoother operation. By utilizing features such as just-in-time compilation and native interoperability, .NET APIs can significantly enhance the overall performance of Android apps.
- Simplified coding: .NET APIs offer a simple yet powerful programming model that enables developers to write clean, concise code. This results in faster development times, fewer bugs, and easier maintenance of the app codebase.
- Enhanced user experience: .NET APIs provide developers with a rich set of tools and features that can be leveraged to create engaging and interactive user interfaces. From animations and graphics to input handling and touch gestures, .NET APIs offer a wide range of options for enhancing the user experience.
Let’s take a look at how we can implement these benefits in our Android app development code:
// Improved performance: load data asynchronously
using System.Threading.Tasks;
public async Task LoadDataAsync()
{
// Load data from server
var data = await GetDataFromServer();
// Display data in UI
DisplayData(data);
}
// Simplified coding: using LINQ
var items = new List<int>() { 1, 2, 3, 4, 5 };
var even = items.Where(i => i % 2 == 0);
// Enhanced user experience: using animations
var anim = ObjectAnimator.OfFloat(button, "alpha", 0f, 1f);
anim.SetDuration(1000);
anim.Start();
By utilizing these features, Android developers can create high-performance, feature-rich apps that deliver an excellent user experience. Enhancing iOS Apps with .NET APIs
iOS app development can be challenging, with a need to constantly balance performance, user experience, and a wide range of features. .NET APIs can simplify this process and enhance iOS app functionality, providing developers with a powerful tool to create engaging user experiences.
One of the most significant advantages of using .NET APIs in iOS app development is the ability to leverage pre-existing code. This means that developers can easily integrate third-party libraries and frameworks into their iOS apps, without needing to recreate functionalities from scratch.
Additionally, .NET APIs are well-suited for handling complex data and business logic, making them a valuable resource for managing and manipulating large amounts of data within iOS apps.
Developers using .NET APIs can also take advantage of the numerous features that they offer, including advanced security features, cross-platform compatibility, and support for a wide range of programming languages.
For example, using .NET APIs in iOS app development can allow developers to implement secure data handling features such as encryption and decryption, as well as integrate with other Microsoft technologies like Azure cloud services.
.NET APIs are also designed to be highly performant, which can help to enhance the overall user experience of iOS apps.
By leveraging the .NET framework, developers can ensure that their apps are optimized for speed and responsiveness, which can be particularly important for apps that require real-time data updates or processing.
.NET APIs are well-suited for cross-platform app development, making it easier to create apps that can run on multiple operating systems. By using frameworks like Xamarin, developers can use .NET APIs to develop iOS apps that can run on both iOS and Android devices, maintaining a consistent user experience across different platforms.
Code Examples
When developing iOS apps with .NET APIs, developers will typically use a combination of Swift or Objective-C, along with the .NET runtime. For example, the following code snippet shows a simple Swift function that uses .NET APIs to access a database:
// Use .NET APIs to connect to a database
let connString = "Server=myServerName;Database=myDataBase;User Id=myUsername;Password=myPassword;"
let connection = SqlConnection(connString)
connection.Open()
// Execute a SQL query using .NET APIs
let query = "SELECT * FROM Customers"
let command = SqlCommand(query, connection)
// Retrieve data from the query result using .NET APIs
let reader = command.ExecuteReader()
while reader.Read() {
let firstName = reader["FirstName"] as String
let lastName = reader["LastName"] as String
}
// Clean up resources using .NET APIs
reader.Close()
command.Dispose()
connection.Close()
This code demonstrates how .NET APIs can be used to establish a connection to a SQL database, execute a query, and retrieve the results. By using .NET APIs, developers can simplify this process and take advantage of the advanced functionality that the framework provides.
Cross-Platform Mobile App Development with .NET APIs
Mobile app development has become a rapidly evolving industry, with the emergence of cross-platform frameworks and tools providing developers with new opportunities to create high-quality apps. .NET APIs offer a powerful solution for cross-platform mobile app development, allowing developers to leverage the capabilities of .NET across multiple platforms.
