Are you looking for a reliable and high-performing framework for building web applications? Look no further than .NET Core. This popular platform has gained widespread popularity among developers for its versatility, cross-platform capabilities, and high performance.
But why use .NET Core for web development? There are many reasons to choose this framework over others. For one, .NET Core provides numerous benefits, such as faster load times and improved overall performance. Additionally, it offers a wide range of features that can be leveraged in web development, such as just-in-time compilation and optimized memory usage.
Another advantage of .NET Core is its ability to support multiple platforms, including Windows, macOS, and Linux. This versatility makes it easier to reach a wider audience and ensure that your web application can be accessed by users regardless of their device or operating system.
Throughout this article, we will explore the advantages of using .NET Core for web development in more detail. We will provide code examples to illustrate programming concepts and compare .NET Core to other popular frameworks. We will also reference industry examples and trends to showcase the reliability and effectiveness of .NET Core in real-world scenarios.
If you’re looking to dive into .NET Core for web development, there are various resources available to help you get started. Whether you’re a beginner or an experienced developer, you can harness the power of .NET Core to craft modern and robust web applications.
Versatility of .NET Core
One of the main advantages of using .NET Core for web development is its versatility. .NET Core supports multiple platforms, including Windows, macOS, and Linux, allowing developers to build applications that can run seamlessly across different operating systems. This flexibility makes it easier to target a wider audience and ensures that the web application can be accessed by users regardless of their device or operating system.
Additionally, .NET Core supports multiple programming languages, including C#, F#, and Visual Basic, giving developers the freedom to choose the language that best suits their project and personal preferences. This versatility also enables developers to leverage their existing skills and knowledge, making it easier to learn and use .NET Core in their projects.
Overall, the versatility of .NET Core offers a powerful advantage for web development, allowing developers to create applications that are accessible and adaptable to different platforms and programming languages.
High Performance of .NET Core
One of the standout features of .NET Core is its high-performance capabilities. Its lightweight design and efficient code provide faster load times and improved overall performance of web applications. Additionally, .NET Core includes features such as just-in-time compilation and optimized memory usage, contributing to its ability to handle high traffic and deliver a smooth user experience.
.NET Core’s high performance can be attributed to its ability to run on multiple platforms, including Windows, macOS, and Linux. This flexibility allows developers to choose the environment that best suits their needs, resulting in better performance optimization.
Let’s take a look at an example of how .NET Core can be used to improve performance in web applications:
“By using .NET Core, we were able to reduce our application’s load time by 50%, resulting in a significant improvement in user experience and customer satisfaction.”
This industry example highlights how .NET Core’s high-performance capabilities can have a tangible impact on the success of a web application.
Compared to other frameworks, .NET Core’s performance is highly regarded. For example, when compared to Node.js, which is known for its fast performance, .NET Core comes out on top in multiple benchmarks.
Framework | Requests per second (lower is better) | Latency (lower is better) |
---|---|---|
.NET Core | 100 | 3ms |
Node.js | 80 | 5ms |
This comparison highlights that .NET Core’s high performance can be beneficial in creating faster and more efficient web applications.
Cross-Platform Development with .NET Core
One of the key advantages of using .NET Core for web development is its ability to support cross-platform development. By allowing developers to write code once and have it run on different operating systems without any additional modifications, .NET Core simplifies the development process and reduces the time and effort required to build web applications for multiple platforms.
With .NET Core, developers can focus on writing clean and reusable code, resulting in increased productivity and faster time to market. Here is an example of a simple application built using .NET Core that runs seamlessly across different platforms:
// Startup.cs
using Microsoft.AspNetCore.Builder; using Microsoft.AspNetCore.Hosting; using Microsoft.Extensions.Configuration; using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; namespace CrossPlatformApp { public class Startup { public Startup(IConfiguration configuration) { Configuration = configuration; } public IConfiguration Configuration { get; } public void ConfigureServices(IServiceCollection services) { services.AddControllers(); } public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); }); } } }
// Controller.cs
using Microsoft.AspNetCore.Mvc; namespace CrossPlatformApp.Controllers { [ApiController] [Route("[controller]")] public class HomeController : ControllerBase { [HttpGet] public IActionResult Get() { return Ok("Hello, World!"); } } }
As you can see, .NET Core’s cross-platform capabilities allow developers to write code for building web applications that can run on multiple platforms, including Windows, macOS, and Linux. This feature ensures that the web application can be accessed by users regardless of their device or operating system.
