What Skills to Look for in a .NET MVC Developer unveils the secret recipe to hiring the crème de la crème of .NET programmers.
When hiring a .NET MVC developer, there are key attributes and skills that you must consider. In this article, we’ll guide you through these skills to ensure you recruit top talent.
The Fundamentals
This section of what skills to look for in a .NET MVC Developer demystifies the skill set that truly distinguishes outstanding .NET talents.
First off, ASP.NET MVC knowledge is non-negotiable. A developer must exhibit a strong grasp of the ASP.NET MVC framework, as this serves as the backbone of .NET web application development.
Next in line is coding skills. Naturally, the ability to write clean, efficient code forms the crux of a developer’s role. Thus, a proficient .NET MVC developer should have a strong foundation in coding.
In addition to that, a solid understanding of programming languages is essential. Specifically, C# expertise is paramount, given that it’s the primary language used in .NET development.
First, let’s clarify some key terms:
- ASP.NET MVC: An open-source software from Microsoft that provides a web development framework combining the features of MVC (Model-View-Controller) architecture, the most up-to-date ideas and techniques from Agile development, and the best parts of the existing ASP.NET platform.
- C#: A modern, object-oriented programming language developed by Microsoft as part of their .NET initiative.
- HTML: Stands for HyperText Markup Language, it is the standard language used to create web pages.
- CSS: Cascading Style Sheets, is a style sheet language used for describing the look and formatting of a document written in HTML.
- JavaScript: An object-oriented computer programming language commonly used to create interactive effects within web browsers.
- jQuery: A fast, small, and feature-rich JavaScript library designed to simplify the client-side scripting of HTML.
- Bootstrap: A free and open-source CSS framework directed at responsive, mobile-first front-end web development.
- Database operations: These refer to the four basic operations of persistent storage: Create, Read, Update, and Delete (CRUD).
- Code Refactoring: The process of restructuring existing computer code without changing its external behavior for improving nonfunctional attributes of the software.
- Unit Testing: A level of software testing where individual units or components of a software are tested.
- Problem-solving skills: The ability to find solutions to problems using your creativity, reasoning, and past experiences along with the available information and resources.
1. What makes ASP.NET MVC knowledge fundamental for a .NET developer?
ASP.NET MVC is a popular framework for building scalable, standards-based web applications using well-established design patterns. It separates an application into three interconnected parts – Model, View, and Controller.
For example:
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
}
Here, HomeController
is a basic controller in ASP.NET MVC that returns a view. Without understanding these principles, a developer would struggle to create robust and organized .NET web applications.
Pro tip: Brush up on advanced ASP.NET MVC concepts like routing, filters, and custom model binders. They can significantly increase your development speed and efficiency.
2. Why are coding skills vital in .NET development?
Coding skills are essential as they form the base of any development task. This includes not only writing code but also understanding algorithms, data structures, and principles of good code organization.
For example:
public class Fibonacci
{
public int Calculate(int n)
{
if (n <= 1)
return n;
else
return Calculate(n - 1) + Calculate(n - 2);
}
}
This C# code is a simple implementation of the Fibonacci sequence. It exhibits a basic understanding of recursion, a fundamental coding concept.
Pro tip: Always follow the DRY (Don’t Repeat Yourself) principle. Aim to write reusable code to save time and effort, and to make the code easier to maintain.
3. Why is proficiency in C# crucial for .NET developers?
C# is the primary language used for .NET development. A developer needs to have a good understanding of C# syntax, type system, control structures, and error handling mechanisms.
For instance:
try
{
// Code that could throw an exception
}
catch (Exception ex)
{
// Handle the exception
}
finally
{
// Code to be executed regardless of an exception
}
This C# code snippet shows a basic try-catch-finally block used for error handling, a crucial feature of robust applications.
Pro tip: Keep exploring the latest features introduced in C#. Regularly updating your knowledge will help you write more efficient and cleaner code.
