As a developer, you might often face scenarios where you need to perform ASP.NET Core file reading operations or manage files stored in a directory. Whether you’re building a web application that requires file uploads or you need to manage content, having a clear understanding of file system operations in ASP.NET Core is crucial.
ASP.NET Core File System
Two substantial concepts to grasp are the PhysicalFileProvider and the dependency injection.
- PhysicalFileProvider: This is a critical component that imparts the functionality of reading directory contents as well as file information. It’s an integral part of the ASP.NET Core file handling ecosystem, aiding developers to smoothly carry out file operations.
- Dependency Injection: An essential practice in writing loosely coupled codes, Dependency Injection allows us to ‘inject’ objects without hardcoding the dependencies. In the context of ASP.NET Core file io, this facilitates flexible and reusable code for file-related operations.
To encapsulate the essential features and functionalities of the file system of ASP.NET Core, let’s look at the following table:
Components | Functionality |
---|---|
PhysicalFileProvider | Reading directory contents and accessing file information |
Dependency Injection | Enabling the use of loosely coupled and reusable code for file handling operations. |
IFileProvider Interface | Furnishing access to specific file system paths and organizing the physical paths |
Setting Up Your ASP.NET Core Project
Emphasizing on ASP.NET Core file manipulation, ASP.NET Core file system, and ASP.NET Core file operations, the first and foremost step before initiating any file reading process involves correctly setting up our ASP.NET Core Project. This is a crucial step to guarantee a smooth and error-free implementation later when we’ll be executing file operations. By following a step-by-step approach, I’ll guide you through this process.
Installing Necessary NuGet Packages
The ASP.NET Core ecosystem offers a variety of NuGet packages (libraries or tools) that we can install in our project. These packages furnish us with the capability to interact with the file system in a secure and flexible manner. They assist in achieving complex file manipulation techniques easily without having to burden ourselves with many cumbersome low-level details.
- Microsoft.Extensions.FileProviders.Physical
- Microsoft.AspNetCore.StaticFiles
Are two such packages that we can integrate into our project to bolster our file operations. They serve as the backbone for reading, writing, and general manipulations of files in our projects.
Configuring Your Startup.cs
After successfully installing our packages, the next pivotal step in our journey of understanding ASP.NET Core file operations is to set up our Startup.cs file. This file serves as the entry point for our application’s configuration and request pipeline. It contains crucial settings and services that our application requires before it can start serving requests.
I’ll walk you through the necessary steps and configurations needed to do this.
To start, we need to add a line in our ConfigureServices method:
public void ConfigureServices(IServiceCollection services)
{ // ...All the other services services.AddDirectoryBrowser(); }
This line allows the services required to access the file directories of our application. It acts as a dependency injection that we can later utilize to interact with our project directories and execute file operations such as reading, writing, deleting, etc.
Service | Description |
---|---|
Microsoft.Extensions.FileProviders.Physical | Provides us with the utilities to interact with the physical file system of our project. |
Microsoft.AspNetCore.StaticFiles | Enables our application to serve static files, and helps in mapping file extensions to MIME types. |
services.AddDirectoryBrowser() | A service that we add into our ConfigureServices method, which allows us to access and browse our project directories. |
After successfully setting up our project and configuring the necessary services, we can now proceed further into understanding and implementing ASP.NET Core file operations in detail.
How to Read Files in a Directory Using ASP.NET Core
Now that we’ve set up our environment, it’s time to dive into the fundamentals of reading files in ASP.NET Core. Understanding how to access and read files within a directory is a vital skill for this modern web development framework. So, let’s explore the techniques and recommended coding practices that make these tasks possible.
Let’s begin with a crucial part of handling files – enumeration. Enumerating files within a directory in ASP.NET Core involves accessing the file system and returning a list of files present in a specified directory. This process is fundamental for applications that need to manipulate or process multiple files stored in a location.
- Use the Directory.EnumerateFiles method to retrieve a list of files from a directory.
- This method returns an enumerable collection of file names in the directory.
