The process of building Android apps using .NET technology can seem daunting. However, we will walk you through each step of the way, from setting up your development environment to deploying your app. In this guide, we will not only cover the basics but also dive into the details of app architecture, UI design, functionality implementation, testing, debugging, and cross-platform development.
If you are wondering why you should choose .NET technology for Android app development, the answer is simple. .NET is a reliable and robust framework that allows the development of high-quality and efficient Android apps. Additionally, .NET offers cross-platform capabilities, making it easier for developers to build apps that run on multiple platforms, including Android.
Throughout this guide, we will provide .NET code examples to illustrate programming concepts, compare and analyze different frameworks and languages, and reference industry examples and trends if relevant. So, let’s get started and discover the endless possibilities of building Android apps using .NET technology!
Introduction to Android App Development with .NET
Welcome to the world of Android app development with .NET! The .NET framework provides a powerful and flexible platform for building high-performance, robust, and feature-rich Android applications. With .NET, developers can leverage their existing skills and experience to create native Android apps quickly and easily.
One major advantage of using .NET for Android app development is its cross-platform capabilities. You can write code once and deploy it to multiple platforms, including Android, iOS, and Windows. This not only saves time and effort but also enables you to reach a broader audience.
In this guide, we will walk you through the process of creating an Android app using .NET. We’ll cover everything from setting up your development environment to deploying your app. We’ll also explore different frameworks, tools, and libraries available for Android app development with .NET. By the end of this guide, you will have a solid understanding of how to build Android apps using .NET and the tools and techniques needed to succeed in the Android app development market.
Setting Up the Development Environment
To start developing Android apps with .NET, you need to set up your development environment. The following steps will guide you through the installation and configuration process:
- Download and install Visual Studio, the Integrated Development Environment (IDE) for .NET development. Visual Studio provides a rich set of features for building Android apps with .NET.
- Install the Android SDK (Software Development Kit) and the Android NDK (Native Development Kit) from the Android Developer website.
- Install the Xamarin Android SDK. Xamarin is a cross-platform development toolset that enables you to develop Android apps using .NET.
- Create a new Android project in Visual Studio. Select the Android template, and choose the minimum required Android version for your app.
- Configure the project settings. Set the package name, target Android version, and other application-specific settings.
- Install the required NuGet packages for your project. NuGet is a package manager for .NET that enables you to easily install and manage external libraries and frameworks for your app.
Once you have completed these steps, you are ready to start building your Android app with .NET.
It is important to note that the installation process may vary depending on the version of Visual Studio and the Android SDK you are using. Always refer to the official documentation for up-to-date instructions.
By properly setting up your development environment, you can ensure that you have all the necessary tools and frameworks to develop high-quality Android apps with .NET. This will help you streamline your development process, making it more efficient and productive.
Programming tip: You can use the Android Emulator to test your app during development. The emulator enables you to simulate different Android devices and versions, allowing you to test your app in a variety of scenarios.
With the right tools and environment, you can start building powerful Android apps with .NET. In the next section, we will discuss how to choose the right framework for your Android app development needs.
Choosing the Right Framework for Android App Development
Choosing the right development framework plays a critical role in the success of any Android app development project. When it comes to using .NET for Android app development, there are multiple frameworks to choose from. In this section, we will compare and analyze the different frameworks, highlighting their features and differences.
Xamarin
Xamarin is a popular framework for cross-platform app development using .NET technologies. With Xamarin, you can develop Android apps using C# and .NET APIs. Xamarin.Forms is a cross-platform UI toolkit that allows developers to create user interfaces that can be shared across Android, iOS, and Windows platforms. With Xamarin, you can share code, business logic, and UI across multiple platforms, making it an efficient option for development teams.
Here is an example of Xamarin code:
// Create a new label
Label label = new Label();
// Set the text property
label.Text = “Hello, World!”;
// Add the label to the layout
layout.Children.Add(label);
Native Android Development
Native Android Development using Java and Kotlin is a widely used framework for Android app development. It offers an extensive library of APIs and tools to develop high-quality Android applications. Native development allows developers to leverage the latest features and updates from Google.
Here is an example of Java code for Android development:
// Create a new button
Button button = new Button(this);
// Set the text property
button.setText(“Click me”);
// Add the button to the layout
layout.addView(button);
Comparison of Xamarin and Native Android Development
When choosing between Xamarin and Native Android Development using Java or Kotlin, developers need to consider the requirements of their project, including performance, speed, and user experience. While Xamarin offers cross-platform capabilities, which can save time and resources, Native Android Development allows developers to leverage the latest features and updates from Google.