A key advantage of .NET APIs is the ability to write code once and deploy it across multiple platforms, such as iOS and Android. The Xamarin framework enables developers to create native apps for these platforms using C# and .NET APIs, providing a streamlined development process.
Comparing .NET APIs with Other Cross-Platform Frameworks
When comparing .NET APIs with other cross-platform frameworks, such as React Native and Flutter, it’s important to consider the specific features and capabilities of each. For example, React Native offers a flexible component-based architecture, while Flutter provides a highly customizable and performant user interface.
However, .NET offers a unique advantage in its compatibility with existing .NET libraries and frameworks, enabling developers to leverage existing code and tools. Additionally, .NET provides a stable and secure development environment, with a large community of developers and resources for support.
Using Visual Studio for Cross-Platform Development
Visual Studio is a popular integrated development environment (IDE) for .NET developers, and offers powerful features for cross-platform mobile app development. With Visual Studio, developers can use Xamarin to create native apps for iOS, Android, and Windows platforms, using a single codebase.
Visual Studio also provides a rich set of debugging and testing tools, enabling developers to quickly identify and resolve issues. Additionally, Visual Studio integrates with other Microsoft tools and services, such as Azure, providing a seamless development and deployment experience.
Best Practices for Integrating .NET APIs in Mobile Apps
Integrating .NET APIs in mobile apps can significantly enhance their functionality and performance. However, to ensure a seamless integration, it is important to follow best practices and industry standards. Here are some best practices for integrating .NET APIs in mobile apps:
Code Organization
When integrating .NET APIs, it is essential to organize the code in a structured and readable manner. Use meaningful names for variables, classes and functions, and separate the code into logical modules. This can improve the maintainability, readability, and scalability of the codebase.
Example: In a shopping app, instead of having all the code related to payment processing in a single file, separate it into multiple files based on functionality, such as “PaymentGateway.cs”, “CouponProcessor.cs”, and “TransactionManager.cs”.
Error Handling
Error handling is crucial when integrating .NET APIs in mobile apps. Always anticipate potential errors and handle them gracefully. Good error handling can improve app stability, prevent crashes and protect user data. Use try-catch blocks to catch exceptions and provide meaningful error messages to the user.
Example: If the app fails to connect to a server, instead of displaying a generic “Server Error” message, provide a detailed message that informs the user about the nature of the error and suggests possible solutions.
Performance Optimization
Performance optimization is essential to ensure that the app performs efficiently and meets user expectations. Use asynchronous programming to avoid blocking the main thread, minimize network requests, and optimize memory usage. Moreover, use caching to reduce data retrieval time and optimize rendering performance.
Example: Use the Task Parallel Library (TPL) to perform long-running or I/O-bound operations in the background, without blocking the UI thread. Use Response Caching and Image Caching to reduce the number of network requests and improve app performance.
Security Considerations
Security is a critical aspect of mobile app development. When integrating .NET APIs, it is important to ensure that the app is secure from potential threats such as data breaches, unauthorized access, and injection attacks. It is recommended to use encryption, secure authentication, and input validation to enhance app security.
Example: Use HTTPS encryption to secure communication between the app and the server. Use secure authentication protocols such as OAuth or OpenID Connect to authenticate users and protect their data. Use input validation to prevent injection attacks and ensure that only valid data is accepted.
By following these best practices, developers can create high-quality mobile apps that integrate .NET APIs seamlessly and efficiently. These practices not only improve app functionality and performance but also contribute to better user experience and security.
Final Thoughts
Integrating .NET APIs in mobile app development can greatly enhance the functionality and performance of Android and iOS apps. By leveraging the capabilities of .NET APIs, developers can create powerful and feature-rich mobile applications.