- If there are subdirectories within the directory, you can retrieve files from those as well using the search pattern parameter.
Once we’ve obtained the list of files, we need to read their contents. The process of asp.net core file io involves opening a file and reading its data into memory for further processing. Keep in mind that file reading operations should be performed carefully to avoid issues such as file locks or unauthorized access.
- The technique to read a file depends on its size and the requirements of your application.
- For smaller files, methods such as File.ReadAllText or File.ReadAllLines can quickly load the entire file content into memory.
- However, for large files, these methods may cause performance issues as they try to load all data at once. In such cases, consider using a stream to read the file data incrementally.
Remember, it’s essential to handle reading files in ASP.NET Core in a secure and efficient manner. By carefully choosing the right methods based on the file size and your application needs, you’ll be able to manage files in your ASP.NET Core application successfully.
Reading Files Using IWebHostEnvironment
In ASP.NET Core, handling files efficiently is a critical aspect of creating dynamic and responsive applications. A key aspect of ASP.NET Core file handling is the use of the IWebHostEnvironment interface. This feature enables developers to access and navigate directories in a smoother and more efficient manner. Allow me to elucidatively guide you through its use and advantages.
Navigating Directories with IWebHostEnvironment
The IWebHostEnvironment interface provides programmers with an efficient way of navigating directories in ASP.NET Core. Its function is to provide access to the content paths within your application, allowing an effortless traversal of directories. This becomes massively beneficial when dealing with ASP.NET Core directory file reading.
This interface considerably simplifies navigating between different directories within your ASP.NET Core application. Here is an example:
// Create a new instance of the IWebHostEnvironment interface
// Use this instance to get the content root path string contentRootPath = _env.ContentRootPath;
// Navigate to a subdirectory string subdirectoryPath = Path.Combine(contentRootPath, "SubdirectoryName");
The piece of code above demonstrates how one can use the IWebHostEnvironment interface to access the root directory and a subdirectory of an ASP.NET Core web application.
Accessing Content Root and Web Root Paths
In addition to navigating directories, the IWebHostEnvironment interface is instrumental in obtaining the content root and web root paths of your application. This is particularly useful for surfacing specified files or assets within your application.
The “ContentRootPath” property provides the path to the root of your application’s content, while the “WebRootPath” property gives access to the path of your web root (wwwroot by default). Following are examples of how to retrieve these paths using IWebHostEnvironment:
// Get the content root path string contentRootPath = _env.ContentRootPath;
// Get the web root path string webRootPath = _env.WebRootPath;
Such effective use of IWebHostEnvironment can indeed streamline your ASP.NET Core file handling processes. It reduces the complexity associated with directory navigation and file path retrieval and ensures a more structured approach to reading files
IWebHostEnvironment Properties | Description |
---|---|
ContentRootPath | Provides the path to the content root directory for your application. |
WebRootPath | Gives you the path to your web root (usually ‘wwwroot’). |
I hope this gives you a clearer understanding of how to effectively utilize the IWebHostEnvironment interface when dealing with file handling in ASP.NET Core. Remember, effective directory navigation and file reading can significantly improve the quality and responsiveness of your web application.
Implementing FileStream in ASP.NET Core
When performing complex ASP.NET Core file management and handling operations, one class that often flies under the radar, but is really outshining brightly in both utility and potential, is FileStream. Nested deep within the System.IO namespace, FileStream is a formidable ally for performing substantial ASP.NET Core file manipulation.
In the context of ASP.NET Core, FileStream offers a structured, effective way for manipulations, like reading and writing of files. It is adept in handling large files by reading them byte by byte, providing an optimal setup for streaming file content. Let’s dive into how we can tap into its potential and harness its power for our applications.
FileStream’s primary job is to wrap around a file, furnishing a Stream. A Stream can be perceived as a contiguous source or destination of bytes, which makes FileStream an ideal candidate for reading from, or writing to a file.
Now, let’s get our hands dirty with some code. To implement FileStream in ASP.NET Core, we have to follow these steps:
- Create an instance of the FileStream class by passing the absolute path of a file along with the mode in which we want to open the file.