Framework | Pros | Cons |
---|---|---|
Xamarin | Cross-platform capabilities, shares code across multiple platforms, fewer lines of code required. | Slower app performance, large app package size, less access to native features and APIs. |
Native Android Development | Offers extensive library of APIs and tools, access to the latest features and updates from Google, fast performance, and smoother user experience. | More time-consuming development process, requires extensive knowledge of Java or Kotlin. |
It’s important to note that both frameworks have their strengths and weaknesses. Developers need to assess their project requirements and choose the framework that best suits their needs.
In conclusion, choosing the right development framework for Android app development is crucial for the success of any project. While Xamarin offers cross-platform capabilities, Native Android Development allows developers to leverage the latest features and updates from Google. We recommend that developers assess their project requirements and choose the framework that best suits their needs.
Understanding Android App Architecture with .NET
Developing Android apps with .NET requires a solid understanding of app architecture. The app architecture defines how the different components of your app interact and how they are organized. A well-designed app architecture can promote scalability, maintainability, and testability.
In Android app development, the Model-View-ViewModel (MVVM) pattern is a popular architectural pattern that has gained traction in recent years. It separates the UI logic from the business logic, making it easier to develop, test, and maintain the app. In the MVVM pattern, the Model represents the data and business logic, the View represents the UI and visual elements, and the ViewModel acts as the mediator between the View and the Model.
With .NET, you can easily implement the MVVM pattern in your Android app development. The .NET framework provides data binding, which allows you to bind the UI elements to the ViewModel properties and methods. This simplifies the process of updating the UI when the ViewModel data changes.
Benefits of the MVVM Pattern
The MVVM pattern offers several benefits for Android app development with .NET:
- Separation of concerns: The MVVM pattern separates the UI logic from the business logic, making it easier to develop and test the app.
- Testability: With the MVVM pattern, the ViewModel can be easily tested without requiring a UI.
- Flexibility: The MVVM pattern allows for easy integration of third-party libraries and frameworks.
Implementing the MVVM pattern with .NET
Here’s an example of how to implement the MVVM pattern in your Android app development with .NET:
ViewModel Class:
“`c#
public class MainViewModel : INotifyPropertyChanged
{
private string _name;public string Name
{
get { return _name; }
set
{
_name = value;
OnPropertyChanged(“Name”);
}
}public event PropertyChangedEventHandler PropertyChanged;
private void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
“`
The ViewModel class above defines the data and business logic for the app. It contains a Name property that can be bound to the UI. The OnPropertyChanged method is called whenever the Name property is updated, which then updates the UI.
View Class:
“`c#
public class MainActivity : AppCompatActivity
{
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);MainViewModel viewModel = new MainViewModel();
SetContentView(Resource.Layout.activity_main);
EditText nameEditText = FindViewById(Resource.Id.nameEditText);
TextView greetingTextView = FindViewById(Resource.Id.greetingTextView);nameEditText.TextChanged += (s, e) =>
{
viewModel.Name = nameEditText.Text;
};viewModel.PropertyChanged += (s, e) =>
{
if (e.PropertyName == “Name”)
{
greetingTextView.Text = $”Hello, {viewModel.Name}!”;
}
};
}
}
“`
The View class above represents the UI of the app. It contains an EditText element for the user to enter their name and a TextView element to display a greeting message. The ViewModel is instantiated and bound to the UI elements in the OnCreate method. Whenever the user enters their name, the Name property of the ViewModel is updated, which then updates the greeting message.
Overall, understanding the MVVM pattern and its implementation with .NET is essential for developing efficient and scalable Android apps. By leveraging the power of MVVM, you can easily separate your UI and business logic, making your app easy to test and maintain.