External Resources
https://learn.microsoft.com/en-us/dotnet/api/system.xml.serialization.xmlserializer
https://learn.microsoft.com/en-us/dotnet/api/system.net.http.httpclient
FAQ
The use of .NET APIs in conjunction with Android and iOS apps is a common scenario, especially when building cross-platform mobile applications. Here are five frequently asked questions about this topic, each with a relevant code sample:
1. Can I consume a .NET Web API in an Android app using Retrofit?
Code Sample (Android with Retrofit):
Android apps can consume .NET APIs using Retrofit, a type-safe HTTP client for Android and Java.
public interface MyApiService {
@GET("api/products")
Call<List<Product>> getAllProducts();
}
// Usage
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://yourapi.com/")
.addConverterFactory(GsonConverterFactory.create())
.build();
MyApiService apiService = retrofit.create(MyApiService.class);
apiService.getAllProducts().enqueue(new Callback<List<Product>>() {
@Override
public void onResponse(Call<List<Product>> call, Response<List<Product>> response) {
if(response.isSuccessful()) {
List<Product> products = response.body();
// Handle the products
}
}
@Override
public void onFailure(Call<List<Product>> call, Throwable t) {
// Handle failure
}
});
This code demonstrates how an Android app can retrieve data from a .NET Web API.
2. How do I use a .NET Web API in an iOS app with Alamofire?
Code Sample (iOS with Alamofire):
iOS apps can easily consume .NET APIs using Alamofire, a Swift-based HTTP networking library.
import Alamofire
// Define the endpoint
let url = "http://yourapi.com/api/products"
// Making a request
Alamofire.request(url).responseJSON { response in
switch response.result {
case .success(let value):
if let JSON = value as? [Any] {
// Handle the response
}
case .failure(let error):
print(error)
}
}
This snippet shows how to request data from a .NET API in an iOS application using Alamofire.
3. Is it possible to use .NET Web APIs with Xamarin for both Android and iOS apps?
Code Sample (Xamarin):
Xamarin.Forms, a cross-platform UI toolkit, can consume .NET Web APIs for both Android and iOS.
public class ProductService
{
HttpClient client = new HttpClient();
public async Task<List<Product>> GetProductsAsync()
{
string url = "http://yourapi.com/api/products";
var response = await client.GetAsync(url);
if(response.IsSuccessStatusCode)
{
var content = await response.Content.ReadAsStringAsync();
return JsonConvert.DeserializeObject<List<Product>>(content);
}
return new List<Product>();
}
}
This code can be used in a Xamarin.Forms project to fetch data from a .NET Web API, working across both Android and iOS.
4. Can React Native interface with .NET Web APIs for mobile app development?
Code Sample (React Native):
React Native, a framework for building native apps using React, can easily work with .NET Web APIs.
import axios from 'axios';
const fetchProducts = async () => {
try {
const response = await axios.get('http://yourapi.com/api/products');
console.log(response.data);
} catch (error) {
console.error(error);
}
};
// Call this function when needed
fetchProducts();
This example uses axios, a promise-based HTTP client, to consume a .NET Web API in a React Native application.
5. How do I handle authentication when using .NET Web APIs in mobile applications?
Code Sample (Using Token-based Authentication):
For secure communication between mobile apps and .NET Web APIs, token-based authentication is commonly used.
// .NET Web API Controller
[HttpPost("authenticate")]
public IActionResult Authenticate([FromBody] UserCredentials credentials)
{
var token = _authenticationService.Authenticate(credentials.Username, credentials.Password);
if (token == null)
return Unauthorized();
return Ok(token);
}
In your mobile app (Android, iOS, Xamarin, or React Native), you would then send a request to this endpoint to receive an authentication token, which should be included in subsequent API requests.
// Example in Android (Retrofit)
@POST("api/authenticate")
Call<Token> authenticate(@Body UserCredentials credentials);
This code shows how to implement a simple token-based authentication system between a .NET Web API and a mobile application.
These examples demonstrate how .NET Web APIs can be effectively used with Android and iOS apps, covering various platforms and scenarios including network requests, data handling, and authentication.
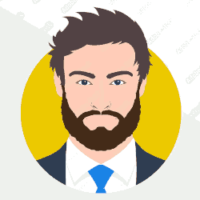
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.