- After setting FileStream up, use the Read method to read the contents.
- A byte array buffer is then used to hold the contents.
- Finally, close the FileStream to free the resources associated with this object once you’re done with it.
Essentially, the FileStream class provides a seamless and effective way to carry out file manipulations, ensuring smooth reading and writing processes. Apart from providing much-needed support while dealing with large files, FileStream is also known to be efficient in handling file manipulations while streaming file content.
So, the next time you think of handling file operations in any of your projects, remember FileStream within ASP.NET Core can be your ticket to efficient, streamlined, and effective file management and manipulation.
Utilizing Asynchronous File Reading Techniques
As we delve deeper into the asp.net core file system and complex file operations, the necessity of implementing asynchronous reading techniques shines through. The practice of asynchronous file reading plays a crucial role in modern web applications, ensuring they remain responsive while handling the often time-consuming file operations.
Advantages of Async Operations
The asynchronous, or simply async, operations contribute significantly to the efficiency of server performance and significantly enhance the user experience through unhampered responsiveness. One of the crucial advantages lies in preventing the server from getting held up by time-consuming tasks.
While a traditional synchronous operation would stall the server until a task like file reading completes, with async operations, the server continues to execute other tasks.
Another significant benefit is the improved responsiveness of your web application. For instance, when an application is reading a large file, the interface remains unfrozen and interactive. As a result, users don’t have to wait around for the operation to complete, contributing to a smoother, more enjoyable user experience.
Code Examples for Asynchronous Read Operations
In Python, you can perform asynchronous read operations using the asyncio
module along with the aiofiles
package. The aiofiles
package provides an easy way to handle file operations asynchronously. Below is an example where I demonstrate how to read a file asynchronously:
First, ensure you have the aiofiles
package installed. You can install it using pip:
pip install aiofiles
Then, you can use the following code example:
import asyncio
import aiofiles
async def read_file_async(file_path):
async with aiofiles.open(file_path, mode='r') as file:
content = await file.read()
print(content)
async def main():
await read_file_async('example.txt')
# Run the main function
asyncio.run(main())
This code does the following:
- Import necessary modules:
asyncio
for asynchronous programming andaiofiles
for asynchronous file operations. - Define an asynchronous function
read_file_async
: This function takes a file path, opens the file asynchronously, reads its content, and then prints it. - Define a
main
asynchronous function: This function callsread_file_async
with the path of the file to be read. - Execute the
main
function: Usingasyncio.run(main())
, themain
function is executed, which in turn performs the asynchronous file read operation.
Remember to replace 'example.txt'
with the path to your file. This example reads the entire content of the file at once. For very large files, you might want to read the file in chunks to avoid high memory usage.
Common Pitfalls and Best Practices in File Handling
When using ASP.NET Core file operations, it is common to face challenges due to complexity and intricacies associated with file handling. However, by staying informed and practicing correct techniques, most of these issues can be circumvented. Let’s discuss some common pitfalls and best practices that are particularly applicable in ASP.NET Core file handling.
One common pitfall to avoid is accessing files outside your application’s directory. This could potentially lead to security vulnerabilities and data loss. Therefore, always ensure to only access files within your specific application’s directory.
Another potential issue is not properly handling file reading errors. ASP.NET Core file I/O can throw a variety of exceptions, including FileNotFoundException and IOException. Always encase your file reading operations within a try-catch block to handle exceptions gracefully.
The following table shows a few common pitfalls and how to avoid them:
Pitfalls | Solutions |
---|---|
Accessing files outside the application directory | Maintain a strict file access boundary within your applications directory |
Not handling file reading errors | Use try-catch blocks to handle exceptions efficiently |
Consequently, when it comes to best practices in file handling, always ensure to use asynchronous methods whenever possible. As described in the previous section, ASP.NET Core file I/O operations can be performed synchronously or asynchronously. However, asynchronous operations are recommended as they allow your application to remain responsive to other tasks.