4. How important is the understanding of client-side technologies?
Client-side technologies like HTML, CSS, JavaScript, jQuery, and Bootstrap aid in creating rich, responsive, and user-friendly interfaces.
For example, using Bootstrap with Razor view engine in ASP.NET MVC:
@{
ViewBag.Title = "Home Page";
}
<div class="jumbotron">
<h1>ASP.NET</h1>
<p class="lead">Welcome to your new app.</p>
</div>
In this Razor view, we use Bootstrap classes to style our elements, demonstrating the importance of understanding these technologies.
Pro tip: Regularly practice creating dynamic and interactive web pages. It enhances your understanding of how client-side technologies can complement .NET MVC development.
5. Why is database application knowledge essential in .NET development?
Most applications involve data storage and retrieval, and .NET MVC developers often interface with databases like SQL Server. They need to understand SQL queries, relational database concepts, and Entity Framework—an ORM for .NET.
For instance:
using (var context = new MyContext())
{
var blogs = context.Blogs
.Where(b => b.Rating > 3)
.OrderBy(b => b.Url)
.ToList();
}
This code snippet uses Entity Framework to retrieve data from a database, showcasing the power and convenience of understanding database operations.
Pro tip: Be sure to understand concepts like database normalization and transactions. They are crucial when working with relational databases.
Delving into Web Development – What Skills to Look for in a .NET MVC Developer
It’s also crucial for the developer to have a comprehensive understanding of client-side technologies. Familiarity with HTML, CSS, JavaScript, jQuery, and Bootstrap contributes to building visually appealing and user-friendly web applications.
Database application knowledge is another vital skill. As .NET often interfaces with databases, a developer must be adept at performing database operations.
A .NET MVC developer should also be capable of code refactoring. This practice refines and optimizes the structure of the software, without altering its functionality.
First, let’s clarify some key terms:
- SQL: Stands for Structured Query Language. It is a standard language for storing, manipulating, and retrieving data in databases.
- Database Design: The process of producing a detailed data model of a database. It includes defining the tables, fields, relationships, views, indexes, and other elements.
- Entity Framework: An open-source object-relational mapping (ORM) framework for ADO.NET, provided by Microsoft. It enables .NET developers to work with relational data using domain-specific objects.
- LINQ: Stands for Language Integrated Query. It’s a Microsoft programming model that adds query capabilities to .NET languages.
- ASP.NET Core: The redesign of ASP.NET, a cross-platform, high-performance, open-source framework for building modern, cloud-based, internet-connected applications.
- Razor Pages: A page-based programming model that makes building web UI easier and more productive. It’s a feature of ASP.NET Core.
- MVC Architecture: Stands for Model-View-Controller. It’s a design pattern that separates an application into three main aspects: Model, View, and Controller.
1. Why is knowledge of client-side technologies significant for a .NET developer?
Client-side technologies like HTML, CSS, JavaScript, jQuery, and Bootstrap are vital for creating user-friendly, interactive, and visually appealing web applications.
For instance:
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
</head>
<body>
<div class="container">
<button class="btn btn-primary" id="myButton">Click me!</button>
</div>
<script src="https://code.jquery.com/jquery-3.3.1.slim.min.js"></script>
<script>
$('#myButton').click(function() {
alert('Button clicked!');
});
</script>
</body>
</html>
In this HTML document, we use Bootstrap for styling, and jQuery for handling a button click event.
Pro tip: Mastering modern JavaScript frameworks like Angular, React, or Vue.js can further enhance your skills and make your applications more robust and maintainable.
2. What makes database application knowledge crucial for .NET developers?
Effective management of data is at the heart of most applications. .NET MVC developers often work with databases and need to understand SQL, Entity Framework, and LINQ (Language Integrated Query).
Here’s a LINQ query example:
var highRatedBlogs = from b in context.Blogs
where b.Rating > 3
orderby b.Url
select b;
This code selects blogs with a rating greater than 3 from a database, showing how LINQ integrates querying capabilities directly into C#.