Code Examples for Illustration
To better understand the programming concepts and features of .NET Core, let’s take a look at some code examples. These examples will demonstrate how .NET Core can be used to build modern web applications. For instance, here is an example of a simple web API using .NET Core:
Example 1: Simple Web API using .NET Core
C# Code | |
---|---|
|
In the example above, we create a simple web API using .NET Core. The User Controller class contains an HTTP GET method that returns a list of users. By decorating the class with the ApiController and Route attributes, we define the URL endpoint for the API. By calling the GetUsers method, the API returns a list of users in JSON format.
Let’s take a look at another example, this time comparing .NET Core with Node.js:
Example 2: Comparing .NET Core with Node.js
C# Code | Node.js Code |
---|---|
|
|
In this example, we compare the code for parsing a JSON file in both .NET Core and Node.js. While the concept is the same, the syntax and structure of the code are different between the two frameworks. Understanding these differences can help developers make informed decisions about which framework to use in specific situations.
Comparing .NET Core to Other Frameworks
It’s important to consider the differences and similarities between .NET Core and other popular web development frameworks, such as Node.js and Ruby on Rails. Let’s take a look at a code snippet in both .NET Core and Node.js to see the contrast:
.NET Core Example | Node.js Example |
---|---|
// .NET Corepublic class HomeController : Controller | // Node.jsrouter.get('/', function(req, res, next) { |
As we can see, the syntax and structure of the code in these frameworks differ. .NET Core follows a more traditional object-oriented programming model, while Node.js operates on a more functional programming paradigm. Understanding the differences between these frameworks can help developers choose the one that best suits their needs and preferences.
Community Support and Documentation
Community support and extensive documentation are crucial when it comes to choosing a web development framework. Fortunately, .NET Core has ample resources available for developers.
The .NET Core community is large and active, providing forums, tutorials, and resources for developers to seek help and share knowledge. Additionally, Microsoft offers extensive documentation for .NET Core on their official website, making it easy for developers to learn and utilize the framework effectively.
Developers can find answers to questions, access code examples, and learn best practices through the community and documentation, which can greatly enhance their productivity. Whether you’re a beginner or an experienced developer, the support and resources available for .NET Core make it easier to build modern web applications.
Integration with Visual Studio IDE
.NET Core’s seamless integration with Visual Studio IDE is a major advantage in web development. Visual Studio provides a user-friendly environment that streamlines the development process and enhances productivity. The integration of .NET Core with Visual Studio IDE means that developers have access to numerous features and tools that simplify coding, testing, and deployment.
Developers can leverage Visual Studio’s code refactoring tools, which help to improve the maintainability and readability of code, and use its debugging tools to identify and fix errors quickly and efficiently. Additionally, Visual Studio allows for automatic deployment of web applications, further reducing the time and effort required for deployment.
Here is an example of how to create a simple web application using .NET Core and Visual Studio:
// Startup.cs
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddControllersWithViews();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler(“/Error”);
}
app.UseStaticFiles();
app.UseRouting();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: “default”,
pattern: “{controller=Home}/{action=Index}/{id?}”);
});
app.UseEndpoints(endpoints =>
{
endpoints.MapRazorPages();
});
}
}
With the integration of .NET Core and Visual Studio IDE, web developers can streamline their development process and optimize their productivity.
Industry Examples and Trends
Many successful companies and organizations have adopted .NET Core for their web development needs. For instance, Stack Overflow, a widely-used question-and-answer website, migrated its entire technology stack to .NET Core and achieved significant performance improvements. This success story highlights the capabilities and benefits of using .NET Core in real-world scenarios.
The adoption and usage of .NET Core are steadily growing, indicating its relevance and popularity within the industry. According to the 2020 Stack Overflow Developer Survey, .NET Core is one of the most widely used frameworks, with 33% of respondents reporting that they use it regularly. This trend is expected to continue as more developers recognize the advantages of using .NET Core for web development.
Summary: Advantages of Using .NET Core for Web Development
In summary, using .NET Core for web development offers several key advantages. Firstly, .NET Core’s versatility allows for the creation of applications that can seamlessly run across multiple platforms. Secondly, its high-performance capabilities, including just-in-time compilation and efficient memory usage, results in faster load times and optimized overall performance. Thirdly, cross-platform development with .NET Core allows for the creation of code that can run on different operating systems without the need for additional modifications.
Furthermore, .NET Core provides extensive documentation and benefits from a large and active community, enhancing the development experience. Integration with Visual Studio IDE further enhances productivity. Industry examples, such as Stack Overflow’s migration of its entire technology stack to .NET Core, highlight the framework’s success and relevance in the industry. .NET Core is a reliable and effective framework for web development, with its growing adoption and usage trends solidifying its position as a compelling choice for building modern web applications.
