Choosing the right programming language can be a daunting task for developers, especially when they need to weigh the pros and cons of multiple options. In this article, we will compare the C# and Dart programming languages, evaluating their language features, syntax, and use cases to provide insights for developers considering C# or Dart.
Overview of C#
C# (pronounced “C sharp”) is a modern, object-oriented programming language developed by Microsoft as part of the .NET framework. It was first introduced in 2000 and has since become one of the most popular programming languages in the world. C# is designed to be simple, efficient, and easy to learn, making it a great choice for both beginners and experienced developers alike.
One of the key features of C# is its strong type system, which helps prevent common programming errors such as null reference exceptions. Additionally, C# provides support for a wide range of programming paradigms, including functional programming, imperative programming, and object-oriented programming.
C# is commonly used in a variety of industries, including finance, healthcare, and gaming. It is particularly popular for building desktop and web applications, as well as mobile apps using Xamarin. C# also has a large and active developer community, with many resources available for learning and support.
Overview of Dart
Dart is a client-optimized programming language developed by Google in 2011. It was designed as a general-purpose language that compiles to JavaScript and provides a more modern and efficient alternative to JavaScript.
Dart’s syntax is similar to that of Java and C++, making it easy for developers to transition to Dart. Dart’s main features include optional typing, garbage collection, and a just-in-time (JIT) compiler.
Dart has gained popularity in recent years and is commonly used for web and mobile app development, as well as for server-side programming. It is particularly popular for building web applications with Flutter, a UI toolkit that allows developers to build natively compiled applications for mobile, web, and desktop from a single codebase.
Syntax Comparison
When comparing C# and Dart programming languages, evaluating language features and syntax is crucial for developers. Both languages have their own unique syntax, but they also share some similarities. Let’s take a closer look.
Syntax Examples
Here is an example of creating a simple variable in C#:
// Creating a variable in C#
string name = “John”;
And here is the same example in Dart:
// Creating a variable in Dart
String name = “John”;
As you can see, the syntax for creating a variable in both C# and Dart is very similar. The main difference is the use of capitalization for the variable type in Dart.
Conditional Statements
The syntax for conditional statements is also similar in both languages. Here is an example of an if statement in C#:
// If statement in C#
if (age >= 18)
{
Console.WriteLine(“You are an adult.”);
}
And here is the same example in Dart:
// If statement in Dart
if (age >= 18)
{
print(“You are an adult.”);
}
Again, the syntax for the if statement is very similar in both languages. The only difference is the use of “Console.WriteLine” in C# versus “print” in Dart.
Conclusion
Overall, while there are some syntax differences between C# and Dart, the languages share many similarities. As a developer, it’s important to understand the syntax of both languages in order to choose the best one for the task at hand.
Frameworks and Libraries
One of the key factors in choosing a programming language is the availability and popularity of frameworks and libraries. Both C# and Dart have a robust set of tools to help with development.
Frameworks in C#
C# has a wide range of popular frameworks, each suited for different purposes. Some of the most commonly used include:
Framework Name | Description |
---|---|
.NET Framework | A widely used framework for building Windows applications and web applications with ASP.NET. |
Xamarin | A mobile development framework that allows for building cross-platform apps for iOS, Android, and Windows Phone. |
Entity Framework | An ORM that enables developers to work with databases using C# objects. |
Let’s take a look at an example of using Entity Framework in C#:
// Define a class that will be a table in the database
public class Product {
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}// Use Entity Framework to query the database
using (var context = new ProductContext()) {
var products = context.Products
.Where(p => p.Price > 10.00)
.OrderBy(p => p.Name)
.ToList();
}
Frameworks in Dart
Dart also has a variety of frameworks and libraries available to developers. Some of the most popular include:
Framework Name | Description |
---|---|
Flutter | A mobile development framework that allows for building high-performance, cross-platform apps for iOS and Android. |
AngularDart | A web development framework that is a port of the popular Angular framework to Dart. |
Aqueduct | A server-side web development framework that enables building REST APIs and web applications. |
Here’s an example of using Flutter in Dart to create a simple app:
// Define a stateful widget for the app
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}// Define the state of the widget
class _MyAppState extends State {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: ‘My App’,
home: Scaffold(
appBar: AppBar(
title: Text(‘My App’),
),
body: Center(
child: Text(‘Hello, world!’),
),
),
);
}
}// Run the app
void main() {
runApp(MyApp());
}
Overall, both C# and Dart have a strong set of frameworks to choose from, allowing developers to build a variety of applications with ease.