Designing the User Interface
Designing a user-friendly and visually appealing UI plays a crucial role in the success of any Android app. With .NET, you have access to a variety of UI frameworks and tools to help you create stunning interfaces. Let’s explore some of these options:
.NET UI Frameworks for Android
The following are some of the most popular UI frameworks for Android app development with .NET:
Framework | Description |
---|---|
Xamarin.Forms | A UI toolkit that allows you to create native user interfaces for Android using a single, shared codebase. It provides a wide range of controls and layout options, making it easy to create cross-platform apps that look and feel native on every platform. |
Xamarin.Android | A UI toolkit that offers a set of pre-built controls and layout templates for Android app development. It also provides access to the Android Designer tool, allowing you to drag-and-drop your UI elements into place. |
Uno Platform | A UI platform that allows you to create cross-platform apps with a single codebase. It provides a wide range of UI controls and layouts, as well as support for both XAML and C#. |
Android App UI Design
When designing your Android app UI, keep in mind the following best practices:
- Keep it simple and intuitive: Use clear and concise labeling for your UI elements, and make sure they are easy to understand and use.
- Consistency is key: Use consistent colors, fonts, and layouts throughout your app to create a cohesive and professional look.
- Make it responsive: Your UI should adapt to different screen sizes and resolutions, ensuring that your app looks great on any device.
Here’s an example of how to create a simple layout using Xamarin.Forms:
//Create a StackLayout
StackLayout layout = new StackLayout();
//Add a label
Label label = new Label();
label.Text = “Hello, World!”;
layout.Children.Add(label);
//Add a button
Button button = new Button();
button.Text = “Click me!”;
button.Clicked += OnButtonClicked;
layout.Children.Add(button);
With Xamarin.Forms, you can create a simple UI layout using just a few lines of code. You can then customize the look and feel of your UI using a wide range of controls and layout options.
Implementing Functionality with .NET
One of the main advantages of using .NET for Android app development is the extensive range of libraries and frameworks available to implement functionality. These tools enable developers to build powerful and efficient features for their Android apps.
The .NET libraries for Android app development are vast, providing solutions for a range of development needs. Some of the most commonly used libraries include:
Library | Functionality |
---|---|
Xamarin.Forms | A cross-platform UI toolkit that allows for building native UIs for Android, iOS, and Windows using a single, shared codebase. |
Xamarin.Android | A library that provides access to the native Android APIs, allowing for the development of native Android apps using C# and .NET. |
Android Support Libraries | A collection of libraries that provide backwards compatibility for new Android features, making it easier to develop across multiple versions of Android. |
Xamarin.Essentials | A library that provides easy access to a range of device-specific and platform-specific features, such as sensors, connectivity, and storage. |
Here is an example of how to implement functionality for requesting data using a REST API with .NET:
// Create a new HttpClient and set the base address
var client = new HttpClient()
client.BaseAddress = new Uri(“https://myapi.com/”);// Send a GET request for data and receive a response
HttpResponseMessage response = await client.GetAsync(“data”);
if (response.IsSuccessStatusCode)
{
var data = await response.Content.ReadAsStringAsync();
// Process the data
}
With .NET, developers have a wide range of tools at their disposal to implement complex functionality for their Android apps with ease. By leveraging these libraries and frameworks, developers can create powerful and efficient features for their apps, maximizing their potential and user experience.
Testing and Debugging Your Android App
Testing and debugging are crucial steps in the Android app development process. Fortunately, .NET provides you with a variety of tools and techniques to help you ensure that your app runs smoothly and reliably.
Testing Your Android App with .NET
Testing your Android app with .NET involves a variety of strategies, ranging from unit testing to user acceptance testing (UAT). By creating unit tests, you ensure that individual components of your app work as they are supposed to, while UAT enables you to test your app under real-world conditions.
.NET provides a variety of testing frameworks that you can use to automate your testing process. One such framework is NUnit, a popular unit testing framework that provides a rich set of features to enable you to write efficient and effective tests for your Android app.
NUnit provides you with a variety of assertions that enable you to verify that your app behaves as it should under a variety of conditions. By using assertions, you can validate the output of your app and ensure that it is performing as expected.
In addition to NUnit, you can also use the Xamarin Test Cloud, a cloud-based testing service that enables you to test your app on a wide variety of devices and platforms. With Xamarin Test Cloud, you can automate your testing process and reduce the amount of time and effort required to test your app thoroughly.
Debugging Your Android App with .NET
Debugging your Android app with .NET involves identifying and resolving issues that prevent your app from running as expected. .NET provides you with a variety of tools and techniques to help you identify and resolve these issues quickly and efficiently.
One of the most useful tools for debugging your Android app with .NET is the Visual Studio debugger. The debugger enables you to step through your code line by line, inspect variables and objects, and identify issues that prevent your app from running as expected.
