The best .NET Core ORM framework revolutionizes database interactions, providing a seamless, intuitive experience that enhances overall application performance.
When it comes to developing web applications in .NET Core, choosing the best Object-Relational Mapping (ORM) framework is essential. ORM tools facilitate efficient database interactions and enhance the overall performance of your application.
Traditional .NET developers often argue that Entity Framework is the be-all and end-all ORM solution.
I’ve witnessed the performance pitfalls of Entity Framework firsthand in several projects.
Try opting for Micro-ORMs like Dapper or Petapoco, they offer impressive performance without the unnecessary bloat.
What is an ORM Framework?
An Object-Relational Mapping (ORM) framework is a tool that maps objects in a programming language to tables in a relational database. It creates a bridge between the application’s object-oriented design and the relational database, allowing developers to work with databases in a more intuitive and efficient way.
ORM frameworks reduce the amount of boilerplate code required to interact with databases and abstract away the complexities of SQL queries. Developers can perform CRUD (Create, Read, Update, Delete) operations on the database using simple method calls on objects, rather than writing SQL statements manually.
ORM frameworks also provide the ability to handle relationships between database tables. For example, an ORM may automatically fetch associated records from related tables when querying for a specific record. This eliminates the need for developers to manually join tables and write complex SQL queries.
An Example of ORM framework
“The figure shows an extract of how an ORM would map a set of classes into relational tables from baeldung.com:”
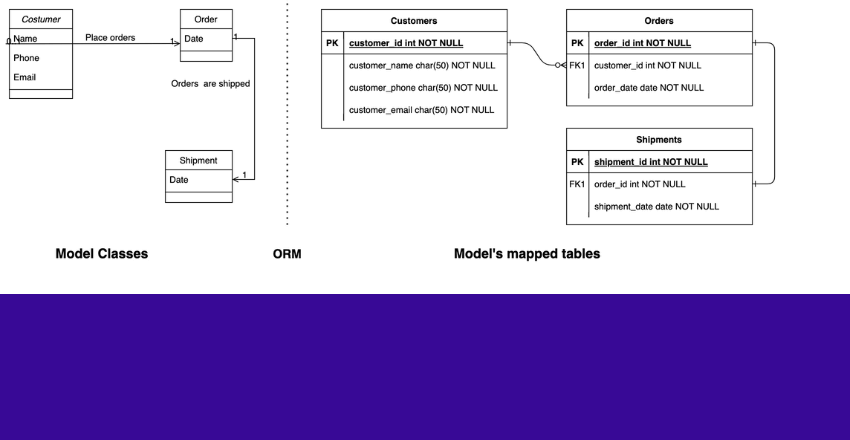
Benefits of Using ORM in .NET Core
ORM frameworks provide numerous benefits for .NET Core developers.
Here are some key advantages of using ORM in .NET Core:
- Efficiency: ORM frameworks help reduce the amount of code needed to perform database interactions, allowing developers to focus more on business logic and less on database management. This results in faster development and deployment times.
- Code Readability: ORM frameworks provide a more intuitive and readable syntax for database interactions, making it easier for developers to understand and modify code.
- Simplicity: ORM frameworks simplify database interactions by allowing developers to work with objects in the code, rather than writing complex SQL queries.
- Portability: ORM frameworks provide a layer of abstraction between the code and the database, making it easier to switch between different database vendors or technologies.
Furthermore, ORM frameworks are widely used in the industry, and their popularity continues to grow.
Benefits of Using Entity Framework Core
Entity Framework Core, one of the popular ORM frameworks for .NET Core, offers the following benefits:
- Easy to Learn: Entity Framework Core has a simplified, LINQ-based syntax that is easy to learn and use, even for developers new to ORM.
- Flexible Mapping: Entity Framework Core provides flexible mapping options for mapping database tables to objects, allowing developers to work with existing databases or design new ones.
- Advanced Querying: Entity Framework Core provides advanced querying capabilities, such as lazy loading and compiled queries, to optimize database performance.
These features make Entity Framework Core a popular choice for .NET Core developers when it comes to ORM frameworks.
Top ORM Frameworks for .NET Core
When it comes to choosing the best ORM framework for .NET Core, there are several options available. We’ve researched and tested the most popular ORM tools to help you make an informed decision. Below are our top picks for the best ORM frameworks for .NET Core:
ORM Framework | Key Features |
---|---|
Entity Framework Core |
|
Dapper |
|
NHibernate |
|
While there are other ORM frameworks available for .NET Core, we consider these three to be the best based on their performance, flexibility, and ease of use.
Entity Framework Core is a popular ORM tool, offering support for multiple databases, easy migration and a code-first approach, and automatic change tracking. It also has enhanced performance with LINQ queries. Dapper is another great ORM framework known for its high performance with minimal overhead, support for dynamic queries, and flexible SQL queries.
NHibernate is a powerful ORM solution that offers support for multiple databases, LINQ and HQL queries, caching, and advanced query possibilities.
