
.NET Core and CMS Integration
For developers looking to build websites or applications with .NET Core, selecting the right content management system (CMS) is crucial. In order to make the most of the benefits offered by .NET Core, choosing a CMS that is compatible with this framework can greatly enhance the efficiency and productivity of your web development project.
So what is .NET Core? It is a free, open-source, cross-platform framework developed by Microsoft and used to build modern, cloud-based, internet-connected applications. .NET Core is an evolution of the .NET Framework that is focused on reducing the complexity of web development.
When it comes to CMS integration, .NET Core offers a number of advantages. First and foremost, its compatibility with a range of CMS solutions allows developers to choose the best fit for their specific requirements. For example, Umbraco, a popular open-source CMS, has been updated to work with .NET Core. The integration of .NET Core and Umbraco has resulted in a more scalable, performant CMS that can efficiently manage content across different devices and platforms.
Another key benefit of .NET Core is its flexibility and versatility. It can integrate with various CMS frameworks, including Sitecore, Kentico, Orchard Core, and more. This provides developers with a wide range of options to choose from, depending on the specific features and functionalities required by their web development project.
When it comes to integrating CMS platforms with .NET Core, there are various approaches that can be taken. For example, developers can use webhooks or APIs to connect the CMS and the .NET Core application. Alternatively, if the CMS has a plugin or extension available, it can be installed and customized to meet the specific requirements of the project.
Ultimately, the integration of CMS solutions with .NET Core offers developers a powerful toolset to build high-performing, user-friendly web applications. By understanding the capabilities and benefits of this framework, developers can make informed decisions about which CMS solutions to choose for their specific project needs.
Key Factors to Consider for CMS Selection in .NET Core Projects
Choosing the right CMS for .NET Core projects requires careful consideration of several key factors. Here are some of the most important aspects to keep in mind when selecting a CMS compatible with .NET Core:
- Scalability: Consider the CMS’s ability to handle large-scale projects and accommodate growth in the future. Look for a CMS that can scale easily and handle heavy traffic without compromising performance.
- Security: Security is a critical aspect of any CMS, especially for websites that handle sensitive user information. Look for CMS solutions that offer robust security features and follow industry best practices.
- Ease of Use: An intuitive and user-friendly CMS interface can save time and increase productivity. Consider how easy it is to navigate, author content, and manage the website.
- Customization Options: Choose a CMS that offers enough customization options to meet your specific needs and requirements. Look for a CMS that allows for seamless integration with other technologies and offers flexibility in design and functionality.
- Community Support: Consider the size and activity level of the CMS’s community. A large community can provide valuable resources, including documentation, tutorials, and support.
By carefully evaluating these factors, developers can select the best CMS solution for their .NET Core projects. Keep in mind that the optimal CMS choice may vary depending on the specific project requirements and the available CMS options.
Best CMS Solutions for .NET Core
When it comes to selecting the best CMS solution for .NET Core, there are a variety of options available, each with its own unique features and benefits. To help you select the optimal CMS for your web application needs, we have identified the top CMS solutions for .NET Core based on their performance, functionality, and community support.
1. Umbraco
Umbraco is a free, open-source CMS platform that offers a flexible and extensible content management system. It is built on the .NET framework and offers seamless integration with .NET Core. With Umbraco, developers have the ability to customize the platform to their specific requirements and create dynamic and interactive websites.
Pros | Cons |
---|---|
Flexible and customizable. | Steep learning curve for beginners. |
Scalable and secure. | Limited themes and templates available. |
Active community and good documentation. | Requires additional plugins for certain functionality. |
Example Code:
// Get content by document type
var service = scope.ServiceProvider.GetService(typeof(IPublishedContentQuery));
var contentItems = service.GetType().GetMethod("Content")
.Invoke(service, new object[] { "documentTypeName" })
as IEnumerable;<IPublishedContent>;
2. Orchard Core
Orchard Core is a modular and multi-tenant CMS platform that offers a range of features and extensibility options. It is built on the .NET Core framework and can be easily integrated with .NET Core applications. With Orchard Core, developers have the ability to create dynamic and scalable websites with ease.
Pros | Cons |
---|---|
Modular and extensible architecture. | Documentation can be limited. |
Integrates easily with .NET Core. | Requires some technical knowledge to fully utilize all features. |
Scalable and secure. | Some features may require additional configuration or customization. |
Example Code:
// Register services for a module
public void ConfigureServices(IServiceCollection services)
{
services.AddScoped<IProductService, ProductService>();
}
By selecting the optimal CMS solution for your .NET Core project, you can ensure that your website performs optimally and offers a superior user experience. With the right CMS in place, developers have the flexibility and versatility to create dynamic and interactive websites with ease.
Considerations for Seamless Integration and Migration
Integrating and migrating existing CMS solutions to .NET Core can be a challenging process. However, with proper planning and best practices, it can be achieved with minimal downtime and data loss. Below are some considerations for ensuring a smooth transition:
1. Identify Dependencies
Before migrating to .NET Core, it’s essential to identify all the dependencies associated with your existing CMS. These could include database connections, third-party libraries, and custom modules. Ensuring that all dependencies are compatible with .NET Core is crucial for a successful migration.
2. Plan for Downtime
Migrating a CMS to a new platform requires some downtime, which can impact user experience and SEO rankings. To minimize the impact, it’s important to plan for downtime in advance and to communicate it clearly to users so they can adjust their expectations accordingly.
3. Test and Validate
Testing and validating the migration process is crucial to ensure that everything works as expected. This includes testing the compatibility of all dependencies, as well as validating that all data and functionality have been successfully migrated. It’s also important to check that the new CMS is performing optimally and providing the expected user experience.