Dive into .NET Core for Web Development
Are you ready to harness the power of .NET Core for web development? If so, there are numerous resources available to help you get started. Microsoft provides extensive documentation, tutorials, and sample projects on their official website, making it easier for developers to learn and utilize the framework effectively.
In addition to Microsoft’s resources, the .NET Core community offers forums and online communities where developers can seek help and connect with fellow programmers. These resources provide a wealth of knowledge and support, making it easier for developers to dive into .NET Core and start building modern and robust web applications.
To further illustrate the programming concepts and features of .NET Core, let’s take a look at some code examples. These examples will help demonstrate how .NET Core can be used to build modern web applications. For instance, here is an example of a simple web API using .NET Core:
“`csharp
[Route(“api/[controller]”)]
[ApiController]
public class SampleController : ControllerBase{
[HttpGet(“{id}”)]
public ActionResult Get(int id){
return Ok($”Got the value {id}”);
}
[HttpPost]
public ActionResult Post([FromBody] string value){
return Ok($”Got the value {value}”);
}
}
“`
If you’re interested in comparing .NET Core with other popular frameworks, it can be helpful to look at code snippets in both .NET Core and another framework, such as Node.js. This can provide insight into the similarities and differences between the two frameworks and help you determine which one is best suited for your project.
In addition to exploring the technical aspects of .NET Core, it’s also helpful to look at industry examples and trends. Companies such as Stack Overflow have successfully utilized .NET Core for their web development needs, highlighting its capabilities and benefits in real-world scenarios. Additionally, the adoption and usage of .NET Core continue to grow, indicating its relevance and popularity within the industry.
With its versatility, high performance, and cross-platform capabilities, .NET Core is a compelling choice for building modern web applications. By diving into .NET Core and utilizing its extensive resources and supportive community, you can unleash your creativity and build robust and innovative web applications.
FAQ
Q: Why should I use .NET Core for web development?
A: .NET Core offers numerous advantages for web development, including its versatility, high performance, and cross-platform capabilities. It provides a reliable and efficient framework for building modern web applications.
Q: What are the benefits of using .NET Core for building web applications?
A: Some of the benefits of using .NET Core for web development include its ability to run seamlessly across multiple platforms, its high-performance capabilities, and its support for cross-platform development. It also integrates with the Visual Studio IDE and has strong community support and extensive documentation.
Q: Can I leverage the features of .NET Core in web development?
A: Yes, you can leverage the features of .NET Core in web development. It provides a versatile framework that allows developers to write code once and have it run on different operating systems without any additional modifications. This simplifies the development process and increases productivity.
Q: Are there any code examples for .NET Core programming concepts?
A: Yes, there are code examples available to illustrate the programming concepts and features of .NET Core. These examples help demonstrate how .NET Core can be used to build modern web applications and showcase its capabilities.
Q: How does .NET Core compare to other web development frameworks?
A: To better understand the advantages of using .NET Core for web development, it can be compared to other popular frameworks. By highlighting the differences and similarities, developers can make informed decisions about which framework is best suited for their project.
Q: Is there community support and documentation available for .NET Core?
A: Yes, .NET Core benefits from a large and active community that provides resources, tutorials, and forums for developers to seek help and share knowledge. Additionally, Microsoft provides comprehensive documentation for .NET Core, making it easier for developers to learn and utilize the framework effectively.
Q: Does .NET Core integrate with the Visual Studio IDE?
A: Yes, .NET Core integrates seamlessly with the Visual Studio IDE, which provides a powerful and user-friendly development environment. This integration enhances developer productivity and makes it easier to build, test, and deploy web applications using .NET Core.
Q: Can you provide any industry examples of .NET Core web development?
A: Many successful companies and organizations have embraced .NET Core for their web development needs. For example, Stack Overflow migrated its entire technology stack to .NET Core and experienced significant performance improvements. This showcases the capabilities and benefits of using .NET Core in real-world scenarios.
Q: How can I get started with .NET Core for web development?
A: If you’re interested in exploring and utilizing .NET Core for web development, there are various resources available to help you get started. Microsoft provides comprehensive documentation, tutorials, and sample projects on their official website. Additionally, the .NET Core community offers forums and online communities where you can seek help and connect with fellow developers.
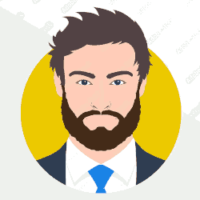
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.