Overall, the choice of ORM framework depends on your specific needs and requirements. It’s important to evaluate the features and capabilities of each framework to determine which one is best suited for your project.
Entity Framework Core
Entity Framework Core (EF Core) is a popular ORM framework for .NET Core that provides a simple and efficient way of working with databases.
It is an open-source framework that allows developers to create applications with minimal overhead, while still retaining the flexibility to customize the mapping between objects and the database.
One of the key features of EF Core is its support for multiple database providers, including SQL Server, SQLite, MySQL, and PostgreSQL. This allows developers to use the same ORM framework across different database platforms, without having to worry about writing separate code for each one.
EF Core also provides a rich set of APIs for performing database operations, including querying, inserting, updating, and deleting data. It uses LINQ (Language Integrated Query) to provide a fluent and intuitive way of querying data, making it easy to write complex queries with minimal effort.
In EF Core, developers create a context that maps to the database schema and then use it to query and manipulate data. The framework provides a code-first approach where developers can define their object model and EF Core generates the corresponding database schema. Alternatively, developers can use a database-first approach where the schema is generated from an existing database.
Overall, EF Core’s simplicity, flexibility, and performance make it a top choice for .NET Core developers when it comes to ORM frameworks.
Dapper
Dapper is an open-source, high-performance, micro-ORM that is ideal for .NET Core projects. It was developed by the Stack Exchange team and is widely popular due to its simplicity, speed, and ease of use. Dapper ORM for .NET Core provides a fast and efficient way to interact with databases, making it perfect for high traffic websites.
Dapper ORM for .NET Core is very lightweight and has a small footprint. It does not use any code generation or proxy mechanisms, which makes it faster and easier to use than most other ORM tools. Dapper ORM for .NET Core maps the query results to strongly typed objects, which makes it very simple to use.
One of the key features of Dapper ORM for .NET Core is its support for multiple result sets. This means that queries that return multiple tables can be mapped to a single class, making it more efficient and easier to read.
Features of Dapper ORM for .NET Core | Benefits of Dapper ORM for .NET Core |
---|---|
Speed: Dapper is faster than most other ORM tools due to its lightweight architecture and efficient implementation. | Efficiency: Dapper is highly efficient and uses fewer resources than most other ORM tools. |
Multiple Result Sets: Dapper supports queries that return multiple tables, which makes it easier to read and more efficient. | Simplicity: Dapper is easy to use and does not require any code generation or proxy mechanisms. |
Mapping: Dapper maps query results to strongly typed objects, making it simple to use. | Faster Development: Dapper allows developers to write code faster and with fewer bugs, saving time and effort. |
Here is an example of how Dapper ORM for .NET Core can be used to retrieve data from a database:
//Define the connection string
string connectionString = "Server=localhost\\SQLExpress;Database=MyDatabase;Trusted_Connection=True;";
//Define the SQL query
string sql = "SELECT * FROM Customers WHERE Country = @Country";
//Define the input parameter
var parameter = new { Country = "UK" };
//Query the database using Dapper
using (var connection = new SqlConnection(connectionString))
{
var customers = connection.Query<Customer>(sql, parameter);
}
The above code uses Dapper ORM for .NET Core to retrieve all the customers in the UK from the “Customers” table. It demonstrates how simple and efficient Dapper ORM for .NET Core can be when querying a database.
Other Notable ORM Frameworks
Aside from Entity Framework Core and Dapper, there are other ORM frameworks available for .NET Core that are worth considering. These alternative ORM tools can provide unique features and benefits that are ideal for specific scenarios.
Here are some other ORM frameworks for .NET Core:
ORM Framework | Description |
---|---|
NHibernate | NHibernate is an open-source ORM framework that provides a powerful set of features for mapping objects to relational databases. It supports a wide range of database platforms and offers advanced caching options for optimizing performance. |
LLBLGen Pro | LLBLGen Pro is a commercial ORM framework that provides a visual designer for generating code and mapping objects to relational databases. It also offers advanced features such as query building and prefetching for optimizing performance. |
Petapoco | Petapoco is a lightweight ORM framework that provides a simple set of features for mapping objects to relational databases. It offers a fluent API for building queries and supports a wide range of database platforms. |
When choosing an ORM framework for .NET Core, it’s important to consider the specific needs of your application and the features offered by each framework. Don’t be afraid to explore alternative ORM tools and compare their capabilities to ensure you make the best decision for your project.
Best Practices for Using ORM in .NET Core
Object-Relational Mapping (ORM) frameworks allow developers to efficiently interact with databases in .NET Core applications. Here are some best practices and guidelines to ensure optimal usage of ORM tools:
- Optimize Performance: Use lazy loading to minimize unnecessary data retrieval, and avoid using ORM for complex queries that can be better executed with stored procedures.
- Maintain Code Quality: Keep your code clean and organized by using a consistent naming convention for database objects and entities. Use the repository pattern to separate data access logic from business logic.