Pro tip: Understanding how to design and normalize databases can significantly improve your application’s efficiency and scalability.
3. How does code refactoring benefit .NET MVC development?
Code refactoring is a process of improving the design, structure, and implementation of the software while preserving its functionality. This aids in maintaining the code in the long run.
Consider the following example:
// Before refactoring
public double CalculateTotal(double price, int quantity, double tax)
{
return price * quantity + price * quantity * tax;
}
// After refactoring
public double CalculateTotal(double price, int quantity, double tax)
{
double subtotal = price * quantity;
return subtotal + subtotal * tax;
}
In the refactored version, the subtotal calculation is separated for clarity and easier maintenance.
Pro tip: Regular refactoring can help prevent your code from becoming overly complex or messy, making it easier to understand and maintain.
4. How does analytical thinking apply to .NET MVC development?
Analytical thinking allows developers to understand problems clearly, think logically, and develop effective solutions. For example, optimizing a slow running web page requires analytical skills to determine the root cause of the slowdown and devise the most efficient solution.
Pro tip: Regularly practice problem-solving and algorithm challenges on platforms like LeetCode or HackerRank to hone your analytical skills.
5. How does good communication aid in .NET MVC development?
Effective communication enables collaboration with team members, stakeholders, and clients. This ensures everyone is on the same page about project requirements and updates.
For instance, if you’re implementing a new feature, it’s crucial to discuss with your team to ensure the feature integrates seamlessly with the existing application.
Pro tip: Regularly practicing active listening and clear, concise speaking can significantly improve your communication skills, making you a more effective team member.
The Thinker and Communicator – What Skills to Look for in a .NET MVC Developer
On the soft skill side, analytical thinking is key. A developer must possess a problem-solving mindset, able to dissect requirements and devise strategic solutions.
Equally important are communication skills. Effective collaboration hinges on clear communication between the developer, the team, and other stakeholders.
First, let’s clarify some key terms:
Analytical Thinking: Analytical thinking is a critical component of visual thinking that gives one the ability to solve problems quickly and effectively.
It involves a methodical step-by-step approach to break down complex problems or processes into their constituent parts, identify their implications, and understand the relationships among these parts.
In the context of programming, analytical thinking allows a developer to decompose a problem into smaller, manageable parts, identify patterns, make logical connections, and make informed decisions or construct solutions for the problem.
It’s a crucial skill for troubleshooting and debugging, optimizing code, and implementing complex functionalities.
1. What is the significance of analytical thinking in .NET MVC development?
Analytical thinking enables a developer to break down complex problems into manageable parts, make logical connections, and devise effective solutions.
For instance, when debugging a performance issue, an analytical approach would involve identifying potential bottlenecks, investigating each area systematically, and making data-driven decisions to optimize the application.
public void OptimizeDataRetrieval()
{
// Using eager loading to prevent multiple round trips to the database
var blogs = context.Blogs
.Include(blog => blog.Posts)
.ToList();
}
Pro tip: Cultivate your analytical thinking by tackling complex coding problems, studying system design, and learning about algorithms and data structures.
2. Who benefits from effective communication in a development team?
Effective communication benefits everyone on the team. It enables the developer to clarify requirements, discuss strategies, share updates, and ask for help when needed. Additionally, it helps the team stay in sync and avoid misunderstandings that can lead to project delays or failure.
Example: A developer communicates with the product owner to understand the user requirements clearly, with fellow developers to ensure seamless integration of their components, and with testers to understand and resolve reported bugs.
Pro tip: Practice empathy and active listening to become a more effective communicator. Clear, concise, and respectful communication often yields better collaboration.
3. Where does communication come into play in .NET MVC development?
Communication plays a role in every stage of development. From initial requirement gathering and planning stages, through coding and testing phases, and onto deployment and maintenance.
Whether it’s discussing design patterns, clarifying business rules, reporting progress, or asking for help in troubleshooting a bug, communication is key.