4. Use Best Practices
When migrating to .NET Core, it’s essential to follow best practices to minimize the risk of data loss and ensure the security of your CMS. This includes making regular backups of your data, using secure passwords for all user accounts, and following recommended security guidelines for .NET Core development.
By following these considerations and best practices, developers can ensure a seamless integration and migration of their existing CMS solutions to .NET Core, enabling them to take advantage of the benefits that .NET Core provides.
Leveraging CMS Features in .NET Core Projects
One of the major benefits of using a CMS solution for .NET Core websites is the ability to leverage its features and functionality within your applications. This section will provide examples of how developers can integrate CMS capabilities into .NET Core projects, highlighting the versatility and flexibility of these solutions.
Content Management
A CMS is designed to manage content, making it an invaluable tool for websites that require frequent updates. Developers can use CMS features to create, edit, and publish content without writing complicated code. For example, in the Umbraco CMS, developers can retrieve content using a simple query, as shown below:
// Retrieve content by Id
var content = Umbraco.Content(id);
This code snippet retrieves the content with the specified Id, allowing developers to easily manipulate and display the content within their applications.
User Authentication
Most CMS solutions come with built-in user authentication functionality, allowing developers to easily manage user accounts and permissions. This can be particularly useful for applications that require user registration and login. In the Kentico CMS, developers can use the built-in authentication API to manage user authentication, as shown below:
// Authenticate user
var user = Authentication.Authenticate(userName, password);
This code snippet authenticates the user with the specified username and password, allowing developers to control access to their applications.
E-commerce Capabilities
Many CMS solutions offer e-commerce capabilities, allowing developers to create online stores and manage product listings. This can be particularly useful for businesses that sell products or services online. In the Sitefinity CMS, developers can use the built-in e-commerce API to manage product listings, as shown below:
// Retrieve product by Id
var product = ecommerceManager.GetProduct(productId);
This code snippet retrieves the product with the specified Id, allowing developers to add, update, or remove products from their online store.
By leveraging the features and capabilities of CMS solutions, developers can save time and effort when building .NET Core websites. With a little bit of code, they can add powerful functionality to their applications, providing an exceptional user experience.
Tips for Optimizing Performance in CMS-Driven .NET Core Websites
Optimizing performance in CMS-driven .NET Core websites is critical to ensuring a seamless user experience. Here are some tips and techniques to help improve website performance:
1. Use Caching Strategically
Caching can significantly improve website performance by reducing the amount of time it takes to generate pages. Implementing caching at different levels in your application can help ensure that frequently accessed content is readily available, reducing the load on your application servers. In .NET Core, you can use the built-in Memory Cache or distributed caching solutions such as Redis or Memcached for maximum performance benefits.
2. Optimize Your Database
Database optimization is often overlooked but can have a significant impact on website performance. Ensure that your database schema is designed with performance in mind, normalize your tables, and create indexes on frequently queried columns. In addition, regularly clean up unnecessary data, monitor database performance metrics, and ensure that you are using the most appropriate and up-to-date database engine for your application needs.
3. Minimize HTTP Requests
The time it takes to download and render web pages is directly affected by the number of HTTP requests your application makes. To optimize performance, you should minimize the number of HTTP requests by:
- Combining multiple files, such as CSS and JavaScript, into a single file
- Using CSS sprites to reduce the number of image requests
- Implementing lazy loading for images and other content, to reduce the number of requests for content that is below the fold
4. Optimize Code
Code optimization can also have a significant impact on website performance. You can optimize your code by:
- Minimizing the number of method calls
- Using caching for frequently accessed data
- Minimizing database roundtrips
- Using efficient algorithms and data structures
5. Use Content Delivery Networks (CDNs)
CDNs can significantly boost website performance by reducing page load times and improving reliability. By caching your website’s content on servers distributed around the globe, CDNs can ensure that users can access your content quickly and reliably, regardless of their location. .NET Core makes it easy to integrate with popular CDNs such as Cloudflare, Akamai, and Amazon CloudFront.
By following these optimization tips, you can ensure that your CMS-driven .NET Core website performs optimally, providing an exceptional user experience.
Final Thoughts
Choosing the right CMS solution for .NET Core projects can have a significant impact on efficiency, productivity, and web application performance. By considering factors such as scalability, security, ease of use, customization options, and community support, developers can select an optimal CMS solution that caters to their specific requirements.
After comparing and contrasting popular CMS solutions, we have highlighted the best options for .NET Core-based websites, including Umbraco, Kentico Xperience, and Sitefinity. These CMS solutions offer unique features, functionalities, and community support, making them ideal for various web application development needs.
By integrating CMS functionality within .NET Core projects, developers can leverage the power of content management, user authentication, and e-commerce capabilities, among others. Additionally, by optimizing the performance of CMS-driven .NET Core websites using caching strategies, database optimizations, and code optimization techniques, developers can ensure an exceptional user experience and website performance.
As the CMS landscape continues to evolve, keeping up with industry trends and new developments can help developers stay ahead of the curve. We hope this article has provided valuable insights and guidance for selecting and leveraging CMS solutions compatible with .NET Core in web application development.
- Best CMS solutions for .NET Core: Umbraco, Kentico Xperience, Sitefinity
- Factors to consider: scalability, security, ease of use, customization options, community support
- Integrating CMS functionality within .NET Core projects
- Optimizing performance: caching strategies, database optimizations, code optimization techniques
External Resources
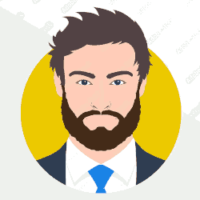
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.