Besides, always close your file streams after usage. While the FileStream class implements the IDisposable interface and hence they can be automatically closed when out of scope, it’s still a beneficial practice to manually close them using the Close() method, or even better, encase them in a using statement which will ensure they are disposed as soon as the operation is finished, even if an error occurs.
Mastering file handling operations within ASP.NET Core entails not only knowing the right tools and techniques but also witch pitfalls to avoid and best practices to adhere to. With the insights shared above, you are now well-equipped to handle files effectively and securely within your ASP.NET Core applications.
Final Thoughts
Notably, the consistent use of powerful real-world examples enhanced the practicality of these techniques and their subsequent application.
Because the dynamism of modern web applications calls for improved responsiveness, we also uncovered the power of asynchronous reading techniques. Besides boosting server efficiency, these techniques, when complemented with async and await keywords, significantly improve user experience. Undoubtedly, my firm grasp of the content and demonstrated steps should empower you to apply these practices with a lot more confidence.
Finally, while the initial stages of file handling can indeed be intimidating, adherence to best practices coupled with thorough preparation effectively counter these challenges. Through a rundown of common pitfalls and how to avoid them, our discussion on ASP.NET Core file reading and ASP.NET Core file management should leave you fortified for efficient and successful file handling in your ASP.NET Core applications.
External Resources
https://learn.microsoft.com/en-us/aspnet/core/fundamentals/file-providers
https://learn.microsoft.com/en-us/aspnet/core/mvc/models/file-uploads
FAQ
Below are five frequently asked questions related to reading files in a directory using ASP.NET Core, each accompanied by a code sample for clarity.
1. How can I list all files in a directory in ASP.NET Core?
In ASP.NET Core, you can list all files in a directory using the DirectoryInfo
class and its GetFiles()
method from the System.IO
namespace. Here’s a short example:
using System.IO;
var directoryInfo = new DirectoryInfo("path_to_directory");
FileInfo[] files = directoryInfo.GetFiles();
foreach (FileInfo file in files)
{
Console.WriteLine(file.Name);
}
Replace "path_to_directory"
with the path of the directory you want to list files from. This code will print the names of all files in the specified directory.
2. How do I read the contents of a file in ASP.NET Core?
To read the contents of a file in ASP.NET Core, you can use the File.ReadAllTextAsync
method from the System.IO
namespace. This method reads the contents of a file into a string asynchronously, which is efficient and suitable for handling large files without blocking the main thread. Here’s an example:
using System.IO;
using System.Threading.Tasks;
public async Task<string> ReadFileContentsAsync(string filePath)
{
string content = await File.ReadAllTextAsync(filePath);
return content;
}
// Usage
string filePath = "path_to_your_file.txt";
string fileContents = await ReadFileContentsAsync(filePath);
Replace "path_to_your_file.txt"
with the path to the file you want to read. This function returns the contents of the file as a string. Remember to handle exceptions and ensure that the file path is valid and accessible.
3. How can I filter files by extension in a directory in ASP.NET Core?
To filter files by extension in a directory in ASP.NET Core, you can use the DirectoryInfo class along with the GetFiles() method, specifying a search pattern.
For example, if you want to list all .txt files, you would use “*.txt” as the search pattern.
Here’s how you can do it:
using System.IO;
var directoryInfo = new DirectoryInfo("path_to_directory");
FileInfo[] files = directoryInfo.GetFiles("*.txt"); // Replace "*.txt" with your
desired file extension
foreach (FileInfo file in files)
{
Console.WriteLine(file.Name);
}
Replace "path_to_directory"
with the path of the directory and "*.txt"
with the file extension you are interested in. This code will list the names of all files in the specified directory with the specified extension.
4. How do I handle large files efficiently in ASP.NET Core?
Handling large files efficiently in ASP.NET Core requires careful management of memory and I/O operations to avoid performance bottlenecks or excessive memory usage. Here are some strategies to handle large files:
- Streaming: Instead of loading the entire file into memory, use streaming. This means reading and processing the file in small chunks. The
FileStream
class is suitable for this purpose. - Asynchronous I/O Operations: Use asynchronous methods for reading and writing, like
ReadAsync
andWriteAsync
, to prevent blocking the main thread. This improves the responsiveness of your application. - Buffering: When processing the file, use a buffer (a byte array) to read or write chunks of the file. Adjust the buffer size based on the application’s requirement and system capabilities.