In addition to the debugger, you can also use logging and tracing to identify and resolve issues in your app. By incorporating logging and tracing into your app, you can track the activity of your app and identify issues that may be difficult to reproduce.
Finally, you can use Xamarin Insights, a real-time crash reporting and analytics service, to identify and resolve issues in your app. With Xamarin Insights, you can track crashes and exceptions in your app, and receive detailed reports that help you diagnose and fix the problem quickly and efficiently.
By using these tools and techniques, you can ensure that your Android app runs smoothly and reliably, and provides your users with a great experience.
Deploying Your Android App
Once you have completed your Android app development, it’s time to deploy it to the market. You need to package your app, sign it, and publish it on the Google Play Store. Follow these steps to deploy your Android app built with .NET:
- Package your app into an APK file. You can do this using the Visual Studio IDE or the Android SDK tools.
- Sign your APK file with a digital certificate. This is required for app distribution on the Google Play Store.
- Create a Google Play Store account and register as a developer. There is a one-time registration fee of $25.
- Upload your signed APK file to the Google Play Console, along with descriptive information about your app, such as title, description, screenshots, and pricing.
- Submit your app for review by the Google Play Store team. This process usually takes a few hours to a few days.
- Once your app is approved, it will be available on the Google Play Store for users to download and install.
Make sure to thoroughly test your app before submitting it for review. Also, keep in mind that the Google Play Store has guidelines and policies that your app must adhere to. Be sure to read and follow these guidelines carefully.
Tip: Consider utilizing beta testing groups on the Google Play Console to test your app with a smaller group of users before releasing it to the public.
Deploying Android apps built with .NET technology to the Google Play Store can be a straightforward process if you follow the steps above. Publishing your app to the market opens up new opportunities for user engagement and revenue generation.
Cross-Platform Development with .NET for Android
.NET offers a powerful set of tools and frameworks for developing cross-platform mobile applications. With its highly popular Xamarin framework, developers can efficiently build Android apps using C# and .NET.
Xamarin allows developers to create native Android apps using .NET and C# code, while also supporting cross-platform development for iOS and Windows platforms. This means that developers can create a single codebase for the three platforms, improving their development speed and reducing costs.
Xamarin comes with a rich set of .NET libraries and API integrations, making it easy to create feature-rich and high-performance Android apps. Developers can use the Xamarin.Forms framework for creating UI elements that are shared across the three platforms, while also customizing platform-specific UIs as needed.
Here’s an example of using Xamarin to create a simple Android app:
// C# code example
using Xamarin.Forms;
namespace HelloWorld
{
public class App : Application
{
public App()
{
MainPage = new ContentPage
{
Content = new Label
{
Text = “Hello, World!”,
VerticalOptions = LayoutOptions.CenterAndExpand,
HorizontalOptions = LayoutOptions.CenterAndExpand,
}
};
}
}
}
In this example, Xamarin.Forms is used to create a label containing the text “Hello, World!” and align it to the center of the screen.
With its cross-platform capabilities and rich feature set, Xamarin makes it easy and efficient to develop high-quality Android apps using .NET.
Conclusion
Congratulations! You have now reached the end of our comprehensive guide on creating Android apps with .NET technology. We hope you found the information informative and helpful in your Android app development journey.
Throughout this guide, we covered all aspects of developing an Android app with .NET, including setting up the development environment, choosing the right framework, designing the user interface, implementing functionality, testing and debugging, and deploying the app.
We also explored cross-platform development options with Xamarin, providing code examples and discussing the benefits and limitations of this approach.
Remember, as technology continues to evolve, so does the world of app development. Be sure to keep up with research and industry trends to stay on top of the game.
Thank you for choosing to learn with us, and we wish you the best of luck in your future endeavors with Android app development!
FAQ
Q: How can I create Android apps using .NET?
A: To create Android apps using .NET, you need to follow a few steps. First, set up your development environment by downloading and installing the necessary tools and SDKs. Next, choose the right framework for your app development needs. Once you have your environment set up and framework selected, you can start designing the user interface and implementing functionality using .NET libraries. Finally, test and debug your app before deploying it to the market. Cross-platform development options are also available with .NET for Android app development.
Q: What are the benefits of using .NET for Android app development?
A: Using .NET for Android app development offers several benefits. Firstly, .NET provides a robust and mature framework with a wide range of tools and libraries. This allows for faster development and code reuse across platforms. Additionally, .NET offers excellent performance and scalability, making it ideal for building complex and high-performance apps. Finally, with the cross-platform capabilities of .NET, you have the option to develop apps that run on multiple platforms, including Android.