Example: During a code review, the developer communicates their coding decisions, explaining why they chose a particular approach over another.
Pro tip: Effective written communication is equally important. Document your code and decisions well, and be clear and concise in your email and chat communications.
4. When is analytical thinking employed in .NET MVC development?
Analytical thinking is employed throughout the development process. It’s used when designing the software architecture, writing and optimizing code, debugging issues, and even when deciding which features would add the most value to the application.
Example: While designing a system, a developer uses analytical thinking to decide whether a microservices architecture would benefit the application more than a monolithic architecture.
Pro tip: Enhance your analytical thinking by exploring different areas of software development, like system design, project management, and user experience design.
5. Why is being both a thinker and communicator crucial in .NET MVC development?
Being both a thinker and communicator is essential because it ensures not only that you can solve complex problems and write efficient code, but also that you can effectively share your ideas, collaborate with your team, and understand the needs of your stakeholders.
It helps keep everyone on the same page, which is key to successful project completion.
Example: When a developer implements a feature, they use analytical thinking to devise the best implementation. They then communicate their approach to their team to ensure it aligns with the overall project.
Pro tip: Keep refining both your analytical thinking and communication skills—they are as crucial to your career as your technical skills.
Ensuring Quality – What Skills to Look for in a .NET MVC Developer
Quality is a cornerstone of good software development. Let’s delve into its importance for a .NET MVC developer through the lens of the what, who, where, when, why, and how structure.
1. What is the role of unit testing in ensuring quality?
Unit testing plays a vital role in ensuring code quality. It verifies that individual components of the application work as intended and helps catch bugs early in the development cycle.
Here’s a basic example of a unit test using the NUnit framework:
[Test]
public void TestAddition()
{
Calculator calculator = new Calculator();
int result = calculator.Add(2, 3);
Assert.AreEqual(5, result);
}
In this test, we create a new instance of the Calculator
class and test its Add
method by asserting that the result of adding 2 and 3 should equal 5.
Pro tip: Writing tests before coding (Test-Driven Development) can help improve code quality and design by forcing you to think through the functionality before implementation.
2. Who is responsible for maintaining code quality?
While the entire team is collectively responsible for maintaining code quality, a .NET MVC developer plays a key role by following best practices like writing clean, understandable code, performing thorough testing, and participating in code reviews.
Example: A developer runs unit tests before pushing their code to ensure they didn’t break any existing functionality.
Pro tip: Incorporate static code analysis tools like StyleCop or SonarQube in your development process to enforce coding standards and catch potential issues early.
3. Where do code refactoring and quality assurance intersect?
Code refactoring, the process of improving code without changing its functionality, intersects with quality assurance in maintaining the long-term health and readability of the codebase. It makes the code easier to understand, test, and maintain, which aids in assuring the overall quality of the software.
Example: Refactoring a long, complex method into several smaller, more manageable methods improves the maintainability and testability of the code.
// Before refactoring
public double CalculateTotal(double price, int quantity, double tax, double discount)
{
return (price * quantity + price * quantity * tax) * (1 - discount);
}
// After refactoring
public double CalculateSubtotal(double price, int quantity)
{
return price * quantity;
}
public double CalculateTax(double subtotal, double tax)
{
return subtotal * tax;
}
public double CalculateTotal(double subtotal, double tax, double discount)
{
double totalBeforeDiscount = subtotal + CalculateTax(subtotal, tax);
return totalBeforeDiscount * (1 - discount);
}
Pro tip: Consider using principles like SOLID and DRY to guide your refactoring and improve your code’s design.
4. When should a .NET MVC developer think about quality?
Quality should be a consideration throughout the entire development cycle. From the design phase, through coding and testing, and even during maintenance – every step of the way should involve checks and measures to ensure high quality.
Example: When adding a new feature, a developer not only thinks about meeting the requirements but also how to design and implement the solution in a way that maintains the code quality.