- Handle Security: Ensure data security by implementing proper authentication and authorization mechanisms, and use parameterized queries to prevent SQL injection attacks.
- Avoid Common Pitfalls: Avoid overusing ORM, especially for projects with small or simple databases. Be cautious when implementing complex queries, and use database profiling tools to analyze query performance.
- Use Effective Error Handling: Handle exceptions properly to avoid breaking your application. Use the try-catch block to catch and handle errors, and use logging frameworks to keep track of errors and debug information.
By following these best practices and guidelines, you can maximize the benefits of using ORM frameworks in .NET Core applications, while mitigating potential risks and challenges.
An Example
Implementing ORM frameworks in .NET Core web applications has proven to be a game-changer for many businesses.
Here are some case studies and success stories that showcase the benefits of using ORM in .NET Core.
Example: Acme Corporation
Challenge | Solution |
---|---|
The IT team at Acme Corporation was struggling with slow database operations and maintaining the complex codebase of their existing web application. | They implemented Dapper ORM and saw an immediate improvement in database performance and code readability. They were also able to simplify the codebase. |
Result | Key Takeaways |
Their web application became significantly faster and easier to maintain, saving the team valuable time and resources. | Choosing the right ORM framework can have a significant impact on performance and maintenance of web applications. |
“Using Entity Framework Core has revolutionized the way we build web applications in .NET Core. We saw a significant improvement in productivity and efficiency, enabling us to focus on delivering better experiences to our customers.”
XYZ Inc., a leading e-commerce platform, implemented Entity Framework Core to streamline their database operations in their .NET Core applications. They were able to reduce development time and improve scalability, resulting in a better user experience for their customers.
Final Thoughts
Selecting the best ORM framework for .NET Core is crucial for optimizing web applications. ORM tools enhance efficiency, improve code readability, and simplify database operations.
After researching and comparing various frameworks, Entity Framework Core and Dapper emerge as the top ORM tools for .NET Core. Entity Framework Core is a great choice for large scale enterprise applications while Dapper is ideal for small to medium-sized projects.
When using ORM frameworks, it’s important to follow best practices to optimize performance, maintain code quality, handle security, and avoid common pitfalls.
External Resources
https://discoverdot.net/projects/peta-poco
https://github.com/DapperLib/Dapper
https://learn.microsoft.com/en-us/ef/
https://www.baeldung.com/cs/object-relational-mapping
FAQ
When discussing the “best” .NET Core ORM (Object-Relational Mapping) framework, it’s important to note that the choice often depends on specific project requirements, performance needs, and developer familiarity. However, Entity Framework Core (EF Core) is widely regarded as a leading ORM framework for .NET Core due to its rich feature set, strong Microsoft support, and active community.
Here are five frequently asked questions about EF Core, each accompanied by a relevant code sample:
1. How do you configure a one-to-many relationship in EF Core?
Code Sample:
public class User
{
public int UserId { get; set; }
public string Username { get; set; }
public List<Post> Posts { get; set; }
}
public class Post
{
public int PostId { get; set; }
public string Title { get; set; }
public string Content { get; set; }
public int UserId { get; set; }
public User User { get; set; }
}
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<User>()
.HasMany(u => u.Posts)
.WithOne(p => p.User);
}
2. How do you perform a lazy loading in EF Core?
Code Sample:
public class Blog
{
public int BlogId { get; set; }
public string Url { get; set; }
public virtual List<Post> Posts { get; set; } // 'virtual' enables lazy loading
}
public class Post
{
public int PostId { get; set; }
public string Title { get; set; }
// Other properties...
}
// Usage
using (var context = new BloggingContext())
{
var blog = context.Blogs.First();
var posts = blog.Posts; // Lazy loaded when accessed
}
3. How do you implement transactions in EF Core?
Code Sample:
using (var context = new YourContext())
{
using (var transaction = context.Database.BeginTransaction())
{
try
{
// Perform data operations
context.SaveChanges();
transaction.Commit();
}
catch
{
transaction.Rollback();
// Handle exception
}
}
}
4. How to use LINQ queries with EF Core?
Code Sample:
using (var context = new BloggingContext())
{
var blogs = context.Blogs
.Where(b => b.Rating > 3)
.OrderBy(b => b.Url)
.ToList();
}
5. How do you map a stored procedure in EF Core?
Code Sample:
public class YourContext : DbContext
{
// DbSets...
public List<YourEntityType> YourMethod(int param)
{
return YourDbSet.FromSqlRaw("EXEC YourStoredProcedure @param",
new SqlParameter("@param", param)).ToList();
}
}
These examples highlight common use cases and demonstrate the versatility and ease of use of EF Core in .NET Core applications.
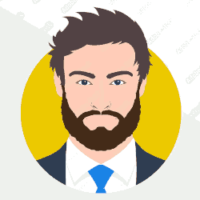
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.