Q: How do I set up the development environment for Android app development with .NET?
A: To set up the development environment for Android app development with .NET, you will need to download and install the necessary tools and SDKs. Start by installing the latest version of Visual Studio, which includes the Android development workload. Next, install the Android SDK and configure it in Visual Studio. You may also need to set up an Android emulator or connect a physical Android device for testing. Detailed step-by-step instructions can be found in the documentation provided by Microsoft.
Q: Which framework should I choose for Android app development with .NET?
A: There are multiple frameworks available for Android app development with .NET, each with its own strengths and features. Some popular options include Xamarin, Xamarin.Forms, and NativeScript. The choice of framework depends on your specific requirements and preferences. Xamarin is a powerful and widely-used framework for cross-platform app development, while Xamarin.Forms provides a UI abstraction layer for creating shared UI code. NativeScript, on the other hand, allows you to build native Android apps using JavaScript and TypeScript. Consider your development needs and explore the features of each framework before making a decision.
Q: What is the Model-View-ViewModel (MVVM) pattern in Android app development with .NET?
A: The Model-View-ViewModel (MVVM) pattern is a popular architectural pattern used in Android app development with .NET. It separates the logic of the app into three components: the model, the view, and the view model. The model represents the data and business logic, the view represents the user interface, and the view model acts as a mediator between the model and the view. MVVM provides a clear separation of concerns, making the app easier to develop, test, and maintain. It also supports data binding, allowing for a more responsive and interactive user interface.
Q: What are the key aspects of designing a user-friendly UI for Android apps with .NET?
A: Designing a user-friendly UI for Android apps with .NET involves considering several aspects. Firstly, focus on creating a visually appealing UI that aligns with the overall branding and theme of your app. Keep the UI clean and clutter-free, ensuring that important elements are easily accessible. Consider the user experience and make sure the app is intuitive and easy to navigate. Pay attention to factors such as touch target sizes, font sizes, and color contrast for improved accessibility. Finally, leverage the .NET UI frameworks available for Android app development, such as Xamarin.Forms, to streamline the UI development process.
Q: What are some of the .NET libraries and frameworks that can be used to implement functionality in Android apps?
A: There are several .NET libraries and frameworks that can be used to implement functionality in Android apps. Some popular options include Xamarin.Android, Xamarin.Essentials, and ReactiveUI. Xamarin.Android provides access to the full Android API, allowing you to leverage the native capabilities of the platform. Xamarin.Essentials offers a set of cross-platform APIs for common functionality such as device sensors, connectivity, and file system access. ReactiveUI, on the other hand, is a powerful framework for building reactive and event-driven UIs. These libraries and frameworks provide a wide range of tools for adding functionality to your Android app using .NET.
Q: How can I test and debug my Android app built with .NET?
A: Testing and debugging are crucial steps in the Android app development process. To test your Android app built with .NET, you can use various testing strategies and tools. Xamarin Test Cloud, for example, allows you to run automated tests on a large number of devices to ensure compatibility and functionality. For debugging, you can use the debugging tools provided by Visual Studio, such as the debugger and the Android Device Monitor. These tools help you identify and resolve potential issues in your app, ensuring a smooth user experience.
Q: How do I deploy my Android app built with .NET?
A: To deploy your Android app built with .NET, you need to follow a few steps. First, you need to package your app by creating an APK file, which contains all the necessary files for installation. Next, you need to sign the APK file using a digital certificate to ensure its authenticity and integrity. Finally, you can publish your app on the Google Play Store by creating a developer account and following the submission guidelines. The Google Play Developer Console provides step-by-step instructions for app deployment to ensure a successful publishing process.
Q: What are the cross-platform development options with .NET for Android?
A: .NET offers cross-platform development options for Android app development, allowing you to build apps that run on multiple platforms. Xamarin is a popular toolset for cross-platform app development with .NET. It allows you to write your app’s code in C# and share it across multiple platforms, including Android, iOS, and Windows. Xamarin.Forms provides an additional level of abstraction, allowing you to create shared UI code for different platforms. This cross-platform capability saves development time and effort, enabling you to reach a wider audience with your app.
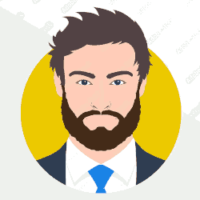
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.