Pro tip: Adopting a ‘Quality First’ mindset can help prevent the accumulation of technical debt in your projects.
5. Why is ensuring quality so important in .NET MVC development?
Ensuring quality is crucial because it affects the maintainability, usability, and reliability of the software. High-quality code is easier to understand, less prone to bugs, and more easily extended or modified, all of which lead to a better end product.
Example: Through code reviews, developers not only catch bugs but also share knowledge, which results in better team alignment and higher quality code.
Pro tip: Regularly schedule time to address technical debt in your projects. This ongoing investment in code quality can pay off in increased productivity and reduced bug counts.
‘Nice-to-Haves’ – What Skills to Look for in a .NET MVC Developer
Having reviewed the essential skills, let’s discuss some ‘nice-to-haves.’ A look at a developer’s experience and portfolio can provide insight into their practical application of these skills.
The developer’s understanding of .NET Core is another bonus. As the future of .NET, this cross-platform version is a major asset for web services and console app development.
Moreover, an in-depth understanding of the MVC architecture — beyond just the ASP.NET MVC framework — is highly desirable.
Familiarity with DevOps practices is also advantageous. As more teams adopt DevOps, skills in continuous integration and delivery (CI/CD) can give a candidate an edge.
Security practices form another important consideration. Depending on the application being developed, an understanding of security best practices in the .NET environment can be crucial.
The Added Bonuses – What Skills to Look for in a .NET MVC Developer
In software development, there are skills and understanding that, while not strictly mandatory, add significant value to a developer’s toolkit. Let’s take a closer look at these “Added Bonuses” in the context of a .NET MVC developer
Before we dive in, let’s clarify some key terms:
- .NET Core: The cross-platform, high-performance, open-source framework for building modern, cloud-based, Internet-connected applications.
- DevOps: A set of practices that combines software development and IT operations to shorten the systems development life cycle and provide continuous delivery with high software quality.
- Security Best Practices: The recommended actions and operational strategies that focus on maintaining the integrity, confidentiality, and availability of IT systems and data.
- Trends and Best Practices: These are shifts in technology and recommended ways of doing things. They often reflect industry-accepted standards.
- Soft Skills: These are non-technical skills that relate to how you work and interact with others.
1. What additional advantage does understanding .NET Core provide?
Understanding .NET Core provides the advantage of being able to develop cross-platform applications. It is leaner and more modular than the standard .NET framework, making it ideal for building high-performance, modern applications that can run on any platform (Windows, Linux, macOS).
public class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Hello World! This is a .NET Core Application.");
}
}
This simple .NET Core application can run on any platform and prints “Hello World! This is a .NET Core Application.” to the console.
Pro tip: Microsoft’s focus is on .NET Core for future development, so investing time in learning it now can pay off in the long run.
2. Who benefits from a .NET MVC developer’s familiarity with DevOps practices?
Everyone involved in the development and deployment cycle benefits from a developer’s familiarity with DevOps practices.
It helps in automating processes, reducing the development cycle, improving collaboration, and speeding up the process of pushing changes to production, all while maintaining high-quality standards.
Example: Using CI/CD pipelines for automating the build, test, and deploy processes can significantly improve efficiency and reduce errors caused by manual steps.
Pro tip: Familiarize yourself with tools like Jenkins, Azure DevOps, or GitHub Actions to implement DevOps practices in your projects.
3. Where do security best practices come into play?
Security best practices come into play across the entire development process, from ensuring secure coding practices, handling data securely, to setting up secure deployment practices.
Example: Implementing proper user authentication and authorization using tools like ASP.NET Core Identity is one of the ways to follow security best practices.
Pro tip: Keep yourself updated with OWASP (Open Web Application Security Project) Top 10 vulnerabilities and the corresponding mitigation techniques.
4. When should a .NET MVC developer focus on trends and best practices?
A developer should always be aware of the latest trends and best practices. It’s part of their job to stay current, as technology is always evolving, and newer, more efficient ways of solving problems are continually being discovered.