Performance and Efficiency
When it comes to evaluating programming languages, performance is often a critical factor. Developers need to ensure their code is efficient and can handle large or complex tasks without slowdowns or crashes. In this section, we will compare the performance and efficiency of C# and Dart, considering key factors such as execution speed, memory usage, and overall resource utilization.
According to recent benchmarks, C# performs well in terms of speed and memory usage, making it an excellent choice for complex enterprise applications or programs that operate on large data sets. Additionally, the language’s ability to interact with hardware and other low-level components makes it useful for building system-level applications.
On the other hand, Dart is designed with performance in mind, and its Ahead-of-Time (AOT) compilation process helps to optimize code for faster execution. This optimization makes Dart a strong choice for mobile development, as it can handle multiple concurrent operations while using minimal memory and processing power.
It is worth noting that while C# has been around for longer and has had time to develop robust performance optimizations, Dart is a younger language that is still evolving. As such, it is possible that Dart’s performance will continue to improve as it gains wider adoption and more research is conducted into its potential use cases.
Overall, the performance and efficiency of C# and Dart will depend on the specific use case and project requirements. Developers should consider the size and complexity of their application, as well as the available development resources and potential hardware limitations. By carefully evaluating the language features and performance benchmarks, developers can choose the programming language that best meets their needs and delivers optimal performance.
Use Cases and Industry Trends
Both C# and Dart have a wide range of potential use cases, with each language being better suited to certain industries or project types. Understanding these use cases can help developers make an informed decision when choosing between the two languages.
One of the primary industries where C# is commonly used is finance. The language’s performance, type safety, and extensive libraries make it an excellent choice for developing complex financial applications. C# is also popular in the gaming industry, with many game engines and tools being built using the language. Additionally, C# is used heavily in enterprise development, particularly for building Windows desktop and server applications.
Dart, on the other hand, is often used in web development. Its client-side and server-side capabilities make it a versatile choice for building web applications. Flutter, Google’s UI toolkit for building natively compiled applications for mobile, web, and desktop, is built using Dart. This makes Dart a popular choice for cross-platform mobile development as well.
In terms of industry trends, C# has seen consistent growth over the past few years, with Microsoft investing heavily in the language and its associated tools and frameworks. The rise of .NET Core, the open-source, cross-platform successor to the .NET Framework, has also increased the popularity of C# among developers. Meanwhile, Dart is seeing increased adoption in the web development world, particularly for building web applications using Google’s Flutter framework.
Overall, both C# and Dart have their strengths and weaknesses when it comes to use cases and industry trends. Developers should consider their project requirements and the industries they are working in when deciding between the two languages.
Learning Curve and Community Support
When deciding between C# and Dart, evaluating the learning curve and community support is essential. C# is known for its easy-to-learn syntax, making it an attractive option for beginners. Microsoft, the developer of C#, offers extensive documentation and resources, including a dedicated website and a large community of developers. Additionally, the .Net framework offers a wide range of resources, including libraries and tools, to facilitate development.
Dart, on the other hand, may have a steeper learning curve for some developers due to its unique syntax and structure. However, it offers a rich set of features that makes it attractive for building complex applications. The documentation provided by Google, the developer of Dart, is comprehensive and frequently updated, and the community support has grown over the years. The Flutter framework, built on top of Dart, has gained popularity in recent years, and offers a wide variety of resources and documentation to aid in development.
Ultimately, your decision will depend on your specific needs and experience level. If you are new to programming or looking for a language with an easy-to-learn syntax, C# may be the better choice. However, if you have experience with programming and need a language with advanced features for building complex applications, you may find Dart to be a better fit. Regardless of your choice, both languages offer ample support and resources to aid in your development journey.
Future Outlook and Adoption
Looking ahead, both C# and Dart have promising futures in the software development industry. C# has been around for over two decades and has continued to evolve with the times, making it a reliable choice for many developers. Microsoft has also shown a commitment to the language, with regular updates and improvements to the .NET framework.
Dart, on the other hand, is still relatively young but has gained traction in recent years, thanks in part to its use in developing Flutter applications. The language has a growing community and is backed by Google, which makes it a language worth watching in the future.
When it comes to adoption, C# has a well-established presence in industries such as finance, healthcare, and gaming. Dart, on the other hand, is commonly used in mobile and web development, particularly for developing native apps with Flutter.