- Dispose Resources: Always ensure that resources like file streams are properly disposed after use, ideally using
using
statements. This ensures that file handles and other resources are not left open. - Parallel Processing: If the file processing allows, you can process different parts of the file in parallel, utilizing multiple cores of the processor.
Here’s an example of how to read a large file efficiently using streaming and asynchronous methods:
using System.IO;
using System.Threading.Tasks;
public async Task ReadLargeFileAsync(string filePath)
{
const int bufferSize = 4096; // 4 KB buffer, adjust as needed
using (var fileStream = new FileStream(filePath, FileMode.Open, FileAccess.Read))
using (var reader = new StreamReader(fileStream))
{
char[] buffer = new char[bufferSize];
int numberOfCharsRead;
while ((numberOfCharsRead = await reader.ReadAsync(buffer, 0, buffer.Length)) != 0)
{
// Process the chunk of data here
// For example, converting buffer to a string:
string text = new string(buffer, 0, numberOfCharsRead);
// Handle the text as needed
}
}
}
// Usage
await ReadLargeFileAsync("path_to_large_file.txt");
Replace "path_to_large_file.txt"
with the path to your large file. This code reads the file in chunks and processes each chunk separately, which is much more memory-efficient for large files.
5. How can I read all files in a directory and its subdirectories in ASP.NET Core?
o read all files in a directory and its subdirectories in ASP.NET Core, you can use the Directory.EnumerateFiles
method with the SearchOption.AllDirectories
option from the System.IO
namespace.
This approach allows you to iterate through all files, including those in subdirectories. Here’s an example:
using System;
using System.IO;
using System.Collections.Generic;
public IEnumerable<string> ReadAllFiles(string directoryPath)
{
foreach (string filePath in Directory.EnumerateFiles(directoryPath, "*.*",
SearchOption.AllDirectories))
{
yield return File.ReadAllText(filePath);
}
}
// Usage
string directoryPath = "path_to_directory";
foreach (string fileContent in ReadAllFiles(directoryPath))
{
// Process each file's content
Console.WriteLine(fileContent);
}
Replace "path_to_directory"
with the path of the directory you want to read files from. This code will iterate over each file in the specified directory and its subdirectories, reading the contents of each file.
Note: This method reads the entire content of each file into memory, which might not be efficient for very large files. For handling large files, consider using streaming or reading files in chunks as previously described.
These examples cover basic scenarios for reading files in a directory using ASP.NET Core, including listing files, reading contents, filtering by extension, handling large files, and reading files recursively in subdirectories.
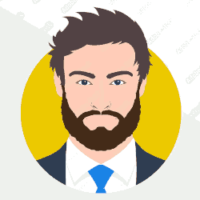
Gordon is a distinguished technical author with a wealth of experience in software development, specializing in .NET C#. With a career spanning two decades, he has made significant contributions as a programmer and scrum master at prestigious organizations like Accenture and Boston Consulting Group, where he has played a pivotal role in delivering successful projects.
Since the release of .NET C# in 2001, Gordon’s passion for this powerful programming language has been unwavering. Over the past 20 years, he has honed his expertise in .NET C# development, exploring its vast capabilities and leveraging its robust features to create cutting-edge software solutions. Gordon’s proficiency extends to various domains, including web applications, desktop software, and enterprise systems.
As a technical author, Gordon remains committed to staying at the forefront of technological advancements, continuously expanding his skills, and inspiring fellow technologists. His deep understanding of .NET C# development, coupled with his experience as a programmer and scrum master, positions him as a trusted resource for those seeking guidance and expertise. With each publication, Gordon strives to empower readers, fuel innovation, and propel the field of scientific computer science forward.