Example: One of the current trends is containerization. Learning how to use Docker with .NET Core can enhance the process of developing, deploying, and scaling applications.
Pro tip: Regularly follow tech blogs, participate in developer forums, and attend webinars or conferences to stay up-to-date with the latest trends and best practices.
5. Why are soft skills important for a .NET MVC developer?
Soft skills are crucial for collaboration and effective communication within a team. They aid in understanding requirements, solving problems, and contributing positively to the team dynamic.
Example: Demonstrating good time management skills can show your ability to efficiently handle tasks and meet deadlines, which is vital for any project’s success.
Pro tip: While technical skills can get your foot in the door, your soft skills can open a lot of doors in your career. Keep improving them alongside your technical skills.
The Modern Developer – What Skills to Look for in a .NET MVC Developer
Today’s developers need a range of skills to stay relevant and competitive. Let’s explore what makes a “Modern Developer” in the context of a .NET MVC developer.
First, let’s clarify some key terms:
- Continuous Learning: This is the process of consistently upgrading one’s skills and knowledge throughout their career.
- Adaptability: The ability to change or be changed to fit altered circumstances. In software development, this refers to quickly learning new technologies and adapting to changes in the project.
- Collaboration Tools: These are tools that facilitate teamwork and communication among team members. They include version control systems, project management tools, and communication platforms.
- Cloud Technologies: Technologies that use the internet to deliver scalable and on-demand computing services. These include data storage, servers, databases, networking, software, analytics, and more.
- Remote Work: Working outside the traditional office environment, relying on technology to perform tasks and communicate with the team.
1. What does continuous learning mean for a .NET MVC developer?
Continuous learning for a .NET MVC developer means staying abreast of the latest technologies, methodologies, and best practices in their field. This could involve learning new programming languages, frameworks, or tools that can improve their effectiveness and efficiency.
Console.WriteLine("Always keep learning!");
This code simply prints “Always keep learning!” to the console, symbolizing a developer’s need for continual learning and growth.
Pro tip: Dedicate a certain amount of time each week for learning. It could involve reading blogs, taking online courses, or coding in a new language.
2. Who uses collaboration tools in a software development team?
Everyone in a software development team uses collaboration tools. These tools help in tracking tasks (like Jira), managing code (like Git), and facilitating communication (like Slack). A modern developer should be proficient in using these tools as they are integral to the software development process.
Example: Using Git, developers can work on different features concurrently, manage versions of code, and collaborate effectively without overwriting each other’s changes.
Pro tip: Learning to use collaboration tools effectively can boost your productivity and make collaboration smoother in a team environment.
3. Where do cloud technologies fit into the .NET MVC developer’s toolkit?
Cloud technologies fit into various aspects of a .NET MVC developer’s toolkit, from development and testing environments to deployment and scaling of applications. With services like Microsoft Azure or AWS, developers can create scalable, high-performing applications and services.
Example: Using Azure, a developer can deploy their .NET MVC application to a scalable and reliable cloud infrastructure, set up continuous integration/continuous deployment, and even leverage services for AI, Machine Learning, or IoT.
Pro tip: Gain hands-on experience with cloud platforms like Azure or AWS. Even a basic understanding of cloud concepts can be a valuable addition to your skills.
4. When does adaptability become crucial for a modern .NET MVC developer?
Adaptability becomes crucial when there are changes in the project requirements, technology stack, team structure, or the software development practices being followed. In the fast-evolving tech industry, being able to adapt quickly to new tools, languages, or methodologies is a valuable skill.
Example: When a project transitions from .NET Framework to .NET Core, a developer needs to adapt to the changes and nuances of .NET Core.
Pro tip: Embrace changes and see them as opportunities for learning and growth, rather than obstacles.
5. Why is understanding remote work important for a modern developer?
Understanding remote work is important as more companies are embracing remote or hybrid work models. It involves being comfortable with communication tools, managing time effectively, being proactive, and being able to work independently.