Overall, both languages have a bright future, but their adoption rates may vary depending on the industry and specific use case. Developers considering either language should evaluate their requirements and consider the strengths and weaknesses of each language before making a decision on which to use.
Conclusion: Which Language to Choose?
After comparing and evaluating the features, syntax, frameworks, performance, use cases, learning curve, community support, future outlook, and adoption rates of C# and Dart, it is clear that both have their unique strengths and weaknesses. Ultimately, the decision of which language to choose will depend on your specific needs and requirements.
If you are already familiar with .NET and Microsoft technologies, then C# may be the best choice for you. It has a large and active developer community, excellent support and documentation, and is commonly used in industries such as finance, healthcare, and gaming.
However, if you are looking for a more modern and versatile language that can be used for both frontend and backend development, then Dart may be the way to go. It has a simpler and more readable syntax, excellent performance, and is commonly used in industries such as web development, mobile app development, and Internet of Things (IoT).
Considerations for Choosing Between C# and Dart
Here are some additional factors to consider when choosing between C# and Dart:
- Project Type: Consider the type of project you will be working on and which language will be the best fit. For example, if you are building a desktop application, C# may be the better choice. If you are building a web application, Dart may be the better choice.
- Team Skills and Experience: If you already have a team of developers who are experienced in C#, it may be more efficient to continue using C#. However, if your team is open to learning a new language, Dart may be a good choice to explore.
- Availability of Frameworks and Libraries: Consider the availability and popularity of frameworks and libraries for both languages. If there is a framework or library that you absolutely need for your project, make sure it is available in the language you choose.
- Future Outlook: Consider the ongoing development and support for both languages. While both languages have promising futures, it may be worth considering which language is seeing more growth and innovation in the software development industry.
Ultimately, the decision of which language to choose should be based on a variety of factors. We hope this comparison has provided insights and guidance as you consider C# and Dart for your next project.
FAQ
Q: What is the difference between C# and Dart?
A: C# and Dart are two different programming languages. C# is primarily used for developing Windows applications and is supported by Microsoft, while Dart is a language developed by Google and is often used for web and mobile app development.
Q: Which language is easier to learn, C# or Dart?
A: The ease of learning C# or Dart depends on your existing programming knowledge and experience. Both languages have comprehensive documentation and active developer communities that can provide support and resources for learning.
Q: Which industries use C# and Dart?
A: C# is commonly used in industries such as finance, gaming, and enterprise software development. Dart is often used in web and mobile app development, and it has gained popularity in industries such as fintech and education.
Q: Are there frameworks and libraries available for C# and Dart?
A: Yes, both C# and Dart have a wide range of frameworks and libraries available. C# has frameworks like ASP.NET and Xamarin, while Dart has frameworks like Flutter. These frameworks provide developers with tools and resources to build applications more efficiently.
Q: Which language has better performance, C# or Dart?
A: The performance of C# and Dart can vary depending on the specific use case and implementation. Both languages are optimized for performance, but factors such as execution speed and memory usage can differ. It’s recommended to benchmark and profile your code to determine which language performs better for your specific requirements.
Q: What are the use cases for C# and Dart?
A: C# is commonly used for developing Windows desktop applications, web applications, and games. Dart is often used for web and mobile app development, specifically with the Flutter framework. It can be used to create cross-platform apps for iOS, Android, and the web.
Q: How is the learning curve for C# and Dart?
A: The learning curve for C# and Dart can vary depending on your prior programming experience. If you are already familiar with object-oriented programming concepts, both languages should be relatively easy to pick up. There are also plenty of learning resources available, including tutorials, documentation, and online communities, to help you learn either language.
Q: What is the future outlook for C# and Dart?
A: Both C# and Dart have strong backing from their respective organizations and continue to receive updates and improvements. C# has a long-standing history and is widely adopted, while Dart is gaining traction in web and mobile app development. The future growth and adoption of these languages will likely be influenced by industry trends and evolving technology needs.
Q: Which language should I choose, C# or Dart?
A: The choice between C# and Dart depends on your specific needs and requirements. If you are developing Windows applications or have experience with the Microsoft ecosystem, C# may be a better fit. If you are focusing on web and mobile app development, Dart with the Flutter framework can be a powerful combination. Consider factors such as industry trends, available resources, and your existing skillset when making your decision.
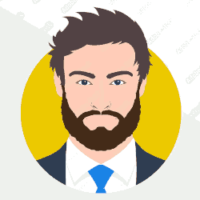
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.