Example: Using tools like Zoom for meetings, Slack for communication, and Trello for managing tasks are part of effectively working remotely.
Pro tip: Good communication is even more crucial when working remotely. Be clear and concise in your communications, and don’t hesitate to ask for clarification when needed.
By focusing on these aspects, you’ll be well-equipped to identify the right .NET MVC developer for your team, ensuring successful, high-quality software development.
The 3 common problems CTOs have when asking: What Skills to Look for in a .NET MVC Developer
Problem 1: Finding Developers with a Balance of Technical Skills and Soft Skills
CTOs often struggle to find .NET MVC developers who not only have the necessary technical skills but also possess essential soft skills such as communication, problem-solving, and adaptability.
The consequence of this issue can lead to inefficient teamwork, miscommunication, and lower overall productivity in the team.
Solution: During the hiring process, besides technical interviews and coding tests, include behavioral interviews and problem-solving scenarios.
For example, you could use pair programming exercises where candidates need to work together with existing team members to solve a problem, allowing you to assess their communication and collaboration skills.
Problem 2: Keeping Up with the Pace of Technological Change
.NET is a rapidly evolving framework with new versions and tools being introduced regularly. CTOs can find it challenging to hire developers who are up-to-date with these changes.
If developers lack current knowledge, they may employ outdated practices, leading to inefficient code and systems that are not as robust or secure as they should be.
Solution: Look for developers who demonstrate a commitment to continuous learning. This can be indicated by their involvement in professional communities, contributions to open-source projects, or taking relevant courses.
Additionally, providing regular training and learning opportunities within the organization can also help in keeping the team up-to-date.
Problem 3: Assessing Practical Knowledge and Experience
CTOs often find it hard to assess the practical experience of a candidate purely based on their resume or an interview. A candidate may theoretically know about .NET MVC development, but lack the hands-on experience to apply this knowledge effectively in real-world projects.
This can lead to slower project completion times, buggy software, and ultimately dissatisfied customers.
Solution: Incorporate practical assessments in your hiring process. This could involve coding tests, review of portfolio projects, or asking the candidate to contribute to a small part of your actual codebase.
For example, you can provide a sample project specification and ask the candidate to submit a small piece of pseudocode or a code sample demonstrating how they would approach the problem.
//Sample: Implementing a simple Controller in ASP.NET MVC
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
}
In this simple example, the candidate is asked to implement a home controller for an ASP.NET MVC application. This not only tests their technical knowledge but also their understanding of the MVC architecture.
Pro tip: Look beyond the specific languages and tools a developer has used. A great developer can pick up new languages and tools, so it’s more important to hire someone who can think logically, solve problems, and has a good understanding of software development principles.
Wrapping about What Skills to Look for in a .NET MVC Developer
Hiring the right talent is critical, but identifying them need not be a struggle. Remember, it’s all about finding the right blend of hard skills, soft skills, and cultural fit.
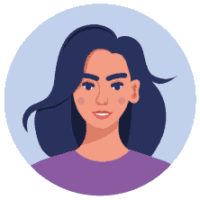
Jessica is a highly accomplished technical author specializing in scientific computer science. With an illustrious career as a developer and program manager at Accenture and Boston Consulting Group, she has made significant contributions to the successful execution of complex projects.
Jessica’s academic journey led her to CalTech University, where she pursued a degree in Computer Science. At CalTech, she acquired a solid foundation in computer systems, algorithms, and software engineering principles. This rigorous education has equipped her with the analytical prowess to tackle complex challenges and the creative mindset to drive innovation.
As a technical author, Jessica remains committed to staying at the forefront of technological advancements and contributing to the scientific computer science community. Her expertise in .NET C# development, coupled with her experience as a developer and program manager, positions her as a trusted resource for those seeking guidance and best practices. With each publication, Jessica strives to empower readers, spark innovation, and drive the progress of scientific computer science.